Question
Need help debugging almost done. Create an application that reads a file that contains an email list, reformats the data, and writes the cleaned list
Need help debugging almost done.
Create an application that reads a file that contains an email list, reformats the data, and writes the cleaned list to another file.
there is file named prospects.csv that contains a list of prospects.
Your application should fix the formatting problems and write a file named prospects_clean.csv.
All names should use title case (an initial capital letter with the rest lowercase).
All email addresses should be lowercase.
All extra spaces at the start or end of a string should be removed.
FileCleanerApp Class:
import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import java.io.PrintWriter; import java.util.ArrayList;
public class FileCleanerApp {
private static String inputFilename = "prospects.csv"; private static String outputFilename = "prospects_clean.csv"; public static void main(String args[]) { System.out.println("File Cleaner"); System.out.println(); System.out.println("Source file: " + inputFilename); System.out.println("Cleaned file: " + outputFilename); System.out.println(); cleanFile(); System.out.println("Congratulations! Your file has been cleaned"); } private static void cleanFile() { ArrayList
// read input file try (BufferedReader in = new BufferedReader( new FileReader(inputFilename))) { String line = in.readLine(); while (line != null) { lines.add(line); line = in.readLine(); } } catch (IOException e) { System.err.println(e); } // write output file try (PrintWriter out = new PrintWriter( new BufferedWriter( new FileWriter(outputFilename)))) {
// clean the data for (String line : lines) { String[] prospect = line.split(",");
String firstName = prospect[0].trim(); firstName = Stringutil.toTitleCase(firstName);
String lastName = prospect[1].trim(); lastName = StringUtil.toTitleCase(lastName);
String email = prospect[2].toLowerCase(); out.println(firstName + "," + lastName + "," + email); } } catch (IOException e) { System.err.println(e); } } }
//*********************************************************************************************************
StringUtil Class:
public class StringUtil { public static String toTitlecase(String name) { String nameTitleCase = name.substring(0, 1).toLowerCase() + name.substring(1).toUpperCase(); return nameTitleCase; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
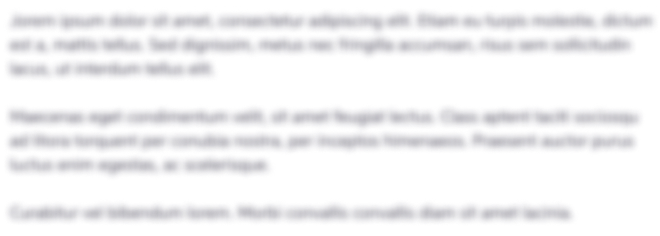
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started