Question
Need help with Intro to Java Programming problem: For this assignment you will write a class MMAccount which is a subclass of the Account class.
Need help with Intro to Java Programming problem:
For this assignment you will write a class MMAccount which is a subclass of the Account class. It represents a money market account, which is a type of savings account that also allows limited use of checks and other withdrawals. I have provided a template for the class found in the MMAccount.java file posted to D2L. You should begin your assignment by downloading that template. Note that I have also provided an Account class and a test program called TestMMAccount. You will need all three files to complete this assignment, although you will only submit the MMAccount.java file.
The MMAccount has three private instance variables minBal, monthlyFee, and interestRate, representing the minimum balance, monthly fee, and interest rate associated with the account. It also has two constants: MIN_A which represents the minimum account balance to avoid fees and earn interest and is set to 1000.0 and MIN_VAL which represents the minimum interest rate, mimimum monthly fee, and the amount below which the account account drop and is set to 0.0.
You will complete the assignment by writing the following methods for the MMAccount class:
public MMAccount(String inName, String number, double amount, double minimum, double fee, double rate): The constructor for the class sets the name, account number and balance on the account using the first three parameters and a call to the Account constructor. These variables must be set using a call to the parent constructor. Any submission that sets these variables directly will earn no credit. The remaining three parameters are used to set minBal, monthlyFee, and interestRate. In all three cases, error checking must be done. If minimum is less than MIN_A then minBal is set using MIN_A. Otherwise minBal is set using minimum. If rate is less than MIN_VAL, then interestRate is set using MIN_VAL. Otherwise interestRate is set using rate. And finally if fee is less than MIN_VAL, then monthlyFee is set using MIN_VAL. Otherwise monthlyFee is set using fee.
public String toString(): This method constructs and returns an output String for the calling MMAccount that consists of the name, account number, balance, fee, minimum balance, and interest rate. The first three must be obtained using a call to the Account toString method. The String returned from that call should be appended onto the remaining information, with a newline placed between the String returned by the Account toString method and the information added onto it. The fee should be displayed to two decimal places, the balance to two decimal places, and the interest rate to four decimal places. Any submission that does not call the Account toString method will earn no credit.
public void processFee(): This method determines whether a monthly fee will be charged for the account. It checks if the balance is less than the minimum balance, and if it is, it deducts the monthly fee from the balance. If in the process the balance drops below MIN_VAL, the balance is reset to MIN_VAL.
public void computeInterest(): This method determines whether the account will earn interest in this monthly period. It checks if the balance is greater than or equal to the minimum balance. If it is, then it increases the balance by the amount earned on an interest rate divided by 12 times 100 (to convert the interest rate from its whole representation to its decimal representation and to adjust for the fact that most interest rates are annual). If the balance is below the minimum balance, nothing is changed.
I have provided a driver program (e.g. a main method that uses the MMAccount class) TestMMAccount.java to help you test your code which has been posted to D2L. Be aware that the grader may add to that program when grading, so if you feel that any of your methods haven't been tested effectively by the driver program, please modify as necessary. However, you should only submit the MMAccount.java class.
Account.java:
// You must use this package package account;
// Do not modify this class public class Account { protected String name; protected String acctNum; protected double balance;
// Default constructor public Account() { System.out.println("Account default constructor called."); }
// Other constructor public Account(String inName, String number, double amount) { System.out.println("Account constructor called."); name = inName; acctNum = number; balance = amount; }
public void deposit(double depositAmount) { balance = balance + depositAmount; }
public void withdraw(double withdrawAmount) { balance = balance - withdrawAmount; } public boolean equals(Account other) { return (name.equals(other.name) && acctNum.equals(other.acctNum)); } public String toString() { return String.format("Name: %s, account #: %s, balance: $%.2f", name, acctNum, balance); } }
