Question
need help with creating the alchemist cpp class the alchemist.h and ele You must use the provided header files ( Element.h, Alchemist.h and Game.h ).
need help with creating the alchemist cpp class the alchemist.h and ele
- You must use the provided header files (Element.h, Alchemist.h and Game.h). You may only add HELPER functions and global constants to these files. Do not add member variables to any class.
- All user input must be validated. For example, if a menu allows for 1, 2, or 3 to be entered and the user enters a 4, it will re-prompt the user. However, the user is expected to always enter the correct data type. i.e. If the user is asked to enter an integer, they will. If they are asked to enter a character, they will. You do not need to worry about checking for correct data types.
- There is a single input files for this project named, proj2_data.txt. The file name can be stored as a constant. The file is already provided in Prof. Dixons course folder on GL.
- For the basic version of this project, pointers are not required, however, pass-by-reference is required. For the extra credit version, you must use pointers for the Alchemists array of elements.
- The players name can have a space (use getline). Additionally, the names of the elements may have a space.
- Have a main menu that asks if the user wants to:
- Display currently known elements in a numbered list.
- Combine Elements
- Asks which elements you would like to combine Validates input for elements chosen. Keeps track of number of attempts (total attempts) and number of repeats (a repeat is any result that yields an element that is already known).
- A recipe for an element is made up of two elements in any order. For example, Fire is a starting element and would be missing the first and second elements. Dust would be made from combining Earth and Air.
- An Alchemist would have to know an element in order to use to in a combination. For example, in order to try and make an Airplane, the Alchemist would have already had to have successfully learned Metal and Bird.
- Display Score
- Score is made up of total attempts, number of repeats, total elements found (starts at 4), and percentage found.
- Exit
- Recommendations
You must use the provided header files (Element.h, Alchemist.h, and Game.h) additionally, we provided you with the makefile and the proj2.cpp.
Here are some general implementation recommendations (do not follow these verbatim these are GENERAL suggestions):
-
- Read each of the header files in detail. Use paper to take notes. Each header file has tons of comments.
- Design the solution (part is already designed, so make sure you understand how it works) on PAPER.
- Start with the Alchemist.cpp file and code everything. Dont forget the constructor! Test everything incrementally.
- Start Game.cpp start building the constructor. Incrementally build it as you are testing a function.
- Once Alchemist.cpp is written, start on Game.cpp start with loading in the elements from the proj2_data.txt file. This will be tricky because you have element names with spaces and a comma delimited data file.
- CombineElements() is one of the most difficult functions to write so expect to spend some time finishing it.
- After the files are successfully loaded (and displayed) work on the main menu.
-
//Title: Element.h //Author: Jeremy Dixon //Date: 2/22/2018 //Description: This is part of the Alchemist Project in CMSC 202 @ UMBC
#ifndef ELEMENT_H //Include/Header Guard #define ELEMENT_H //Include/Header Guard
#include #include #include
using namespace std;
// Name: Element struct // Desc - Used to store data about elements struct Element { string m_name; //Name of the element string m_element1; //Required element 1 to create string m_element2; //Required element 2 to create Element() {} //Default constructor Element(string name, string element1, string element2) { m_name = name; //Name of this element m_element1 = element1; //Name of first required element to create m_element2 = element2; //Name of second required element to create } };
#endif
-
//Title: Alchemist.h //Author: Jeremy Dixon //Date: 2/22/2019 //Description: This is part of the Alchemist Project in CMSC 202 @ UMBC // This is the pointer version header files
#ifndef ALCHEMIST_H //Include/Header Guard #define ALCHEMIST_H //Include/Header Guard
#include "Element.h" //Include for the element struct #include #include #include #include using namespace std;
//Constants (allowed to add more as needed) const int PROJ2_SIZE = 390;
// class - allowed to add HELPER functions only class Alchemist { public: // Name: Alchemist() - Default Constructor // Desc: Used to build a new Alchemist // Preconditions - Requires default values // Postconditions - Creates a new Alchemist Alchemist(); // Name: Alchemist(name) - Overloaded constructor // Desc - Used to build a new Alchemist // Preconditions - Requires name // Postconditions - Creates a new Alchemist Alchemist(string name); // Name: GetName() // Desc - Getter for Alchemist name // Preconditions - Alchemist exists // Postconditions - Returns the name of the Alchemist string GetName(); // Name: SetName(string) // Desc - Allows the user to change the name of the Alchemist // Preconditions - Alchemist exists // Postconditions - Sets name of Alchemist void SetName(string name); // Name: DisplayElements() // Desc - Displays a numbered list of