Question
Need C code for the methods listed in the above document for the following: handle_process_arrival_pp handle_process_completion_pp handle_process_arrival_srtp handle_process_completion_srtp handle_process_arrival_rr handle_process_completion_rr Here is full document: -----------------------------------------------------------------------------------------------------------------------
Need C code for the methods listed in the above document for the following: handle_process_arrival_pp
handle_process_completion_pp
handle_process_arrival_srtp
handle_process_completion_srtp
handle_process_arrival_rr
handle_process_completion_rr
Here is full document:
-----------------------------------------------------------------------------------------------------------------------
Source code of oslab.h -
#define QUEUEMAX 10
#define MAPMAX 10
#define TABLEMAX 10
#define POOLMAX 10
#define REFERENCEMAX 20
#define MAX( a, b ) ( ( a > b) ? a : b )
#define MIN( a, b ) ( ( a > b) ? b : a )
struct RCB {
int request_id;
int arrival_timestamp;
int cylinder;
int address;
int process_id;
};
struct PCB {
int process_id;
int arrival_timestamp;
int total_bursttime;
int execution_starttime;
int execution_endtime;
int remaining_bursttime;
int process_priority;
};
struct MEMORY_BLOCK {
int start_address;
int end_address;
int segment_size;
int process_id; //0 indicates a free block
};
struct PTE {
int is_valid;
int frame_number;
int arrival_timestamp;
int last_access_timestamp;
int reference_count;
};
struct RCB handle_request_arrival_fcfs(struct RCB request_queue[QUEUEMAX], int *queue_cnt, struct RCB current_request, struct RCB new_request, int timestamp);
struct RCB handle_request_completion_fcfs(struct RCB request_queue[QUEUEMAX],int *queue_cnt);
struct RCB handle_request_arrival_sstf(struct RCB request_queue[QUEUEMAX],int *queue_cnt, struct RCB current_request, struct RCB new_request, int timestamp);
struct RCB handle_request_completion_sstf(struct RCB request_queue[QUEUEMAX],int *queue_cnt,int current_cylinder);
struct RCB handle_request_arrival_look(struct RCB request_queue[QUEUEMAX],int *queue_cnt, struct RCB current_request, struct RCB new_request, int timestamp);
struct RCB handle_request_completion_look(struct RCB request_queue[QUEUEMAX],int *queue_cnt, int current_cylinder, int scan_direction);
struct MEMORY_BLOCK best_fit_allocate(int request_size, struct MEMORY_BLOCK memory_map[MAPMAX],int *map_cnt, int process_id);
struct MEMORY_BLOCK first_fit_allocate(int request_size, struct MEMORY_BLOCK memory_map[MAPMAX],int *map_cnt, int process_id);
struct MEMORY_BLOCK worst_fit_allocate(int request_size, struct MEMORY_BLOCK memory_map[MAPMAX],int *map_cnt, int process_id);
struct MEMORY_BLOCK next_fit_allocate(int request_size, struct MEMORY_BLOCK memory_map[MAPMAX],int *map_cnt, int process_id, int last_address);
void release_memory(struct MEMORY_BLOCK freed_block, struct MEMORY_BLOCK memory_map[MAPMAX],int *map_cnt);
int process_page_access_fifo(struct PTE page_table[TABLEMAX],int *table_cnt, int page_number, int frame_pool[POOLMAX],int *frame_cnt, int current_timestamp);
int count_page_faults_fifo(struct PTE page_table[TABLEMAX],int table_cnt, int refrence_string[REFERENCEMAX],int reference_cnt,int frame_pool[POOLMAX],int frame_cnt);
int process_page_access_lru(struct PTE page_table[TABLEMAX],int *table_cnt, int page_number, int frame_pool[POOLMAX],int *frame_cnt, int current_timestamp);
int count_page_faults_lru(struct PTE page_table[TABLEMAX],int table_cnt, int refrence_string[REFERENCEMAX],int reference_cnt,int frame_pool[POOLMAX],int frame_cnt);
int process_page_access_lfu(struct PTE page_table[TABLEMAX],int *table_cnt, int page_number, int frame_pool[POOLMAX],int *frame_cnt, int current_timestamp);
int count_page_faults_lfu(struct PTE page_table[TABLEMAX],int table_cnt, int refrence_string[REFERENCEMAX],int reference_cnt,int frame_pool[POOLMAX],int frame_cnt);
struct PCB handle_process_arrival_pp(struct PCB ready_queue[QUEUEMAX], int *queue_cnt, struct PCB current_process, struct PCB new_process, int timestamp);
struct PCB handle_process_completion_pp(struct PCB ready_queue[QUEUEMAX], int *queue_cnt, int timestamp);
struct PCB handle_process_arrival_srtp(struct PCB ready_queue[QUEUEMAX], int *queue_cnt, struct PCB current_process, struct PCB new_process, int time_stamp);
struct PCB handle_process_completion_srtp(struct PCB ready_queue[QUEUEMAX], int *queue_cnt, int timestamp);
struct PCB handle_process_arrival_rr(struct PCB ready_queue[QUEUEMAX], int *queue_cnt, struct PCB current_process, struct PCB new_process, int timestamp, int time_quantum);
struct PCB handle_process_completion_rr(struct PCB ready_queue[QUEUEMAX], int *queue_cnt, int timestamp, int time_quantum);
This lab project addresses the implementation of CPU-scheduling algorithms in an operating system. Each process in an operating system is managed by using a data structure called the Process Control Block (PCB). A PCB contains the process ID, arrival timestamp, total burst time, execution start time, execution end time, remaining burst time and the priority of the process in the system. The PCB class is defined as follows and is accessible from the rest of the code in this lab project: struct PCB \{ int process_id; int arrival_timestamp; int total_bursttime; int execution_starttime; int execution_endtime; int remaining_bursttime; int process_priority; \} The set of processes in the operating system that are ready to execute are maintained in a data structure called the Ready Queue. This data structure is an array of PCBs of the