Question
need help with problem 5. i posted functions 1-4 that are supposed to be used as helper functions in python pls you can make your
need help with problem 5. i posted functions 1-4 that are supposed to be used as helper functions
in python pls
you can make your own helper functions in addition to the ones i have created.
Here is the code:
def numToBaseB(N, B):
"""Takes two arguments (an integer and a base) and returns a string
that represents the integer N in base B"""
#return empty string when the value of N is 0
if N == 0:
return ""
else:
if(N%B==0):
return numToBaseB(N//B, B) + "0"
else:
return numToBaseB(N//B, B) + "1"
def baseBToNum(S, B):
"""Accepts a string S that is in base B and returns
the number S in base 10"""
n=len(S)
if S=="":
return 0
else:
return baseBToNum(S[1:], B) + int(S[0])*(B**(n-1))
def baseToBase(B1, B2, SinB1):
"""Accepts a base B1, another base B2, and a string of a number
that is represented in B1; returns the same number in base B2"""
num= baseBToNum(SinB1, B1)
return numToBaseB(num, B2)
def add(S, T):
"""Accepts two binary strings S and T, and returns their sum"""
num1= baseBToNum(S, 2)
num2= baseBToNum(T, 2)
return numToBaseB(num1+num2, 2)
def xorStrings(S1, S2):
"""Accepts a string to encode, S1, and a string representing a one-time
pad, S2; converts them to binary and idk"""
S1= strToInt(S1)
S2= strToInt(S2)
str1= strBinaryRep(S1)
str2= strBinaryRep(S2)
newStr= helpXOR(str1, str2)
return intToChr(newStr)
def helpXOR(S1, S2):
"""Accepts two binary strings and adds them based on XOR"""
if S1== "" or S2=="":
return ""
elif S1[0] == "0" and S2[0] == "0":
return "0" + helpXOR(S1[1:], S2[1:])
elif S1[0] == "1" and S2[0] == "1":
return "0" + helpXOR(S1[1:], S2[1:])
elif S1[0] == "1" and S2[0] == "0":
return "1" + helpXOR(S1[1:], S2[1:])
elif S1[0] == "0" and S2[0] == "1":
return "1" + helpXOR(S1[1:], S2[1:])
Problem 5. Cryptography using one-time pads! In this problem, you'll implement the one-time encryption/decryption algorithm described in class. Here's the idea: Given two strings S1 and 2 of the same length, where si is the string that we wish to encode and s2 is a string representing the one-time pad, we first convert these strings into binary strings. Each character of si or S2 has a corresponding 8-bit representation, and the binary representation of the string is the string created by concatenating all those 8-bit strings. So both strings will be represented by binary sequences whose length is a multiple of 8. Next, we compute the XOR of those two binary strings. Finally, we pack the XOR back into characters and return that string! This is all done by a function called xor Strings (S1, S2). Here are a few examples of this function in action: >>> encrypted xorStrings (spam", "zng!") # "spam" is our secret, "ang!" is the one-time pad >>> encrypted "It\xle\x06L' # There are still four characters here; some are funny-looking control characters >>> len(encrypted) # Now, we'll decrypt using our one-time pad >>> xorStrings (encrypted, "ung!") spam In the above, the strange notation \xle is Python's way of saying "I can't print this character, so here it is as two hexadecimal (base 16) digits instead. The backslash says "something special is next"; the "x" says "the special thing is two hexadecimal digits", and the "1e" is those digits (which represent one 8-bit value) You will need to write several small helper functions to get xorStrings (S1, S2) to do its job. Keep those functions very small and very simple. Other than the functions that you wrote for Problems 1 through 4 (you'll certainly use some of those functions as helper functions!) and other than def lines and doscstrings, the total line count for our implementation (including xorStrings and all the new helper functions) is 13 lines (and it could easily be shorter). Don't write more than 15 lines of code total. If you do, you're probably not using higher order functions and list comprehensions to your full advantage. total. If you ation (includingainly use som def