all elements known by the Alchemist // Preconditions - Alchemist exist // Postconditions - None void DisplayElements(); // Name: GetNumElements() // Desc - Returns number of elements known by the Alchemist // Preconditions - Alchemist exists // Postconditions - None int GetNumElements(); // Name: CheckElement(Element) // Desc - Checks to see if the Alchemist has an element // Preconditions - Alchemist already has elements // Postconditions - Returns true if Alchemist has element else false bool CheckElement(Element); // Name: AddElement(Element) // Desc - Adds element to m_myElements if not known increases numElements // Preconditions - Alchemist exists and new element not already known // Postconditions - Adds element to m_myElements void AddElement(Element); // Name: GetElement(int) // Desc - Checks to see if the alchemist has an element // Preconditions - Alchemist already has elements // Postconditions - Returns element at index Element GetElement(int); // Name: AddAttempt // Desc - Increments attempts // Preconditions - Alchemist attempts a merge // Postconditions - Increments every time a merge is attempted void AddAttempt(); // Name: GetAttempts() // Desc - Returns number of attempts for that Alchemist // Preconditions - Alchemist exists // Postconditions - Returns total number of attempts int GetAttempts(); // Name: AddRepeat() // Desc - Increments repeats for every time an element has already been found // Preconditions - Alchemist attempts a merge // Postconditions - Increments every time a merge yields an element already found void AddRepeat(); // Name: GetRepeats() // Desc - Returns number of repeats for that Alchemist // Preconditions - Alchemist exists // Postconditions - Returns total number of repeats int GetRepeats(); private: string m_myName; //Name of Alchemist int m_attempts; //Total Combination Attempts int m_repeats; //Combination Attempts Repeated int m_numElements; //Number of elements known to the Alchemist Element m_myElements[PROJ2_SIZE] = {}; //List of elements known to the Alchemist };
#endif //Exit Header Guard
-
//Title: Game.h //Author: Jeremy Dixon //Date: 2/22/2019 //Description: This is part of the Alchemist Project in CMSC 202 @ UMBC
#ifndef GAME_H //Header Guard #define GAME_H //Header Guard #include
#include "Element.h" #include "Alchemist.h" //Constants (additional constants allowed) const string PROJ2_DATA = "proj2_data.txt"; //File constant
//Additional HELPER functions allowed to be added as needed
class Game { public: /* Name: Game() Default Constructor // Desc - Builds a new game by: // 1. Loads all Alchemy recipes into m_elements (by making elements) // 2. Asks user for their alchemist's name (store in m_myAlchemist as m_myName) // 3. Adds fire, water, air, and earth to m_myElements (in m_myAlchemist) // Preconditions - None // Postconditions - m_elements is populated */ Game(); //Default Constructor // Name: LoadElements // Desc - Loads each element into m_elements from file // Preconditions - Requires file with valid element data // Postconditions - m_element is populated with element structs void LoadElements(); // Name: StartGame() // Desc - Manages the game itself including win conditions continually // calling the main menu // Preconditions - Player is placed in game // Postconditions - Continually checks to see if player has entered 5 void StartGame(); // Name: DisplayMyElements() // Desc - Displays the current elements // Preconditions - Player has elements // Postconditions - Displays a numbered list of elements void DisplayElements(); // Name: MainMenu() // Desc - Displays and manages menu // Preconditions - Player has an Alchemist // Postconditions - Returns number including exit int MainMenu(); // Name: CombineElements() // Desc - Attempts to combine known elements // Preconditions - Alchemist is populated with elements // Postconditions - May add element to Alchemist's list of elements void CombineElements(); // Name: RequestElement() // Desc - Requests element to use to merge // Preconditions - Alchemist has elements to try and merge // Postconditions - Returns void RequestElement(int &choice); // Name: SearchRecipes() // Desc - Searches recipes for two strings // Preconditions - m_elements is populated // Postconditions - Returns int index of matching recipe int SearchRecipes(string, string); // Name: CalcScore() // Desc - Displays current score for Alchemist // Preconditions - Alchemist is populated with elements // Postconditions - None void CalcScore(); // Name: GameTitle() // Desc - Title for Game (Do not edit) // Preconditions - None // Postconditions - None void GameTitle() { cout << "**************************************************************" << endl; cout << " AAA L CCCCC H H EEEEE M M I SSSSS TTTTT " << endl; cout << " A A L C H H E MM MM I S T " << endl; cout << " AAAAA L C HHHHH EEEEE M M M I SSSSS T " << endl; cout << " A A L C H H E M M I S T " << endl; cout << " A A LLLLL CCCCC H H EEEEE M M I SSSSS T " << endl; cout << "**************************************************************" << endl; } private: Alchemist m_myAlchemist; //Player's Alchemist for the game Element m_elements[PROJ2_SIZE]; //All elements in the game (loaded from file) };
#endif //Exit Header Guard
Step by Step Solution
There are 3 Steps involved in it
Step: 1
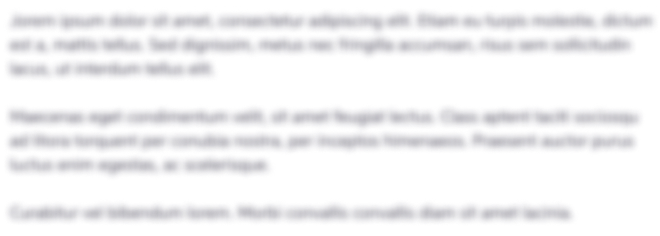
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started