Question
need help in C++: Sorting is one of the most broadly used fundamental operations in data processing. There are numerous sorting algorithms available. In this
need help in C++: Sorting is one of the most broadly used fundamental operations in data processing. There are numerous sorting algorithms available. In this project, you are asked to modify the existing algorithms for the real-world applications. You are required to modify three sorting algorithms for solving this problem: Two O(n log n) algorithms (merge sort and quick sort), and one algorithm (your choice). Project Objective: In completing this project, you will further develop your ability to 1. Simplify and solve real world problems; 2. Apply the existing algorithms into real world applications; 3. Modify and implement algorithmic approaches; 4. Apply knowledge of asymptotic complexity analysis in algorithm selection. This can be done in any language but C++ is prefered Also, just imagine there are 5 arrays of length n for the project. I have sources but I don't need help with file part. Thanks here is the code I got however the inversions keep becoming 0
//#include "stdafx.h"
//#include
//#include
//#include
//#include
//#include
//using namespace std;
//// Qucksort method //
//int InvCount(int arr[], int n)
//{ // int inversion_count = 0;
// for(int i = 0; i < n - 1; i++)
{
// arr[i];
// for(int j = i + 1; j> source1[n]; // n++; // }
// // //
cout << "The number of inversions in source 1 is: " << InvCount(source1, sz) << endl;
// File.close();
// cout << endl; // // File.open("source2.txt");
// // while (!File.eof())
// {
// File >> source2[n];
// n++; //
}
// cout << "The number of inversions in source 2 is: " << InvCount(source2, sz) << endl;
// File.close();
// cout << endl; //
//
// File.open("source3.txt");
// while (!File.eof())
// {
// File >> source3[n];
// n++;
// }
// cout << "The number of inversions in source 3 is: " << InvCount(source3, sz) << endl;
// File.close();
// cout << endl;
//
// File.open("source4.txt");
// while (!File.eof())
// {
// File >> source4[n];
// n++;
// }
// cout << "The number of inversions in source 4 is: " << InvCount(source4, sz) << endl;
// File.close();
// cout << endl;
//
// File.open("source5.txt");
// while (!File.eof())
// {
// File >> source5[n];
// n++;
// }
// cout << "The number of inversions in source 5 is: " << InvCount(source5, sz) << endl;
// File.close();
// return 0;
//
//}
//merge sort method;
#include "stdafx.h"
#include
#include
#include
using namespace std;
//int InvCount(int arr[], int n)
//{
// int inversion_count = 0;
// for(int i = 0; i < n - 1; i++) {
// arr[i];
// for(int j = i + 1; j left) {
//cout << "debug if _mergeFunc";
mid = (right + left) / 2;
Inversion_count = _mergeFunc(array, Temporary, left, mid);
Inversion_count += _mergeFunc(array, Temporary, mid + 1, right);
} return Inversion_count;
} int merge(int array[], int Temporary[], int left, int mid, int right)
{
int i, j, k;
int inversion_count = 0;
i = left;
j = mid;
k = left;
while ((i <= mid - 1) && (j <= right))
{
if (array[i] <= array[j])
{
Temporary[k++] = array[j++];
for (i = left; i <= right; i++)
{
array[i] = Temporary[i]; return inversion_count;
}
}
}
}
void setArray(int arr[], int sz)
{
for (int i = 0; i < sz; i++)
{ arr[i] = 0; }
}
// main program
int main() {
const int sz = 10000;
int source1[sz];
setArray(source1, sz);
int source2[sz];
setArray(source1, sz);
int source3[sz];
setArray(source1, sz);
int source4[sz];
setArray(source1, sz);
int source5[sz];
setArray(source5, sz);
int n=0;
ifstream File;
File.open("source1.txt");
if (File)
{
cout << "debug file is open" << endl;
while (!File.eof())
{
File >> source1[n]; n++;
}
/*for (int i = 0; i < sz; i++)
{
cout << source1[i]<< ", ";
}*/
cout << "The number of inversions in source 1 is: " << mergeFunc(source1, sz) << endl;
File.close();
cout << endl; }
else { cout << "error opening file" << endl;
}
return 0; }
// //// Bubble sort code //
//void bubbleSort(int arr[], int n) //
{ // int i, j;
// for (i = 0; i < n - 1; i++)
// //
// Last i elements are already in place
// for (j = 0; j < n - i - 1; j++)
// if (arr[j] > arr[j + 1])
// swap(&arr[j], &arr[j + 1]);
//}
////// I separated the code the best I could
need to review my code:
We are doing an algorithm assignment that takes: mergesort, Quicksort, and one of your choice(bubblesort) and counts the number of inversions of each one out of 10,000 numbers. I believe its getting stuck in the _mergeFunc function.
I need code that will tell me the inversion counts of the following:
merge sort.
Quick sort.
Bubble sort
Step by Step Solution
There are 3 Steps involved in it
Step: 1
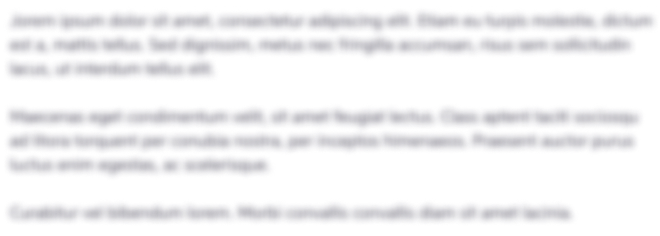
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started