Question
Need help for JAVA lab that takes a infix expression and converts it to postfix notation then evaluates it. Especially the Error Checking covers 4
Need help for JAVA lab that takes a infix expression and converts it to postfix notation then evaluates it.
Especially the Error Checking covers 4 cases: adjacent operators, adjacent operands, missing parenthesis and Extra parenthesis.
Error checking needs to output that the expression is incorrect and then evaluate the next expression for validity. My idea was to assign a false value to those expression that when sent to the evaluate class which would determine if the expression was valid and if not exit the class
anyway Heres My Code and input Data:
8 + 4 * 2 - 6 7 * 2 - 4 * 3 + 2 * 5 2 * 3 * 4 - 8 + 9 / 3 / 3 5 + 7 * 4 - 6 4 * ( 3 + 2 * 4 ) - 7 ( 5 + 7 ) / ( 9 - 5 ) 3 * ( 5 * ( 5 - 2 ) ) - 9 ( ( 5 * ( 4 + 2 ) - ( 8 + 8 ) / 2 ) - 9 ) ^ 3 ( ( 5 + 5 * ( 6 - 2 ) + 4 ^ 2 ) * 8 ) ( ( ( 3 ^ 4 ) ) )
1 + * 2 1 2 * 3 ^ 4 ( 1 + 2 ( 1 + 2 ) * 3 )
/** * ObjectStackInterface - contains all public methods in the ObjectStack class * * @author Josh Mumford * @version 09/17/17 */ public interface ObjectStackInterface { public boolean isEmpty(); public void clear(); public void push(Object o); public Object pop(); public Object top(); }
/** * ObjectStack - creates a stack object that has methods to change it size, check if * the stack is empty or full, clear the stack, push or remove a value from the * stack and return the value at the top of the stack */ public class ObjectStack implements ObjectStackInterface { private Object[] item; private int top; public ObjectStack() { item = new Object[1]; top = -1; }
public boolean isEmpty() { return top == -1; }
public boolean isFull() { return top == item.length-1; } public void clear() { item = new Object[1]; top = -1; } public void push(Object o) { if (isFull()) resize(2 * item.length); item[++top] = o; } private void resize(int size) { Object[] temp = new Object[size]; for (int i = 0; i <= top; i++) temp[i] = item[i]; item = temp; } public Object pop() { if (isEmpty()) { System.out.println("Stack Underflow."); System.exit(1); } Object temp = item[top]; item[top--] = null; if (top == item.length/4) resize(item.length/2); return temp; } public Object top() { if (isEmpty()) { System.out.println("Stack Underflow."); System.exit(1); } return item[top]; } }
import java.io.*; import java.util.Scanner;
/** * Driver - reads data from a file and parses through until EOF calling other classes to convert an * infix expression to a Postfix expression and evaluating the expression that is returned. * creates an ouput file that recieves both data from input file and output from conversion * and evaluation * * @author Josh Mumford * @version 09/14/2017 */ public class Driver { public static void main(String[] args) throws IOException { Scanner filescan = new Scanner(new File("infix.txt")); PrintWriter pw = new PrintWriter(new FileWriter("csis.txt")); EvalPostfix eval = new EvalPostfix(pw); InfixToPostfix infix = new InfixToPostfix(pw); try{ while (filescan.hasNextLine()) { String line = filescan.nextLine(); String postfix = infix.infixToPostfix(line); int value = eval.evalPostfix(postfix); System.out.println(line + " Postfix:" + postfix + " Value:" + value); pw.println(line + " Postfix:" + postfix + " Value:" + value); } filescan.close(); pw.close(); } catch(ParenthesisMismatchException ex){ System.out.println(ex.getMessage()); } catch(InvalidExpressionException num){ System.out.println(num.getMessage()); } catch(MissingParenthesisException miss) { System.out.println(miss.getMessage()); } } }
import java.io.*; import java.util.Scanner;
/** * InfixToPostfix - takes a infix expression represented as a string & converts the * expression to postfix notation * * @author Josh Mumford * @version 09/21/17 */ public class InfixToPostfix { // instance variables private ObjectStack operator; private PrintWriter pw; /** * InfixToPostfix - initializes all instance variables * * @param pw passes PrintWriter object from driver class * */ public InfixToPostfix(PrintWriter pw) { // initialise instance variables this.pw = pw; operator = new ObjectStack(); }
