Question
Need help with Game constructor method. Looking for, input: none output: none 1) create the two players and a pigDice 2) inintializes the current player
Need help with Game constructor method.
Looking for,
input: none
output: none
1) create the two players and a pigDice
2) inintializes the current player to start the game and sets game over condition to false.
This is what I need help with, I have attached the code for Game, Player, PairOfDice, and Die Classes in case you need to see what I have done so far, the Die and PairOfDice classes have been checked off so they are set and just there for reference if needed.
Thankyou,
import java.util.Scanner;
//******************************************************************************************
//
//
//******************************************************************************************
public class Game
{
//---------------------------------------------------------------------------------------
//
//---------------------------------------------------------------------------------------
private Player p1, p2, current;
private PigDice d;
private boolean gameOver;
private final int WINNING_SCORE = 100;
//---------------------------------------------------------------------------------------
// Game Constructor method
//---------------------------------------------------------------------------------------
public Game()
{
Scanner scan = new Scanner(System.in);
}
//---------------------------------------------------------------------------------------
// need details of PigDice to play game
//---------------------------------------------------------------------------------------
public boolean playGame()
{
}
//---------------------------------------------------------------------------------------
//
//---------------------------------------------------------------------------------------
public boolean gameOver()
{
return gameOver;
}
//---------------------------------------------------------------------------------------
//
//---------------------------------------------------------------------------------------
public Player getCurrentPlayer()
{
return p1;
}
//---------------------------------------------------------------------------------------
//
//---------------------------------------------------------------------------------------
public Player getHuman()
{
return p2;
}
//---------------------------------------------------------------------------------------
//
//---------------------------------------------------------------------------------------
public Player getComputer()
{
return getComputer;
}
//--------------------------------------------------------------------------------------
//
//--------------------------------------------------------------------------------------
public PigDice getPigDice()
{
return d;
}
//--------------------------------------------------------------------------------------
//
//--------------------------------------------------------------------------------------
public String toString()
{
return "Game{" +
"p1=" + p1 +
",p2=" + p2 +
",current=" + current +
", d=" + d +
",gameOver=" + gameOver +
",WINNER_SCORE=" + WINNER_SCORE + "}";
}
}
****************************************************************************************************************
Player Class code, is not finished
****************************************************************************************************************
//*************************************************************************************
// Player.java Author: 3-31-18
//
//*************************************************************************************
public abstract class Player
{
//---------------------------------------------------------------------------------
// declaring protected variables
//---------------------------------------------------------------------------------
protected String name;
protected int roundPoints, gamePoints;
protected boolean pigness;
//---------------------------------------------------------------------------------
// constructor for name
//---------------------------------------------------------------------------------
public Player(String name)
{
this.name = name;
}
//---------------------------------------------------------------------------------
// getter name
//---------------------------------------------------------------------------------
public String getName()
{
return name;
}
//---------------------------------------------------------------------------------
// getter RoundPoints
//---------------------------------------------------------------------------------
public int getRoundPoints()
{
return roundPoints;
}
//---------------------------------------------------------------------------------
// getter GamePoints
//---------------------------------------------------------------------------------
public int getGamePoints()
{
return gamePoints;
}
//---------------------------------------------------------------------------------
// getter boolean Pigness
//---------------------------------------------------------------------------------
public boolean getPigness()
{
return pigness;
}
//---------------------------------------------------------------------------------
//
//---------------------------------------------------------------------------------
public void takeTurn(PigDice p)
{
}
//---------------------------------------------------------------------------------
//
//---------------------------------------------------------------------------------
public abstract boolean beAPig(boolean isPig);
@Override
public String toString()
{
return "Player{" +
"name = " + name +
", roundPoints =" + roundPoints +
", gamePoints =" + gamePoints +
", pigness =" + pigness + "}";
*****************************************************************************************************************************************************************
PairOfDice code is finished
*************************************************************************************************************************************************************
import javafx.scene.image.Image;
//********************************************************************
// PairOfDice.java Author: 3/14/2018
//
// Represents a Pair of Dice that aggregates two Die objects
//********************************************************************
public class PairOfDice
{
//Class level variables
private Die die1, die2;
//-----------------------------------------------------------------