processes that are waiting for the CPU to become available so that they can execute. The NULLPCB is defined as [PID:O, AT:O, TBT:O, EST:O, EET:O, RBT:O, Priority:0] To determine the schedule of execution for the processes in an operating system, we consider three policies: - Priority-based Preemptive Scheduling (PP) - Shortest-Remaining-Time-Next Preemptive Scheduling (SRTP) - Round-Robin Scheduling (RR) In order to implement the above policies, we need to develop methods that handle arrival of processes for execution and the completion of process execution. Whenever a process arrives for execution, it can either join the ready queue and wait for its chance to execute or execute immediately (if there is no other process currently executing or if the currently-running process can be preempted). Whenever a process completes execution, another process from the ready queue is chosen to execute next, based on the scheduling policy. The details of these methods are described below in the specification and you need to develop code for these functions that follow the specification and place the code in a file named cpu.c. You should include the ostabs,h file. handle_process_arrival_pp This function implements the logic to handle the arrival of a new process in a Priority-based Preemptive Scheduler. Specifically, it takes five inputs: 1. the ready queue (an array of PCB structs) 2. The number of items in the ready queue 3. the PCB of the currently running process 4. the PCB of the newly arriving process 5. the current timestamp. The method determines the process to execute next and returns its PCB. If there is no currently-running process (i.e., the third argument is the NULLPCB), then the method returns the PCB of the newly-arriving process, indicating that it is the process to execute next. In this case, the PCB of the new process is modified so that the execution start time is set to the current timestamp, the execution end time is set to the sum of the current timestamp and the total burst time and the remaining burst time is set to the total burst time. If there is a currently-running process, the method compares the priority of the newly-arriving process with the currently-running process. If the new process has equal or lower priority (smaller integers for the priority field in the PCB indicate higher priority), then its PCB is simply added to the ready queue and the return value is the PCB of the currently-running process. As the newly-arriving process is added to the ready queue, its execution start time and execution end time are set to 0 , and the remaining burst time is the same as its total burst time. If the new process has a higher priority, then the PCB of the currently-running process is added to the ready queue and the return value is the PCB of the new process. In this case, the PCB of the new process is modified so that the execution start time is set to the current timestamp, the execution end time is set to the sum of the current timestamp and the total burst time and the remaining burst time is set to the total burst time. Also, the PCB of the currently-running process is added to the ready queue after marking its execution end time as 0 , and adjusting its remaining burst time. The signature of the method is as follows: struct PCB handle_process_arrival_pp(struct PCB ready_queue[QUEUEMAX], int *queue_cnt, struct PCB current_process, struct PCB new_process, int timestamp); Please refer to Section 2.4 of the Modern Operating Systems book for a detailed discussion of the Priority-based algorithm. This method implements the logic to handle the completion of execution of a process in a Priority-based Preemptive Scheduler. Specifically, it takes three inputs: 1. the ready queue (an array of PCB structs) 2. The number of items in the ready queue 3. the current timestamp. The method determines the process to execute next and returns its PCB. If the ready queve is empty, the method returns the NULLPCB, indicating that there is no process to execute. Otherwise, the method finds the PCB of the process in the ready queue with the highest priority (smaller integers for the priority field in the PCB mean higher priorities), removes this PCB from the ready queue and returns it. Before returning the PCB of the next process to execute, it is modified to set the execution start time as the current timestamp and the execution end time as the sum of the current timestamp and the remaining burst time. The signature of the method is as follows: struct PCB handle_process_completion_Pp(struct PCB ready_queue[QUEUEMAX], int *queue_cnt, int timestamp); Please refer to Section 2.5.3 of the Modern Operating Systems book for a detailed discussion of the Priority-based algorithm. handle_process_arrival_srtp This method implements the logic to handle the arrival of a new process in a Shortest-Remaining-Time-Next Preemptive Scheduler. Specifically, it takes five inputs: 1. the ready queue (an array of PCB structs) 2. The number of items in the ready queue 3. the PCB of the currently-running process 4. the PCB of the newly-arriving process 5. the current timestamp. The method determines the process to execute next and returns its PCB. If there is no currently-running process (i.e., the third argument is the NULLPCB), then the method returns the PCB of the newly-arriving process, indicating that it is the process to execute next. In this case, the PCB of the new process is modified so that the execution start time is set to the current timestamp, the execution end time is set to the sum of the current