Question
need help using a pointer based linked list to hold the information, rather than an array of records. Write a C++ object-oriented program First Name
need help using a pointer based linked list to hold the information, rather than an array of records.
Write a C++ object-oriented program
First Name string
Last Name string
Student ID unsigned integer
Email string
GPA - float
The program will manipulate the student list based on students from the file students.dat
Class requirements
Create a student class or struct based on the student structure
Create a students class which contains two private members a pointer to a list of students and a function to print a students record. The students class will also provide the following functionality.
Load data from a data file into a student list
Retrieve and print a student from the student list
Insert a student into the student list
Delete a student from the student list
Print the contents of a student record
Print the contents for a list of students
Sort the student list by last Name
Sort the student list by GPA
Processing requirements
Load the students file students.dat
Create a menu to carry out the given operations
Implement input validation to avoid erroneous program errors
Structure of the file
students.dat First Name, Last Name, Student ID, Email, GPA
If you had a working program 1, then there is no need to change your main function. If you do not have a satisfactory working program, I can provide you with one.
Smith Jefferey 891789 j.smith@spartans.nsu.edu 3.75 Lee Jackson 3678902 j.lee@spartans.nsu.edu 3.66 Gonzales Mariana, 168790 m.gonzales18@spartans.nsu.edu 4.0 Jones Mike 8973125 m.jones143@spartans.nsu.edu 3.1 Williams Anita 2985465 a.williams@spartans.nsu.edu 3.64 Ronsinson Taylor 3278976 t.robinson@spartans.nsu.edu 3.55 Clark Allen 1094567 a.clark@spartans.nsu.edu 3.48 Turner Tavon 318796 t.turner@spartans.nsu.edu 3.2 Jenkins Nelson 289563 n.jenkins@spartans.nsu.edu 3.0
need help using a pointer based linked list to hold the information, rather than an array of records. This is what I have so far.
#include
using namespace std;
struct studentInfo;
typedef studentInfo * studentptr, * head, * tail;
struct studentInfo { string firstName; string lastName; unsigned int studentID; string email; float gpa; studentptr next; };
class studentInfoProc // Change this. And just a note, wherever you see studentsProc elsewhere in my code, change it to whatever you change it to... { public: studentInfoProc(); void loadList(); void printList(); void retrieveStudent(); void insertStudent(); void deleteStudent(); void printStudent(); void sortLastName(); void sortGPA(); private: // Took the private members out, read the instructions and you'll know what to put here studentInfo students[40]; int size; void printList() const; };
studentInfoProc::studentInfoProc() { studentInfo = 0; }
void studentInfoProc::loadList() { ifstream infile; infile.open("students.dat"); studentInfo = NULL; studentInfo temp; studentptr temp_ptr; while (!infile.eof()) { temp_ptr = new student; infile >> temp_ptr->lastName >> temp_ptr->firstName >> temp_ptr->studentID >> temp_ptr->email >> temp_ptr->gpa;//place data in the node temp_ptr->next = students; studentsInfo = temp_ptr; } infile.close(); }
void studentInfoProc::retrieveStudent() { string lastName; cout << endl; print(); cout << endl; cout << "Enter the students information " << endl; cin >> lastName; cout << endl << "here is the information you need: " << endl; for (studentptr s = students; s != NULL; s = s->next) if(lastName==s->lastName) { cout << "students information: " << s->lastName << ", " << s->firstName << endl << endl; } }
void studentInfoProc::insertStudent() { studentptr temp = new student; cout << endl; cout << "enter the following info: " << endl; cout << "last name: "; cin >> temp->lastName; cout << "first name: "; cin >> temp->firstName; cout << "stu id: "; cin >> temp->studentID; cout << "email: "; cin >> temp->email; cout << "gpa: "; cin >> temp->gpa; cout << endl; temp->next = students; students = temp; cout << "student list: " << endl; printList(); }
void studentInfoProc::deleteStudent() { cout << endl; studentptr prev, pos; string lastName; bool done = false; pos = NULL; prev = NULL; print(); cout << endl; cout << "enter the student's last name: " << endl; cin >> lastName; cout << endl; for (studentptr s = students; (s != NULL) && (done == false); s = s->next) { if (s->lastName == lastName) { done = true; pos = s; } else { prev = s; } } if(prev != NULL) { prev->next = pos->next; } else { students = students->next; } cout << "student list " << endl; printList(); }
void studentInfoProc::printList() { cout << endl; for (studentptr s = students; s != NULL; s = s->next) cout << "first name: " << s->firstName << " * " << "last name: " << s->lastName << " * " << "student id: " << s->studentID << " * " << "e-mail: " << s->email << " * " << "gpa: " << s->gpa << endl; }
void studentInfoProc::sortLastName() { }
void studentInfoProc::sortGPA() { }
void studentInfoProc::printStudent() { string lastName; cout << endl; print(); cout << endl; cout << "enter info: " << endl; cin >> lastName; cout << endl << "here is the info: " << endl; for (studentptr s = students; s != NULL; s = s->next) if(lastName==s->lastName) { cout << "last name: " << s->lastName << " * " << "first name: " << s->firstName << " * " << "student id: " << s->studentID << " * " << "email: " << s->email << " * " << "gpa: " << s->gpa << endl; } cout << endl; } int main() { studentInfo stu; //Decalre object for a process int size; ifstream infile; int list = -1; //Deterimines which process list char answer; int selection;
cout << " " << endl; stu.loadData();
do {
switch (selection) { case 1: studentInfo.printList(); break; case 2: studentInfo.insertStudent(); break; case 3: studentInfo.deleteStudent(); break; case 4: studentInfo.retrieveStudent(); break; case 5: studentInfo.sortByLastName(); break; case 6: studentInfo.sortByGPA(); break; case 0: exit(0); break; default:cout << "Invalid. Please choose an option 1-6!" << endl; } } while (selection != 0);
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
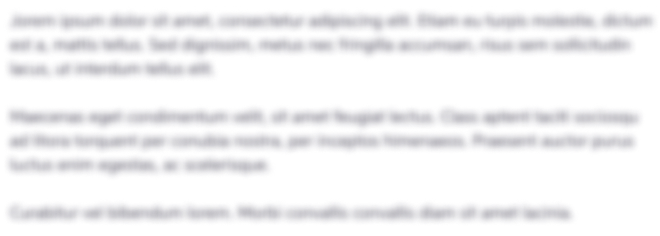
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started