// Constructor: Instantiates the two Die objects and stores them
// as class level variables
//-----------------------------------------------------------------
public PairOfDice()
{
die1 = new Die();
die2 = new Die();
}
//-----------------------------------------------------------------
// die 1 value accessor (getter)
//-----------------------------------------------------------------
public int getDie1()
{
return die1.getFaceValue();
}
//-----------------------------------------------------------------
// die 2 value accessor (getter)
//-----------------------------------------------------------------
public int getDie2()
{
return die2.getFaceValue();
}
//-----------------------------------------------------------------
// die 1 value mutator (setter)
//-----------------------------------------------------------------
public void setDie1(int val)
{
die1.setFaceValue(val);
}
//-----------------------------------------------------------------
// die 2 value mutator (setter)
//-----------------------------------------------------------------
public void setDie2(int val)
{
die2.setFaceValue(val);
}
//-----------------------------------------------------------------
// Rolls both of the die objects and returns the sum of the roll
//-----------------------------------------------------------------
public int roll()
{
return die1.roll() + die2.roll();
}
//-----------------------------------------------------------------
// Returns a string representation of this PairOfDice object with
// the values labeled appropriately
// Overrides the toString method inherited from Object
//-----------------------------------------------------------------
public String toString()
{
return "Die 1: " + die1.toString()
+ " Die 2: " + die2.toString()
+ " \tSum: " + (die1.getFaceValue() + die2.getFaceValue());
}
//-----------------------------------------------------------------
// Image die1 (getter), from Die program aggregation
// PigDice program will use inheritance from PairOfDice program
//-----------------------------------------------------------------
public Image getDie1Image()
{
return die1.getDieImage();
}
//-----------------------------------------------------------------
// Image die2 (getter), from Die program aggregation
// PigDice program will use inheritance from PairOfDice program
//-----------------------------------------------------------------
public Image getDie2Image()
{
return die2.getDieImage();
}
***********************************************************************************************************************************
Die class code is finished
**********************************************************************************************************************************
import javafx.scene.image.Image;
//********************************************************************
// Die.java 3/13/2018
//
// Represents one die (singular of dice) with faces showing values
// between 1 and 6.
//********************************************************************
public class Die
{
private final int MAX = 6; // maximum face value
private int faceValue; // current value showing on the die
//-----------------------------------------------------------------
// Constructor: Sets the initial face value.
//-----------------------------------------------------------------
public Die()
{
faceValue = 1;
}
//-----------------------------------------------------------------
// Rolls the die and returns the result.
//-----------------------------------------------------------------
public int roll()
{
faceValue = (int)(Math.random() * MAX) + 1;
return faceValue;
}
//-----------------------------------------------------------------
// Face value mutator.
//-----------------------------------------------------------------
public void setFaceValue (int value)
{
faceValue = value;
}
//-----------------------------------------------------------------
// Face value accessor.
//-----------------------------------------------------------------
public int getFaceValue()
{
return faceValue;
}
//-----------------------------------------------------------------
// Returns a string representation of this die.
//-----------------------------------------------------------------
public String toString()
{
String result = Integer.toString(faceValue);
return result;
}
//----------------------------------------------------------------
// getter of 6 die images from file
// PairOfDice program, aggregation to get images form Die Program
//----------------------------------------------------------------
public Image getDieImage()
{
Image pic = null;
switch (faceValue)
{
case 1:
pic = new Image("die1.png");
break;
case 2:
pic = new Image("die2.png");
break;
case 3:
pic = new Image("die3.png");
break;
case 4:
pic = new Image("die4.png");
break;
case 5:
pic = new Image("die5.png");
break;
case 6:
pic = new Image("die6.png");
break;
}
return pic ;
}
}
*********************************************************************************************************************
PigDice Code
***************************************************************************************************************
//*******************************************************************************
// PigDice.java Author: 3-14-18
//
//*******************************************************************************
public class PigDice extends PairOfDice
{
//---------------------------------------------------------------------------
// Constructor PigDice
//---------------------------------------------------------------------------
public PigDice()
{
super();
}
//--------------------------------------------------------------------------
// Inheritance from PairOfDice
// Adjust the roll to output 3 everytime a 1 is rolled with any
// other number except one. Snake eyes produces sum: 2
//--------------------------------------------------------------------------
public int roll()
{
int sum = super.roll();
if (((getDie1()==1) && (getDie2()!=1)) || ((getDie2()==1) && (getDie1()!=1)))
sum = 3;
return sum;
}
//----------------------------------------------------------------------------
//
//
//----------------------------------------------------------------------------
public String toString()
{
int sum = getDie1() + getDie2();
if (((getDie1()==1) && (getDie2()!=1)) || ((getDie2()==1) && (getDie1()!=1)))
sum = 3;
return "\tDie 1: " + getDie1()
+ " \tDie 2: " + getDie2()
+ " \tSum: " + sum;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
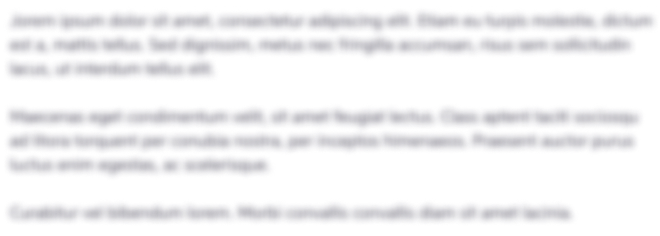
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started