_______________________________________________________________
MMAccount.java:
// Use must use this package name // You must use this package package account;
// Put your name here // Put your collaboration statement here
public class MMAccount extends Account {
private double minBal; private double monthlyFee; private double interestRate; private final double MIN_A = 1000.0; private final double MIN_VAL = 0.0; // The method must make a call to the Account constructor to set the // name, account number, and balance public MMAccount(String inName, String number, double amount, double minimum, double fee, double rate) { } public String toString() { // stub -- remove when you write the method return ""; } public void processFee() { } public void computeInterest() { } }
_______________________________________________________________
TestMMAccount.java:
// Use must use this package package hw6;
import account.MMAccount;
public class TestMMAccount {
public static void main(String[] args) { MMAccount acct1 = new MMAccount("Djengo Settle", "12345", 100.0, 500.0, 50.0, 1.0); System.out.println("Account 1: " + acct1); System.out.println("Processing the monthly fee for Account 1 ..."); acct1.processFee(); System.out.println("Account 1: " + acct1); System.out.println("Processing the monthly interest for Account 1 ..."); acct1.computeInterest(); System.out.println("Account 1: " + acct1); System.out.println(); MMAccount acct2 = new MMAccount("Cookie Settle", "45678", 10000.0, 500.0, 50.0, 1.0); System.out.println("Account 2: " + acct2); System.out.println("Processing the monthly fee for Account 2 ..."); acct2.processFee(); System.out.println("Account 2: " + acct2); System.out.println("Processing the monthly interest for Account 2 ..."); acct2.computeInterest(); System.out.println("Account 2: " + acct2); System.out.println(); MMAccount acct3 = new MMAccount("Joon Elam", "78900", 45.0, 500.0, 50.0, 1.0); System.out.println("Account 3: " + acct3); System.out.println("Processing the monthly fee for Account 3 ..."); acct3.processFee(); System.out.println("Account 3: " + acct3); System.out.println("Processing the monthly interest for Account 3 ..."); acct3.computeInterest(); System.out.println("Account 3: " + acct3); System.out.println(); MMAccount acct4 = new MMAccount("Sochi Hastings", "55588", 350.0, 500.0, 50.0, 1.0); System.out.println("Account 4: " + acct4); System.out.println("Processing the monthly interest for Account 4 ..."); acct4.computeInterest(); System.out.println("Account 4: " + acct4); System.out.println("Processing the monthly fee for Account 4 ..."); acct4.processFee(); System.out.println("Account 4: " + acct4); System.out.println();
}
}
______________________________________________________________________
Below you can see the sample output produced by the TestMMAccount class when it is run in conjunction with a correctly completed MMAccount class. Note that you will need to use two packages for this assignment: account to hold the Account.java and MMAccount.java files and hw6 to hold the TestMMAccount.java file.
Account constructor called. Account 1: Name: Djengo Settle, account #: 12345, balance: $100.00 fee: $50.00, minBal: $1000.00, rate: 1.0000% Processing the monthly fee for Account 1 ... Account 1: Name: Djengo Settle, account #: 12345, balance: $50.00 fee: $50.00, minBal: $1000.00, rate: 1.0000% Processing the monthly interest for Account 1 ... Account 1: Name: Djengo Settle, account #: 12345, balance: $50.00 fee: $50.00, minBal: $1000.00, rate: 1.0000% Account constructor called. Account 2: Name: Cookie Settle, account #: 45678, balance: $10000.00 fee: $50.00, minBal: $1000.00, rate: 1.0000% Processing the monthly fee for Account 2 ... Account 2: Name: Cookie Settle, account #: 45678, balance: $10000.00 fee: $50.00, minBal: $1000.00, rate: 1.0000% Processing the monthly interest for Account 2 ... Account 2: Name: Cookie Settle, account #: 45678, balance: $10008.33 fee: $50.00, minBal: $1000.00, rate: 1.0000% Account constructor called. Account 3: Name: Joon Elam, account #: 78900, balance: $45.00 fee: $50.00, minBal: $1000.00, rate: 1.0000% Processing the monthly fee for Account 3 ... Account 3: Name: Joon Elam, account #: 78900, balance: $0.00 fee: $50.00, minBal: $1000.00, rate: 1.0000% Processing the monthly interest for Account 3 ... Account 3: Name: Joon Elam, account #: 78900, balance: $0.00 fee: $50.00, minBal: $1000.00, rate: 1.0000% Account constructor called. Account 4: Name: Sochi Hastings, account #: 55588, balance: $350.00 fee: $50.00, minBal: $1000.00, rate: 1.0000% Processing the monthly interest for Account 4 ... Account 4: Name: Sochi Hastings, account #: 55588, balance: $350.00 fee: $50.00, minBal: $1000.00, rate: 1.0000% Processing the monthly fee for Account 4 ... Account 4: Name: Sochi Hastings, account #: 55588, balance: $300.00 fee: $50.00, minBal: $1000.00, rate: 1.0000%
Step by Step Solution
There are 3 Steps involved in it
Step: 1
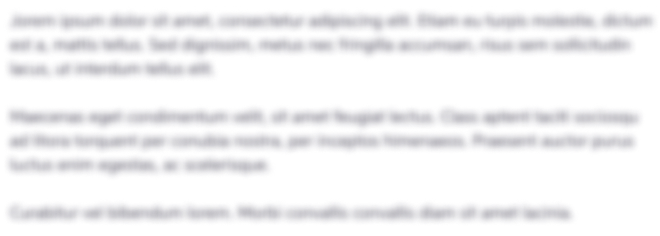
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started