Question
Need help with making the graph like it is in the correct_output.txt here is a snippet of my code // Prints a scaled bar relevant
Need help with making the graph like it is in the correct_output.txt
here is a snippet of my code
// Prints a scaled bar relevant to the maximum value in the samples array
void printBar(int val, int max) {
int i;
int barlength = GRAPH_WIDTH * val / max;
cout << "| ";
for (i = 0; i < barlength; ++i) {
cout << "*";
}
cout << " ";
printInt(val, 0); // Print the sample value right next to the bar
cout << " |" << endl; // Adjusted the formatting
}
// Prints a graph comparing the sample values visually
void printGraph(int samples[], int noOfSamples, const char* label) {
int max = findMax(samples, noOfSamples);
labelLine(GRAPH_WIDTH + 10, label);
for (int i = 0; i < noOfSamples; ++i) {
printBar(samples[i], max);
}
line(GRAPH_WIDTH + 10);
}
and here is the whole set of code that I made with it
graph.cpp
#include "graph.h"
#include "io.h"
#include
using namespace std;
namespace seneca {
// Fills the samples array with the statistic samples
void getSamples(int samples[], int noOfSamples) {
for (int i = 0; i < noOfSamples; ++i) {
line(28);
cout << "\\ " << (i + 1) << "/" << noOfSamples << " : ";
samples[i] = getInt(1, 1000000);
}
}
// Finds the largest sample in the samples array, if it is larger than GRAPH_WIDTH,
// otherwise, it will return GRAPH_WIDTH.
int findMax(int samples[], int noOfSamples) {
int max = samples[0];
for (int i = 1; i < noOfSamples; ++i) {
if (max < samples[i]) max = samples[i];
}
return max < GRAPH_WIDTH ? GRAPH_WIDTH : max;
}
// Prints a scaled bar relevant to the maximum value in the samples array
void printBar(int val, int max) {
int i;
int barlength = GRAPH_WIDTH * val / max;
cout << "| ";
for (i = 0; i < barlength; ++i) {
cout << "*";
}
cout << " ";
printInt(val, 0); // Print the sample value right next to the bar
cout << " |" << endl; // Adjusted the formatting
}
// Prints a graph comparing the sample values visually
void printGraph(int samples[], int noOfSamples, const char* label) {
int max = findMax(samples, noOfSamples);
labelLine(GRAPH_WIDTH + 10, label);
for (int i = 0; i < noOfSamples; ++i) {
printBar(samples[i], max);
}
line(GRAPH_WIDTH + 10);
}
} // namespace seneca
graph.h
#ifndef SENECA_GRAPH_H
#define SENECA_GRAPH_H
namespace seneca {
// Width of the graph
const int GRAPH_WIDTH = 65;
// Function prototypes for graph module
void getSamples(int samples[], int noOfSamples);
int findMax(int samples[], int noOfSamples);
void printBar(int val, int max);
void printGraph(int samples[], int noOfSamples, const char* label);
} // namespace seneca
#endif // SENECA_GRAPH_H
io.cpp
#include "io.h"
#include
#include
#include
using namespace std;
namespace seneca {
// Prints an integer value with a specified field width
void printInt(int value, int fieldWidth) {
cout << setw(fieldWidth) << value;
}
// Returns the number of digits in an integer
int intDigits(int value) {
if (value == 0) return 1;
int count = 0;
while (value != 0) {
value /= 10;
++count;
}
return count;
}
// Performs a fool-proof integer entry
int getInt(int min, int max) {
int val = min - 1;
bool done = false;
while (!done) {
std::cin >> val;
if (cin.fail() || val < min || val > max) {
cin.clear(); // clear error flag
cin.ignore(std::numeric_limits
cout << "Invalid value! Enter a number between " << min << " and " << max << ": ";
}
else {
done = true;
}
}
return val;
}
// Moves the cursor backward
void goBack(int n) {
for (int i = 0; i < n; ++i) {
cout << "\b";
}
}
// Displays the user interface menu
int menu(int noOfSamples) {
line(28);
cout << "| No Of Samples: ";
printInt(noOfSamples, 1);
cout << " |" << endl;
line(28);
cout << "| 1- Set Number of Samples |" << endl;
cout << "| 2- Enter Samples |" << endl;
cout << "| 3- Graphs |" << endl;
cout << "| 0- Exit |" << endl;
cout << "\\ > /";
goBack(24);
return getInt(0, 3);
}
// Draws a line and adds a label
void labelLine(int n, const char* label) {
cout << "+";
for (int i = 0; i < n - 2; ++i) {
cout << "-";
}
cout << "+";
if (label) {
goBack(n - 4);
cout << label;
}
cout << std::endl;
}
// Draws a line
void line(int n) {
cout << "+";
for (int i = 0; i < n - 2; ++i) {
cout << "-";
}
cout << "+";
cout << endl;
}
} // namespace seneca
io.h
#ifndef SENECA_IO_H
#define SENECA_IO_H
namespace seneca {
// Function prototypes for io module
void printInt(int value, int fieldWidth);
int intDigits(int value);
int getInt(int min, int max);
void goBack(int n);
int menu(int noOfSamples);
void labelLine(int n, const char* label);
void line(int n);
} // namespace seneca
#endif // SENECA_IO_H
main.cpp
#include "io.h"
#include "graph.h"
#include
using namespace std;
using namespace seneca;
// Maximum number of samples in an graph
const int MAX_NO_OF_SAMPLES = 20;
void samplesFirst();
// SeneGraph program
int main() {
int noOfSamples = 0;
int samples[MAX_NO_OF_SAMPLES] = { 0 };
bool done = false;
labelLine(33, "Welcome to SeneGraph");
while (!done) {
switch (menu(noOfSamples)) {
case 1:
cout << "Enter number of available samples: ";
noOfSamples = getInt(1, MAX_NO_OF_SAMPLES);
break;
case 2:
if (noOfSamples == 0) {
samplesFirst();
}
else {
cout << "Please enter the sample values: " << endl;
getSamples(samples, noOfSamples);
}
break;
case 3:
if (noOfSamples == 0) {
samplesFirst();
}
else if (samples[0] == 0) {
cout << "Samples missing. Please enter the sample values!" << endl;
}
else {
printGraph(samples, noOfSamples, " SeneGraph ");
}
break;
case 0:
cout << "Thanks for using SeneGraph" << endl;
done = true;
}
}
return 0;
}
// prints samples error message
void samplesFirst() {
cout << "Number of Samples must be set first!" << endl;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
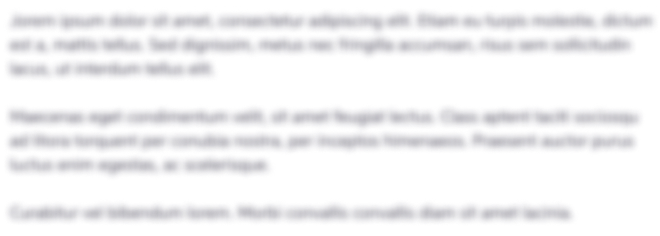
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started