Answered step by step
Verified Expert Solution
Question
1 Approved Answer
need help for coding Problem Specification You are asked to write a C program that allows the user to play with a machine. Before writing
need help for coding
Problem Specification You are asked to write a C program that allows the user to play with a machine. Before writing your program, you are asked to analyze a flowchart that will be provided to you during the lab meeting by the TA. Your code needs to follow the flowchart. PROGRAM: The goal is to write a program that implements a simple algorithm capable of guessing an integer number. It means, the program you write should be able to guess a number which you are thinking of. The program accomplishes that by asking for hints, i.e., asking if a number it has guessed is larger or smaller than the one you come up with. The program should execute the following: O ask the user for two values that defines the range for a number that the program has to guess ask for a minimum value of the range: min ask for a maximum value of the range: max' create a variable named 'counter' to store how many times the program has guessed the value of the number you (user) came up with create a number in the provided range, let us call it program_guessed_number', according to the following formula: min+max 2 display that number to the user o display a menu with the following options: 1. TOO LARGE 2. TOO SMALL 3. FOUND ask the user to select one of the options (via entering an option number: 1, 2 or 3) based on the option chosen by the user do: if it is TOO LARGE', then assign a new value to the variable max': 'max = program_guessed_number - 1', and increment the value of counter' o if it is 'TOO SMALL, then assign a new value to the variable min': *min = program_guessed_number + 1', and increment the value of 'counter o if FOUND, display the text: FOUND IT, display the value of counter, and exit the program This algorithm is called BINARY SEARCH, and it will always win a game in at most logzN tries, where N is a number of integer values in the range. So, for N = 1000000 it needs at most 20 tires. An example of interaction between the program and the user is shown below: Program displays: User's responses: (a number generated/came up with by a user is 23) Range: minimum 0 Range: maximum 100 Guessed number: 50 1. TOO LARGE 2. TOO SMALL 3. FOUND 1 Guessed number: 24 1. TOO LARGE 2. TOO SMALL 3. FOUND 1 Guessed number: 11 1. TOO LARGE 2. TOO SMALL 3. FOUND 2 Guessed number: 17 1. TOO LARGE 2. TOO SMALL 3. FOUND 2 Guessed number: 20 1. TOO LARGE 2. TOO SMALL 3. FOUND 2 Guessed number: 22 1. TOO LARGE 2. TOO SMALL 3. FOUND 2 Guessed number: 23 1. TOO LARGE 2. TOO SMALL 3. FOUND 3 FOUND IT No of tries: 7 Implementation Details It's important that you DESIGN your program FIRST!!!! In your code, please follow the logic of the flowchart provided to you. The whole program is just one function int main(void). We have not covered data structures in the class; therefore, all variables can be simple ones (like in the examples in the course slides). The program should use loop and selection statements: while, if-else (see below). Use comments in your program. A comment should describe a goal/purpose of the following few lines of code. The comment should not "copy" the lines of codes, but enable a better understating of what the code should do/accomplish. Please, use the terms and names defined in the flowchart. Naming Convention: please follow the following rules when you name your variables, and functions (in the next labs). 1) use names that describe what the variable represents, or function does, 2) decide a way of building a name and follow this rule in the whole code, for example, a function's name can be built using verb and object/result of the action - add_numbers (or addNumbers) then be consistent, it means to not have names like numbers multiplied but mult numbers (or multNumbers); 3) make the names short, you have to type them so be reasonable. A much more detailed description of coding conversion and program structure is contained in the document Coding Convention that is available on the eClass. Please download it, make yourself familiar with it, and follow it. Hints 1. Start with a simple version of the program: the first step can be just asking for a name, then the second step menu, then the third step one of the groups ... Such an approach is called "incremental development. 2. DO NOT DO EVERYTHING with the FIRST ATTEMPT. 3. Compile your program after adding a few statements. DO NOT wait till the end!!! 4. Use the debugger!!! It is the best tool to verify correctness of your program. 5. DO NOT use the instruction GOTO! Useful information/functions Control structure while while (condition) { i This instruction works in the same way as while in MATLABC. It is a loop that is executed as long as the condition is evaluated to true. Please note usage of brackets both ( ) and { }. #includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
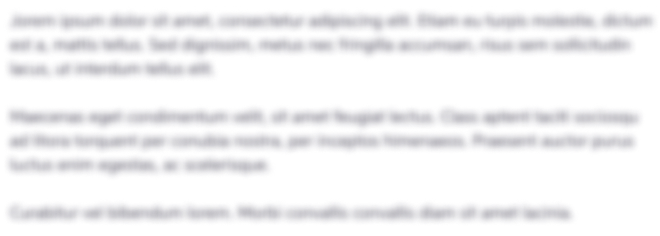
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started