Question
Need help with C++. Please answer this question as soon as possible! Objectives: Understanding the nuances of working with pointers in classes. Working with abstract
Need help with C++. Please answer this question as soon as possible!
Objectives:
- Understanding the nuances of working with pointers in classes.
- Working with abstract classes.
- Working with virtual functions to achieve run-time polymorphism.
Preliminary Notes:
- Download the files Instrument.h, Instrument.cpp, Tuba.h, Tuba.cpp, Harp.h, Harp.cpp and Client.cpp from Canvas. Use these files to create a project in Visual Studio.
- Imagine youre writing a simulator client that involves playing Harps and Tubas. Tuba and Harp are both Instruments and inherit from the Instrument base class.
- Notice that Instrument is abstract. In our client, we dont expect to instantiate a Instrument that isnt a specific type of Instrument.
- The Instrument base class provides general attributes that are common among all Instruments. In our program, all Instruments have a brand and sound.
- The base class also provides instructions for things that must be implemented in derived classes. In our program, all Instruments must support a play() function, which prints out the instruments information and makes a sound. However, every type of Instrument has different types of information, so those operations are required to be implemented in derived classes.
- To make the program more spontaneous, the client interacts with different types of Instruments in a randomized order. The order in which each Instrument is generated is determined at run-time. At compile-time, the order in which the Instruments will be generated is unknown.
Part One:
Implement the Instrument base class, Tuba derived class, and Harp derived class according to the following TODO instructions:
Part Two:
Modify the provided client.cpp file to demonstrate the use of run-time polymorphism by following these TODO instructions:
Below are the given codes:
Client.cpp
#include
using namespace std;
const int LENGTH = 12;
int main() { srand(static_cast
// TODO: Declare an array of 12 pointers to base class Instrument objects. // (4 points).
// TODO: Loop through the array of base class pointers. Use the pointer // at each index to point to a new derived class object. Randomize // whether derived class objects will be a Tuba or a Harp. // // HINT: Consider this pseudo-code for inside the for-loop... // if some random number divided by two has a remainder of zero // the pointer at this index points to a new Tuba object. // else // the pointer at this index points to a new Harp object. // (30 points).
// TODO: Loop through the array, and call play on each object. // Since the order of Instrument objects is randomized at run-time, // how do we know whether we're playing a Tuba or a Harp? // Do we have to know which? Why or why not? // (10 points).
// TODO: Loop through the array once more to delete dynamically // allocated memory. // (5 points).
return 0; }
Harp.cpp
#include "Harp.h"
Harp::Harp() { // TODO: Implement the Harp derived class default constructor. Initialize the // the numberOfStrings member variable to 0. // (3 points). }
Harp::Harp(string instrumentBrand, string instrumentSound, int numStrings) :Instrument(instrumentBrand, instrumentSound) { // TODO: Implement the Harp derived class parameterized constructor. Initialize the // member variables requiring initialization according to the values passed in the // parameters. // (3 points). }
Harp::~Harp() {}
void Harp::setNumberOfStrings(int numStrings) { // TODO: Implement the setNumberOfStrings function. Set the numberOfStrings member variable // according to the value passed in the parameter. // (3 points). }
int Harp::getNumberOfStrings() const { // TODO: Implement the getNumberOfStrings function. Return the numberOfStrings member // variable. // (3 points). }
void Harp::play() const { // TODO: Implement the play function. Print the Instrument name, its sound, // and its number of strings. // ex. "Playing the Pixie brand harp, which has 40 strings, makes the sound: 'Pling-pluck' " // (3 points). } Harp.h
#pragma once #include
using namespace std;
Instrument.cpp
#include "Instrument.h"
Instrument::Instrument() { // TODO: Implement the base class default constructor. Initialize the // brand and sound to unknown. // (3 points). }
Instrument::Instrument(string instrumentBrand, string instrumentSound) { // TODO: Implement the base class default constructor. Initialize the // vechicleName and topSpeed to the values passed in the parameters. // (3 points). }
Instrument::~Instrument() { // TODO: When a class has virtual functions, a virtual destructor must be // included. Does any code need to be written it this specific virtual // destructor? Why or why not? // (3 points). }
void Instrument::setBrand(string instrumentBrand) { // TODO: Implement the setBrandfunction. Set the brand member // variable according to the value passed in the parameter. // (3 points). }
void Instrument::setSound(string instrumentSound) { // TODO: Implement the setSound function. Set the sound member // variable according to the value passed in the parameter. // (3 points). }
string Instrument::getBrand() const { // TODO: Implement the getBrand function. Return the // brand member variable. // (3 points). }
string Instrument::getSound() const { // TODO: Implement the getSound function. Return the // sound member variable. // (3 points). }
class Harp : public Instrument { public: Harp(); Harp(string, string, int); virtual ~Harp(); void setNumberOfStrings(int); int getNumberOfStrings() const; virtual void play() const;
private: int numberOfStrings; };
Instrument.h
#pragma once #include
using namespace std;
class Instrument { public: Instrument(); Instrument(string, string); virtual ~Instrument(); void setBrand(string); void setSound(string); string getBrand() const; string getSound() const; virtual void play() const = 0;
private: string brand; string sound; };
Tuba.cpp
#include "Tuba.h"
Tuba::Tuba() { // TODO: Implement the Tuba derived class default constructor. Initialize the // the isSousaphone member variable to false. // (3 points). }
Tuba::Tuba(string instrumentBrand, string instrumentSound, bool isSous) :Instrument(instrumentBrand, instrumentSound) { // TODO: Implement the Tuba derived class parameterized constructor. Initialize the // member variables requiring initialization according to the values passed in the // parameters. // (3 points). }
Tuba::~Tuba() {}
void Tuba::setIsSousaphone(bool isSous) { // TODO: Implement the setIsSousaphone function. Set the isSousaphone member variable // according to the value passed in the parameter. // (3 points).
}
bool Tuba::getIsSousaphone() const { // TODO: Implement the getIsSousaphone function. Return the IsSousaphone member // variable. // (3 points). }
void Tuba::play() const { // TODO: Implement the play function. Print the Instrument name, its sound, // and if it is a sousaphone. // ex. "Playing the Yamaha brand tuba, which is not a sousaphone, makes the sound: 'Oom-pah' " // (3 points). } Tuba.h
#pragma once #include
using namespace std;
class Tuba : public Instrument { public: Tuba(); Tuba(string, string, bool); virtual ~Tuba(); void setIsSousaphone(bool); bool getIsSousaphone() const; virtual void play() const;
private: bool isSousaphone; };
Step by Step Solution
There are 3 Steps involved in it
Step: 1
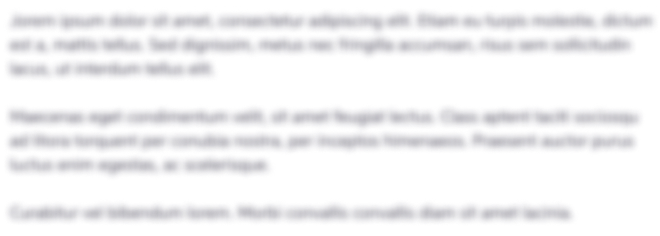
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started