Question
Need help ASAP please. Implement C++ Linked List. Please read through all of documentation. Starter files below. ll.h #ifndef LL_H #define LL_H #include #include namespace
Need help ASAP please. Implement C++ Linked List. Please read through all of documentation. Starter files below.
ll.h
#ifndef LL_H
#define LL_H
#include
#include
namespace cs126linkedlist {
// Template linked list class
templatetypename ElementType>
class LinkedList {
// You may add anything here you need to implement the linked list.
// You will probably want to define the LinkedListNode class here.
public:
LinkedList(); // Default constructor
explicit LinkedList(const std::vector
// Big 5
LinkedList(const LinkedList& source); // Copy constructor
LinkedList(LinkedList&& source) noexcept; // Move constructor
~LinkedList(); // Destructor
LinkedList
LinkedList
void push_front(ElementType value); // Push value on front
void push_back(ElementType value); // Push value on back
ElementType front() const; // Access the front value
ElementType back() const; // Access the back valueW
void pop_front(); // remove front element
void pop_back(); // remove back element
int size() const; // return number of elements
bool empty() const; // check if empty
void clear(); // clear the contents
void RemoveNth(int n); // remove the Nth element from the front 0 indexed
bool operator==(const LinkedList
// iterator
class iterator : std::iterator<:forward_iterator_tag elementtype> {
// You may add any data you need here
public:
iterator() : current_(nullptr) {};
// You may add any constructors you want here
iterator& operator++();
ElementType& operator*();
bool operator!=(const iterator& other);
};
iterator begin();
iterator end();
// const_iterator
class const_iterator : std::iterator<:forward_iterator_tag elementtype> {
// You may add any data you need here
public:
const_iterator() : current_(nullptr) {};
// You may add any constructors you want here
const_iterator& operator++();
const ElementType& operator*();
bool operator!=(const const_iterator& other);
};
const_iterator begin() const;
const_iterator end() const;
};
templatetypename ElementType>
std::ostream& operatorconst LinkedList
// needed for template instantiation
#include "ll.cpp"
} // namespace cs126linkedlist
#endif //LL_H
ll.cpp
// You can add any helper functions or headers that you need.
// You should also fill in all the function bodies.
templatetypename ElementType>
LinkedList
}
templatetypename ElementType>
LinkedList
}
// Copy constructor
templatetypename ElementType>
LinkedList
}
// Move constructor
templatetypename ElementType>
LinkedList
}
// Destructor
templatetypename ElementType>
LinkedList
}
// Copy assignment operator
templatetypename ElementType>
LinkedList
}
// Move assignment operator
templatetypename ElementType>
LinkedList
}
templatetypename ElementType>
void LinkedList
}
templatetypename ElementType>
void LinkedList
}
templatetypename ElementType>
ElementType LinkedList
}
templatetypename ElementType>
ElementType LinkedList
}
templatetypename ElementType>
void LinkedList
}
templatetypename ElementType>
void LinkedList
}
templatetypename ElementType>
int LinkedList
}
templatetypename ElementType>
bool LinkedList
}
templatetypename ElementType>
void LinkedList
}
templatetypename ElementType>
std::ostream& operatorconst LinkedList
}
templatetypename ElementType>
void LinkedList
}
templatetypename ElementType>
bool LinkedList
}
templatetypename ElementType>
bool operator!=(const LinkedList
}
templatetypename ElementType>
typename LinkedList
}
templatetypename ElementType>
ElementType& LinkedList
}
templatetypename ElementType>
bool LinkedList
}
templatetypename ElementType>
typename LinkedList
}
templatetypename ElementType>
typename LinkedList
}
templatetypename ElementType>
typename LinkedList
}
templatetypename ElementType>
const ElementType& LinkedList
}
templatetypename ElementType>
bool LinkedList
}
templatetypename ElementType>
typename LinkedList
}
templatetypename ElementType>
typename LinkedList
}
1.1 Intro In this assignment you will create a container class that implements a linked list class that is templated so that it can contain any data type you want 1.2 Provided Files We are providing you with two source files . 11.h- You may not change the public interface of this class. You may add constructors to the iterator class and the const_iterator though you may not add anything else to the public interface of these classes 11.cpp - You should put all the function implementations in this file You may add any files you need to test or develop the class but all code to implement the LinkedList class must be contained in the two provided files 2 Linked List A linked list is a very standard data structure in computer science. It is designed to efficiently use use space when compared to an array. In a linked list you can remove an element irom the list without having to copy other data as you would need to do in an array This data structure is implemented with a head pointer that points to the first element in the list with each element pointing to the next and finally when all items are done pointing to null. This can be seen in Figure 1 head 2 Figure 1. Linked List The most basic operation in a linked list is to insert a node at the front of the list. This is done using the following steps Create a new list node containing the item to be inserted Update the next pointer in the new list node to point at the same node as head pointed to . Change the head node to point to the new node 1.1 Intro In this assignment you will create a container class that implements a linked list class that is templated so that it can contain any data type you want 1.2 Provided Files We are providing you with two source files . 11.h- You may not change the public interface of this class. You may add constructors to the iterator class and the const_iterator though you may not add anything else to the public interface of these classes 11.cpp - You should put all the function implementations in this file You may add any files you need to test or develop the class but all code to implement the LinkedList class must be contained in the two provided files 2 Linked List A linked list is a very standard data structure in computer science. It is designed to efficiently use use space when compared to an array. In a linked list you can remove an element irom the list without having to copy other data as you would need to do in an array This data structure is implemented with a head pointer that points to the first element in the list with each element pointing to the next and finally when all items are done pointing to null. This can be seen in Figure 1 head 2 Figure 1. Linked List The most basic operation in a linked list is to insert a node at the front of the list. This is done using the following steps Create a new list node containing the item to be inserted Update the next pointer in the new list node to point at the same node as head pointed to . Change the head node to point to the new nodeStep by Step Solution
There are 3 Steps involved in it
Step: 1
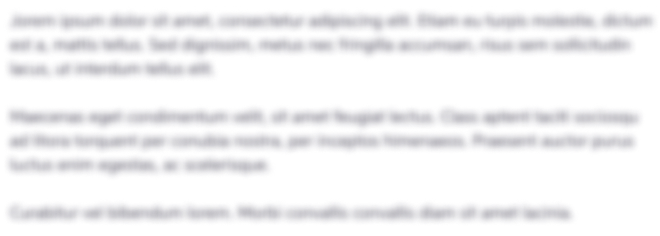
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started