timestamp and the total burst time and the remaining burst time is set to the total burst time. If there is a currently-running process, the method compares the remaining burst time of the currently-running process and the total burst time of the newly-arriving process. If the new process does not have a shorter burst time, then its PCB is simply added to the ready queue and the return value is the PCB of the currently running process. As the newly-arriving process is added to the ready queue, its execution start time and execution end time are set to 0 , and the remaining burst time is set to the total burst time. If the new process has a shorter burst time, then the PCB of the currently-running process is added to the ready queue and the return value is the PCB of the new process. In this case, the PCB of the new process is modified so that the execution start time is set to the current timestamp, the execution end time is set to the sum of the current timestamp and the total burst time and the remaining burst time is set to the total burst time. Also, the PCB of the currently-running process is added to the ready queue, after marking its execution start time and execution end time as 0 , and adjusting its remaining burst time. The signature of the method is as follows: struct PCB handle_process_arrival_srtp(struct PCB ready_queue[QUEUEMAX], int queue_cnt, struct PCB current_process, struct PCB new_process, int time_stamp); This method implements the logic to handle the completion of execution of a process in a Shortest-Remaining-Time Preemptive Scheduler. Specifically, it takes three inputs: 1. the ready queue (an array of PCB structs) 2. The number of items in the ready queue 3. the current timestamp. The method determines the process to execute next and returns its PCB. If the ready queue is empty, the method returns the NULLPCB, indicating that there is no process to execute next. Otherwise, the method finds the PCB of the process in the ready queue with the smallest remaining burst time, removes this PCB from the ready queue and returns it. Before returning the PCB of the next process to execute, it is modified to set the execution start time as the current timestamp and the execution end time as the sum of the current timestamp and the remaining burst time. The signature of the method is as follows: struct PCB handle_process_completion_srtp(struct PCB ready_queue[QUEUEMAX], int "queue_cnt, int timestamp); This method implements the logic to handle the completion of execution of a process in a Shortest-Remaining-Time Preemptive Scheduler. Specifically, it takes three inputs: 1. the ready queue (an array of PCB structs) 2. The number of items in the ready queue 3. the current timestamp. The method determines the process to execute next and returns its PCB. If the ready queue is empty, the method returns the NULLPCB, indicating that there is no process to execute next. Otherwise, the method finds the PCB of the process in the ready queue with the smallest remaining burst time, removes this PCB from the ready queue and returns it. Before returning the PCB of the next process to execute, it is modified to set the execution start time as the current timestamp and the execution end time as the sum of the current timestamp and the remaining burst time. The signature of the method is as follows: struct PCB handle_process_completion_srtp(struct PCB ready_queue[QUEUEMAX], int "queue_cnt, int timestamp); Please refer to Section 2.4 of the Modern Operating Systems book for a detailed discussion of the Shortest-Remaining-Time-Next algorithm. handle_process_arrival_rr This method implements the logic to handle the arrival of a new process in a Round-Robin Scheduler. Specifically, it takes six inputs: 1. the ready queue (an array of PCB structs) 2. The number of items in the ready queue 3. the PCB of the currently-running process 4. the PCB of the newly-arriving process 5. the current timestamp 6. the time quantum. The method determines the process to execute next and returns its PCB. If there is no currently-running process (i.e., the third argument is the NULLPCB), then the method returns the PCB of the newly-arriving process, indicating that it is the process to execute next. In this case, the PCB of the new process is modified so that the execution start. time is set to the current timestamp, the execution end time is set to the sum of the current timestamp and the smaller of the time quantum and the total burst time. The remaining burst time is set to the total burst time. If there is a currently-running process, the method simply adds the PCB of the newly-arriving process to the ready queue and the return value is the PCB of the currently running process. As the newly-arriving process is added to the ready queue, its execution start time and execution end time are set to 0 , and the remaining burst time is set to the total burst time. The signature of the method is as follows: struct PCB handle_process_arrival_rr(struct PCB ready_queue[QUEUEMAX], int *queue_cnt, struct PCB current_process, struct PCB new_process, int timestamp, int time_quantum); A sample execution input and output: Please refer to Section 2.4 of the Modern Operating Systems book for a detailed discussion of the Round-Robin algorithm. Please refer to Section 2.4 of the Modern Operating Systems book for a detailed discussion of the Round-Robin algorithm
Step by Step Solution
There are 3 Steps involved in it
Step: 1
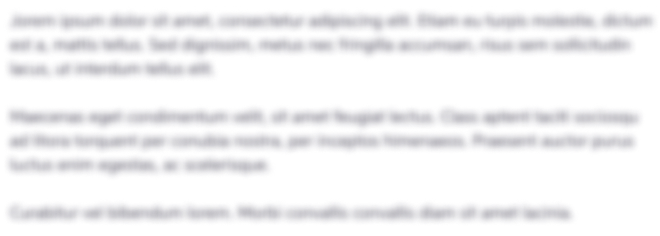
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started