numToBaseB(N, B): ""Takes two arguments (an integer and a base) and returns a string that represents the integer N in base B #return empty string when the value of N is 0 if N == 0: return else: if(N%B==0): return numToBaseB(N//B, B) + "0" else: return numToBaseB(N//B, B) + "1" def baseBToNum(S, B): ""Accepts a string S that is in base B and returns the number S in base 10" n=len(S) if S=="": return else: return baseToNum(S(1:), B) + int(S[0] )*(B**(n-1)), def baseToBase (B1, B2, SinB1): ""Accepts a base Bi, another base B2, and a string of a number that is represented in B1; returns the same number in base B2 num= baseBToNum(SinB1, B1) return numToBaseB (num, B2) def add(S, T): ""Accepts two binary strings S and T, and returns their sum". num1= baseBToNum(S, 2) num2= baseBToNum(T, 2) return numToBaseB(num1+num2, 2) def xorStrings(S1, S2): | ff Problem 5. Cryptography using one-time pads! In this problem, you'll implement the one-time encryption/decryption algorithm described in class. Here's the idea: Given two strings S1 and 2 of the same length, where si is the string that we wish to encode and s2 is a string representing the one-time pad, we first convert these strings into binary strings. Each character of si or S2 has a corresponding 8-bit representation, and the binary representation of the string is the string created by concatenating all those 8-bit strings. So both strings will be represented by binary sequences whose length is a multiple of 8. Next, we compute the XOR of those two binary strings. Finally, we pack the XOR back into characters and return that string! This is all done by a function called xor Strings (S1, S2). Here are a few examples of this function in action: >>> encrypted xorStrings (spam", "zng!") # "spam" is our secret, "ang!" is the one-time pad >>> encrypted "It\xle\x06L' # There are still four characters here; some are funny-looking control characters >>> len(encrypted) # Now, we'll decrypt using our one-time pad >>> xorStrings (encrypted, "ung!") spam In the above, the strange notation \xle is Python's way of saying "I can't print this character, so here it is as two hexadecimal (base 16) digits instead. The backslash says "something special is next"; the "x" says "the special thing is two hexadecimal digits", and the "1e" is those digits (which represent one 8-bit value) You will need to write several small helper functions to get xorStrings (S1, S2) to do its job. Keep those functions very small and very simple. Other than the functions that you wrote for Problems 1 through 4 (you'll certainly use some of those functions as helper functions!) and other than def lines and doscstrings, the total line count for our implementation (including xorStrings and all the new helper functions) is 13 lines (and it could easily be shorter). Don't write more than 15 lines of code total. If you do, you're probably not using higher order functions and list comprehensions to your full advantage. total. If you ation (includingainly use som def numToBaseB(N, B): ""Takes two arguments (an integer and a base) and returns a string that represents the integer N in base B #return empty string when the value of N is 0 if N == 0: return else: if(N%B==0): return numToBaseB(N//B, B) + "0" else: return numToBaseB(N//B, B) + "1" def baseBToNum(S, B): ""Accepts a string S that is in base B and returns the number S in base 10" n=len(S) if S=="": return else: return baseToNum(S(1:), B) + int(S[0] )*(B**(n-1)), def baseToBase (B1, B2, SinB1): ""Accepts a base Bi, another base B2, and a string of a number that is represented in B1; returns the same number in base B2 num= baseBToNum(SinB1, B1) return numToBaseB (num, B2) def add(S, T): ""Accepts two binary strings S and T, and returns their sum". num1= baseBToNum(S, 2) num2= baseBToNum(T, 2) return numToBaseB(num1+num2, 2) def xorStrings(S1, S2): | ffStep by Step Solution
There are 3 Steps involved in it
Step: 1
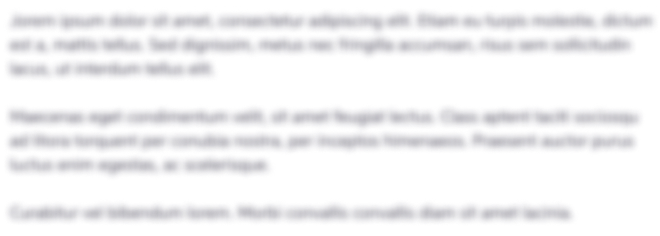
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started