Question
Need help for an assignment I need to write in Java! I get some of it but I'm really struggling with the language and I'm
Need help for an assignment I need to write in Java! I get some of it but I'm really struggling with the language and I'm getting overwhelmed. Here is the assignment I am working on.
Write a quiz program that will:
Welcome the user to your quiz program
Prompt the user for their name and then collect that name and store it in an appropriately named variable. The entire name must be collected at once. Consider using the nextLine method of the Scanner class in case the user includes spaces.
Thank the user by name (the name they supplied) and tell them to get ready to take the quiz
Provide the user with 5 separate quiz questions that are all related to the same subject. For example, you could create a quiz that asks 5 trivia questions about a movie you enjoy.
Each question will have a question and between 3 and 4 possible answers. Each possible answer will appear on a different line and it will be labeled with a letter. For example, the first answer should be A and the second answer should be B.
It is up to you what the subject is and what the correct answers are.
You must validate the value someone types in as an answer with a switch statement (do not use an if statement to do this).
A capital or lowercase letter will be considered a valid response. You may want to capitalize or lowercase the value the user typed in or you can check for both values.
If the user did not type in one of the valid letters, you will prompt the user that they typed in an invalid response, redisplay the question with the answers and re-read a response. This will require a separate loop for each question. I suggest a do..while loop, though a while loop will work as well. Only exit the loop after the user has typed in a valid response
Note: Early versions of Java did not allow Strings to be used in switch statements. Newer versions of Java do allow it.
Each answer will need to be considered for correctness. Dont confuse valid and correctness. The user can answer with a valid response that is incorrect. At the end of the program you will need to determine how many correct answers there were. So, I recommend a running counter (which keeps track of how many correct answers there are)
To display each question- You may use multiple println methods or one single println method with /n escape sequences included.
After you have collected the 5 answers from the user, output the number of questions they got correct out of 5. For example, they could have scored a 2 out of 5. The number should be based on the value stored in the variable.
On the next line, output 1 of 6 possible statements in regard to the score. Utilize a single if statement with multiple else if statements to handle the different output scenarios. For example, if they scored 0 out of 5, you may want to put a pity statement in there like, Well, that wasnt good. If they score a 4 out of 5, you may want to congratulate them on almost getting every question correct, etc. Use your creativity with the statements you come up with, but please keep the comments PG rated. No crass humor please.
After step 6, for every question the user got wrong, I want you to tell them what Question(s) they got wrong, what they answered (which should still be in a variable), and what the correct answer was. Then I want you to give a fun little fact about the correct answer. See my attached example to see what I am looking for.
a. Each of the 5 questions should be checked for this step and they should each have their own fun facts
b. Only output a fun fact for the questions that the user got wrong.
c. If the user got all the questions correct, then the program should skip these question corrections
Step by Step Solution
There are 3 Steps involved in it
Step: 1
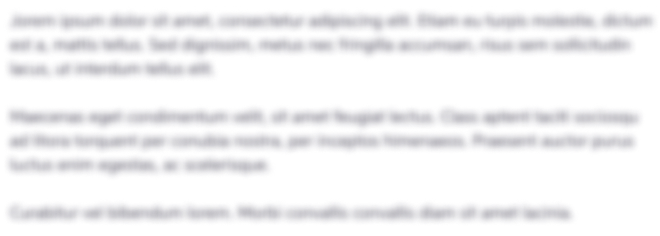
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started