Question
Need help with last part of this java. I can get everything except recognizing the name at the end. I posted the requirements and both
Need help with last part of this java. I can get everything except recognizing the name at the end. I posted the requirements and both java classes.
import java.util. Scanner; import java.io.*; import java.util.ArrayList; public class StudentsQuiz2 {
public static void main(String[] args) throws FileNotFoundException { ArrayList
public class StudentsQuiz { //This class stores information about the student and quiz grades
//Declare class attributes private String sFirstName = ""; //First name of student String sLastName = ""; //Last name of student private int nQuiz1 = 0; //Grade for Quiz 1 private int nQuiz2 = 0; //Grade for Quiz 2 private int nQuiz3 = 0; //Grade for Quiz 3 private static int nNumberStudents = 0; //Number of students //Declare constants public static final int QUIZZES = 3; //Number of quiz grades //Default constructor for Student public StudentsQuiz() { //Update number of students created nNumberStudents++; } //end default constructor //Overloaded constructor for Student public StudentsQuiz(String sFName, String sLName, int nQ1, int nQ2, int nQ3) { //Assign parameter values to class attributes sFirstName = sFName; sLastName = sLName; nQuiz1 = nQ1; nQuiz2 = nQ2; nQuiz3 = nQ3; //Update number of students created nNumberStudents++; } //end overloaded constructor
//Accessor for First Name public String getFirstName() { return sFirstName; } //end accessor for First Name
//Mutator for First Name public void setFirstName(String sFstName) { sFirstName = sFstName; } //end mutator for First Name
//Accessor for Last Name public String getLastName() { return sLastName; } //end accessor for Last Name
//Mutator for Last Name public void setLastName(String sLstName) { sLastName = sLstName; } //end mutator for Last Name
//Accessor for Quiz 1 grade public int getQuiz1() { return nQuiz1; } //end accessor for Quiz 1
//Mutator for Quiz 1 grade public void setQuiz1(int nQz1) { nQuiz1 = nQz1; } //end mutator for Quiz 1
//Accessor for Quiz 2 grade public int getQuiz2() { return nQuiz2; } //end accessor for Quiz 2
//Mutator for Quiz 2 grade public void setQuiz2(int nQz2) { nQuiz2 = nQz2; } //end mutator for Quiz 2
//Accessor for Quiz 3 grade public int getQuiz3() { return nQuiz3; } //end accessor for Quiz 3
//Mutator for Quiz 3 grade public void setQuiz3(int nQz3) { nQuiz3 = nQz3; } //end mutator for Quiz 3 //Accessor for number of students public static int getNumberStudents() { return nNumberStudents; } //end accessor for number of students //Calculates the average of the three quiz grades for the student public double calculateQuizAverage() { double dAverage = 0.0; //Local variable to store quiz average //Calculate quiz average dAverage = (nQuiz1 + nQuiz2 + nQuiz3)/QUIZZES; return dAverage; } //end method calculateQuizAverage } //end class StudentsQuiz
Because there are several different things your program might do depending upon what the user enters, please refer to the examples below to use to test your program. Run your final program one time for each scenario to make sure that you get the expected output.
Be sure to format the output of your program so that it follows what is included in the examples. Remember, in all examples bold items are entered by the user when the program runs (and therefore can change each time the program runs).
Scenario 1: User searches for a student who exists. STUDENT ROSTER AND QUIZ AVERAGES
Students in class: 10
Student Name: Leann ROSENBERRY Quiz average: 85.0
Student Name: Gia WALCK Quiz average: 83.0
Student Name: Archie SELLS Quiz average: 78.0
Student Name: Giuseppe DIMARTINO Quiz average: 73.0
Student Name: Lizabeth GOAD Quiz average: 79.0
Student Name: Paul ELLINGTON Quiz average: 76.0
Student Name: Eusebia TONGUE Quiz average: 79.0
Student Name: Drew BUCHWALD Quiz average: 73.0
Student Name: Lyndsey PACK Quiz average: 66.0
Student Name: Arica BARRETT Quiz average: 69.0
Please enter the last name of a specific student: buchwald Student Name: Drew BUCHWALD Quiz average: 73.0
1
Scenario 2: User searches for a student who does not exist. STUDENT ROSTER AND QUIZ AVERAGES Students in class: 10 Student Name: Leann ROSENBERRY
Quiz average: 85.0
Student Name: Gia WALCK Quiz average: 83.0
Student Name: Archie SELLS Quiz average: 78.0
Student Name: Giuseppe DIMARTINO Quiz average: 73.0
Student Name: Lizabeth GOAD Quiz average: 79.0
Student Name: Paul ELLINGTON Quiz average: 76.0
Student Name: Eusebia TONGUE Quiz average: 79.0
Student Name: Drew BUCHWALD Quiz average: 73.0
Student Name: Lyndsey PACK Quiz average: 66.0
Student Name: Arica BARRETT Quiz average: 69.0
Please enter the last name of a specific student: Smith No student with name Smith found.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
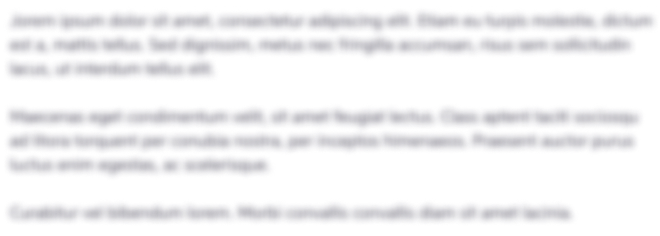
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started