Need help solving this Java Program
Instructions:
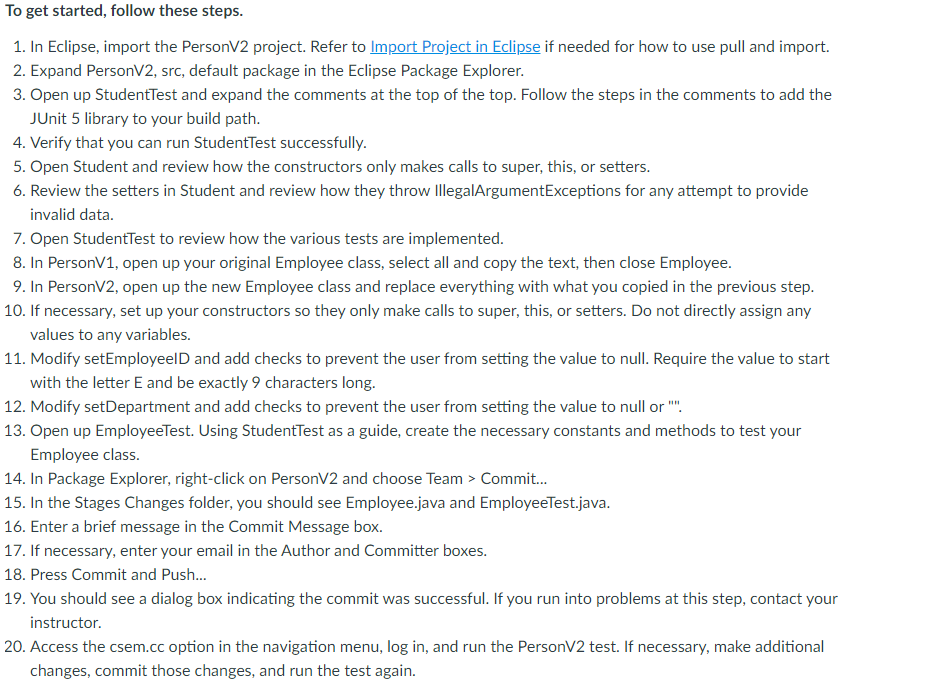
EmployeeTest.java
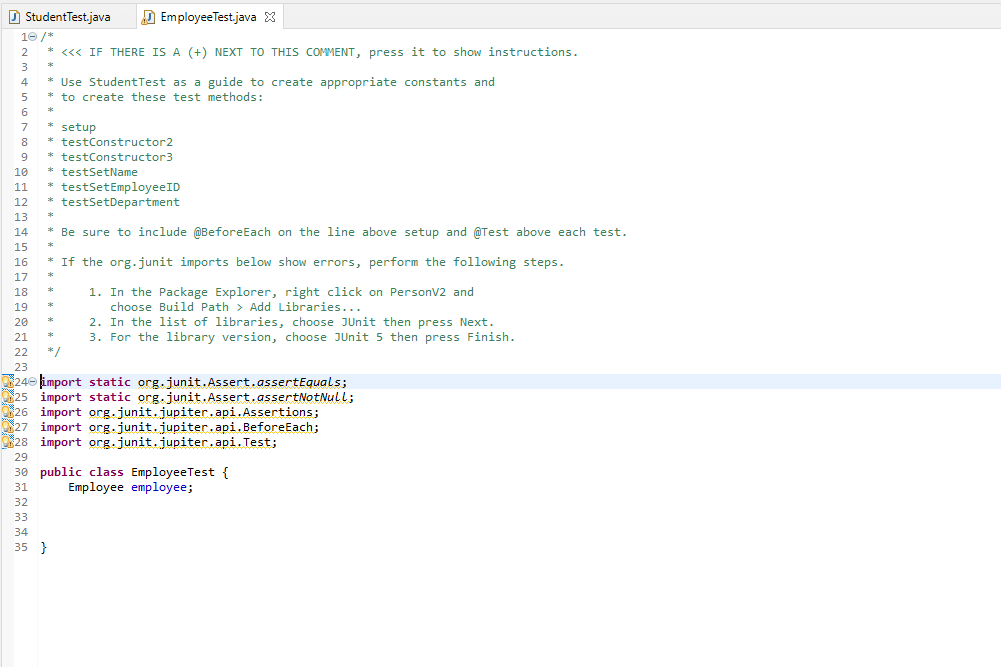
StudentTest.Java
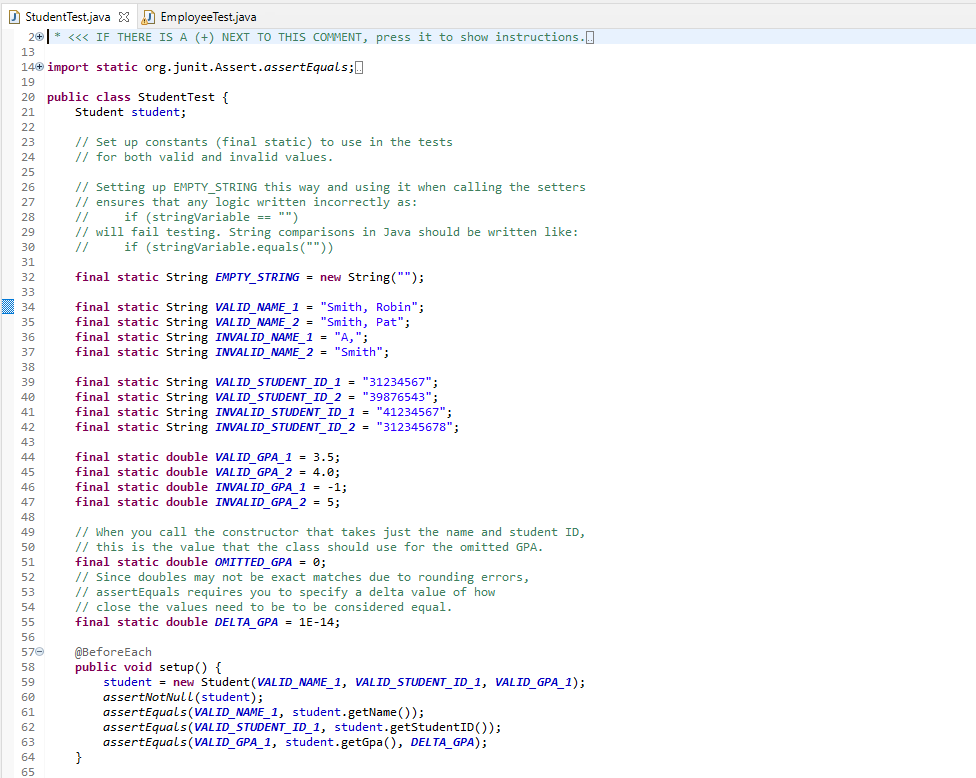
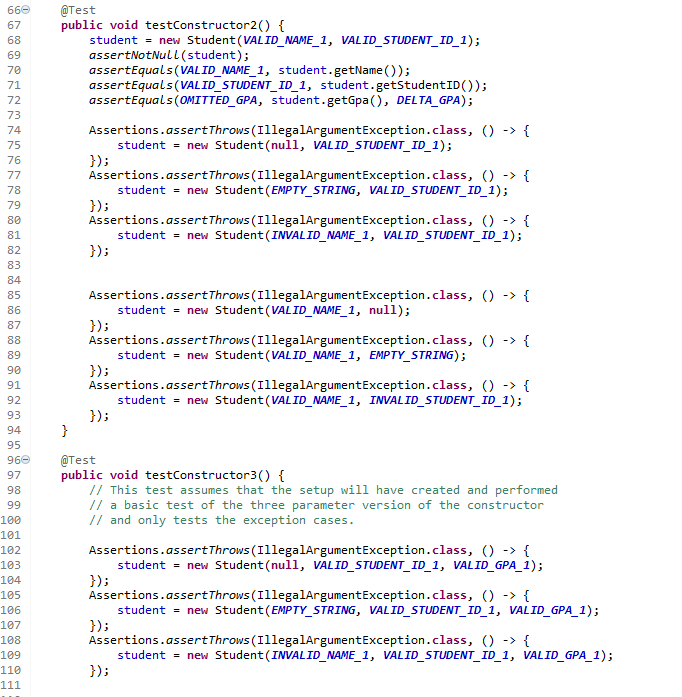
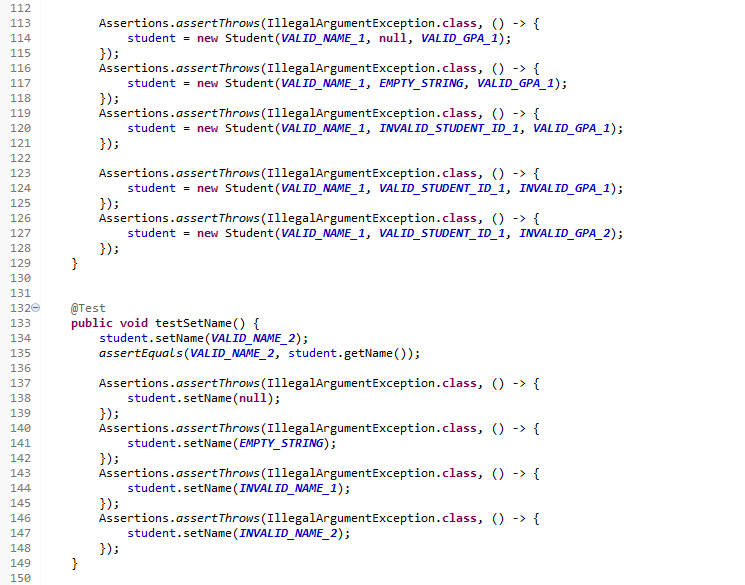
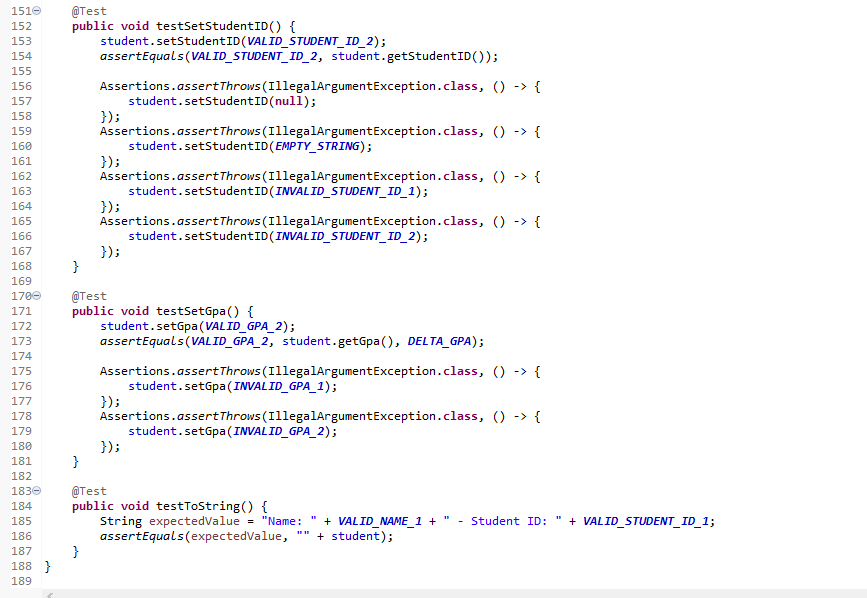
Employee Class from PersonV1
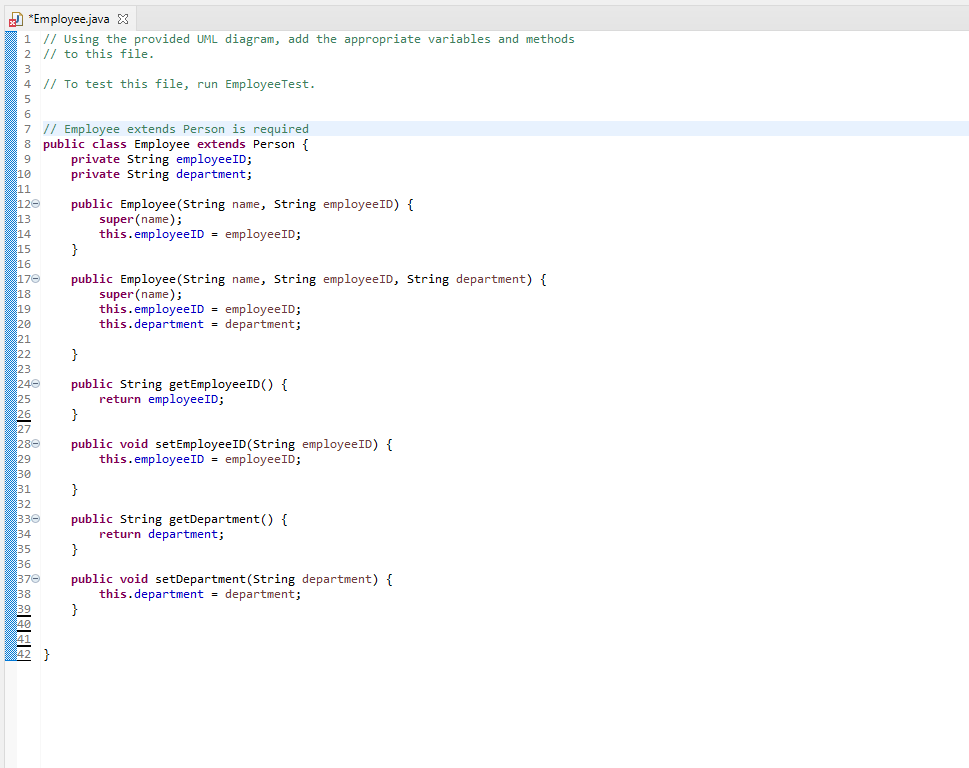
To get started, follow these steps. 1. In Eclipse, import the PersonV2 project. Refer to Import Project in Eclipse if needed for how to use pull and import. 2. Expand PersonV2, src, default package in the Eclipse Package Explorer. 3. Open up StudentTest and expand the comments at the top of the top. Follow the steps in the comments to add the JUnit 5 library to your build path. 4. Verify that you can run StudentTest successfully. 5. Open Student and review how the constructors only makes calls to super, this, or setters. 6. Review the setters in Student and review how they throw IllegalArgumentExceptions for any attempt to provide invalid data. 7. Open StudentTest to review how the various tests are implemented. 8. In PersonV1, open up your original Employee class, select all and copy the text, then close Employee. 9. In Personv2, open up the new Employee class and replace everything with what you copied in the previous step. 10. If necessary, set up your constructors so they only make calls to super, this, or setters. Do not directly assign any values to any variables. 