/** * priority() - takes an operator represented as a char, returning a priority number * for each case * * @param op a character that represents operators * @return int returns an integer */ private int priority(char op) { switch(op) { case '^': return 3; case '*': case '/': return 2; case '+': case '-': return 1; default : return 0; } } /** * convertToPostfix() - contains all methods neccessary to convert a infix expression to postfix * * @param infix reference to the argument line in driver * @return String the method returns a string value */ public String infixToPostfix(String infix) throws ParenthesisMismatchException, InvalidExpressionException, MissingParenthesisException { StringBuilder post = new StringBuilder(); for(int i = 0; i < infix.length(); i++) { char ch = infix.charAt(i); if (ch >= '0' && ch <= '9'){ if(i < (infix.length() - 1) && (infix.charAt(i + 1) >= '0' && infix.charAt(i + 1) <= '9')) {throw new InvalidExpressionException("invalid infix expression: adjacent operands");} post.append(ch); } else if (ch == '('){ operator.push(ch); } else if (ch == '+' || ch == '-' || ch == '*' || ch == '/' || ch == '^') { if (i < (infix.length() - 1) && (infix.charAt(i + 1) == '+' || infix.charAt(i + 1) == '^' || infix.charAt(i + 1) == '-' || infix.charAt(i + 1) == '*' || infix.charAt(i + 1) == '/' )) { throw new InvalidExpressionException("invalid infix expression: adjacent operators");} if (operator.isEmpty() || priority(ch) > priority((Character)operator.top())) operator.push(ch); else { while(!operator.isEmpty() && priority(ch) <= priority((Character)operator.top())) { post.append((Character)operator.pop()); } operator.push(ch); } } else if (ch == ')') { while (!operator.isEmpty() && (Character)operator.top() != '(') { post.append((Character)operator.pop()); if (operator.isEmpty()) { throw new ParenthesisMismatchException("invalid expression: extra parenthesis"); } if (!operator.isEmpty()) operator.pop(); } } } while (!operator.isEmpty()) { //try if top of stack is ( then its a invalid expression if((Character)operator.top() == '(') { throw new MissingParenthesisException("invalid expression: missing parentthesis"); } post.append((Character)operator.pop()); } return post.toString(); } }
import java.io.*;
/** * EvalPostFix - takes an arithmetic expression (string) and converts the expression from infix * notation to postfix notation, returning the expression * * @author Josh Mumford * @version 09/17/17 */ public class EvalPostfix { private PrintWriter pw; private ObjectStack operand; private int value;
/** * EvalPostfix - initializes all instance variables * * @param pw passes the pw object from driver class */ public EvalPostfix(PrintWriter pw) { this.pw = pw; operand = new ObjectStack(); }
/** * evalPostExp() - evaluates a (string) postfix expression, returns it as an integer and * prints that number to the output file * * @param exp passes a reference to the actual parameter * @return int returns a integer value */ public int evalPostfix(String exp) { for(int i = 0; i < exp.length(); i++) { char ch = exp.charAt(i); if (ch >= '0' && ch <= '9') operand.push(ch - 48); else if (ch == '+' || ch == '-' || ch == '*' || ch == '/' || ch == '^') { int val2 = (Integer)operand.pop(); int val1 = (Integer)operand.pop(); if (ch == '+') operand.push(val1 + val2); else if (ch == '-') operand.push(val1 - val2); else if (ch == '*') operand.push(val1 * val2); else if (ch == '/') operand.push(val1 / val2); else if (ch == '^') operand.push((int) Math.pow(val1, val2)); } } return (Integer)operand.pop(); } }
/** * Write a description of class MissingParenthesisException here. * * @author (your name) * @version (a version number or a date) */ public class MissingParenthesisException extends Exception { /** * Constructor for objects of class MissingParenthesisException */ public MissingParenthesisException(String message) { super(message); }
}
/** * Write a description of class InvalidExpression here. * * @author (your name) * @version (a version number or a date) */ public class ParenthesisMismatchException extends Exception { public ParenthesisMismatchException(String message) { super(message); } }
/** * Write a description of class InvalidExpression here. * * @author (your name) * @version (a version number or a date) */ public class ParenthesisMismatchException extends Exception { public ParenthesisMismatchException(String message) { super(message); } }
/** * Write a description of class InvalidExpressionException here. * * @author (your name) * @version (a version number or a date) */ public class InvalidExpressionException extends Exception { public InvalidExpressionException(String message2) { super(message2); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
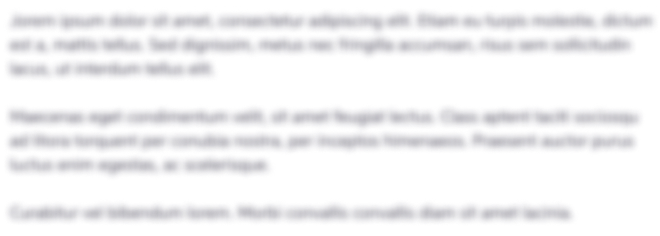
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started