11. Modify setEmployeeld and add checks to prevent the user from setting the value to null. Require the value to start with the letter E and be exactly 9 characters long. 12. Modify setDepartment and add checks to prevent the user from setting the value to null or "". 13. Open up EmployeeTest. Using StudentTest as a guide, create the necessary constants and methods to test your Employee class. 14. In Package Explorer, right-click on PersonV2 and choose Team > Commit... 15. In the Stages Changes folder, you should see Employee.java and EmployeeTest.java. 16. Enter a brief message in the Commit Message box. 17. If necessary, enter your email in the Author and Committer boxes. 18. Press Commit and Push... 19. You should see a dialog box indicating the commit was successful. If you run into problems at this step, contact your instructor 20. Access the csem.cc option in the navigation menu, log in, and run the PersonV2 test. If necessary, make additional changes, commit those changes, and run the test again. * setup Student Test.java Employee Test.java X 10/ * 2 * Add Libraries... 20 2. In the list of libraries, choose JUnit then press Next. 21 3. For the library version, choose JUnit 5 then press Finish. 22 */ 23 24e fimport static org. junit. Assert.assertEquals; 25 import static org. junit. Assert.assertNotNull; 0:26 import org. junit.jupiter.api. Assertions; 27 import org. junit.jupiter.api.BeforeEach; 28 import org.junit.jupiter.api.Test; 29 30 public class Employee Test { 31 Employee employee; 32 33 34 35} * StudentTest.java X Employee Test.java 24|* { student = new Student (null, VALID_STUDENT_ID_1); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (EMPTY_STRING, VALID_STUDENT_ID_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (INVALID_NAME_1, VALID_STUDENT_ID_1); }); 76 }); 77 78 79 80 81 82 83 84 85 86 87 88 89 Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, null); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, EMPTY_STRING); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, INVALID_STUDENT_ID_1); }); 90 91 92 93 94 95 } 960 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 @Test public void testConstructor3() { // This test assumes that the setup will have created and performed // a basic test of the three parameter version of the constructor // and only tests the exception cases. Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (null, VALID_STUDENT_ID_1, VALID_GPA_1); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (EMPTY_STRING, VALID_STUDENT_ID_1, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (INVALID_NAME_1, VALID_STUDENT_ID_1, VALID_GPA_1); }); }); Assertions.assertThrows (IllegalArgumentException.class, ) -> { student = new Student (VALID_NAME_1, null, VALID_GPA_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, EMPTY_STRING, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, INVALID_STUDENT_ID_1, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, VALID_STUDENT_ID_1, INVALID_GPA_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, VALID_STUDENT_ID_1, INVALID_GPA_2); }); } 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 @Test public void testSetName() { student.setName(VALID_NAME_2); assertEquals(VALID_NAME_2, student.getName(); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setName(null); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setName(EMPTY_STRING); }); Assertions.assert Throws (IllegalArgumentException.class, () -> { student.setName (INVALID_NAME_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setName (INVALID_NAME_2); }); } @Test public void testSetStudentID) { student.setStudentID(VALID_STUDENT_ID_2); assertEquals(VALID_STUDENT_ID_2, student.getStudentIDO); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setStudentID(null); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setStudentID(EMPTY_STRING); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setStudentID(INVALID_STUDENT_ID_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setStudentID(INVALID_STUDENT_ID_2); }); } 1510 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 1700 171 172 173 174 175 176 177 178 179 180 181 182 1830 184 185 186 187 188 } 189 @Test public void testSetGpa() { student.setGpa (VALID_GPA_2); assertEquals(VALID_GPA_2, student.getGpa(), DELTA_GPA); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setGpa(INVALID_GPA_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setGpa(INVALID_GPA_2); }); } @Test public void testToString() { String expectedValue = "Name: assertEquals(expectedValue, } Student ID: + VALID_NAME_1 + + student); + VALID_STUDENT_ID_1; *Employee.java X 1 // Using the provided UML diagram, add the appropriate variables and methods 2 // to this file. 3 4 // To test this file, run EmployeeTest. 5 6 7 // Employee extends Person is required 8 public class Employee extends Person { 9 private String employeeID; 10 private String department; 11 120 public Employee(String name, String employeeID) { 13 super (name); 14 this.employeeID = employeeID; 15 } 16 170 public Employee(String name, String employeeID, String department) { 18 super(name); this.employeeID = employeeID; this.department = department; } 23 240 25 public String getEmployeeID) { return employeeID; public void setEmployee ID(String employeeID) { this. employeeID = employeeID; } 280 29 30 31 32 330 34 35 36 370 38 public String getDepartment() { return department; } public void setDepartment(String department) { this.department = department; } }