Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Need help with python code following: Making the Employee class an abstract class Adding a ShiftSupervisor subclass of employee with an accessor and mutator method
Need help with python code following:
Making the Employee class an abstract class
Adding a ShiftSupervisor subclass of employee with an accessor and mutator
method for each of the two added subclass attributes, annual salary and
annual production bonus that a shift supervisor has earned. Naming the
accessor method getbonus for the annual production bonus.
My code part
class Employee:
def setnameself name:
self.name name
def setidnumberself idnumber:
self.idnumber idnumber
def getnameself:
return self.name
def getidnumberself:
return self.idnumber
class ProductionWorkerEmployee:
def setshiftnumberself shiftnumber:
self.shiftnumber shiftnumber
def setpayrateself payrate:
self.payrate payrate
def getshiftnumberself:
return self.shiftnumber
def getpayrateself:
return self.payrate
class ShiftSupervisorEmployee:
def setannualsalaryself annualsalary:
self.annualsalary annualsalary
def setannualbonusself annualbonus:
self.annualbonus annualbonus
def getannualsalaryself:
return self.annualsalary
def getannualbonusself:
return self.annualbonus
My code part two:
from employee import
def main:
workername inputEnter the name:
workerid inputEnter the ID number:
workershift intinputEnter the shift number:
workerpay floatinputEnter the hourly pay rate:
# Create an instance of ProductionWorker
worker ProductionWorker
worker.setnameworkername
worker.setidnumberworkerid
worker.setshiftnumberworkershift
worker.setpayrateworkerpay
# Display worker information
printProduction worker information:
printfName: workergetname
printfID number: workergetidnumber
printfShift: workergetshiftnumber
printfHourly Pay Rate: $workergetpayrate:f
# You can add similar code for ShiftSupervisor here after instantiating it
def showworkerworker:
printfName: workergetname
printfID Number: workergetidnumber
if isinstanceworker ProductionWorker:
printfShift Number: workergetshiftnumber
printfHourly Pay Rate: $workergetpayrate:f
elif isinstanceworker ShiftSupervisor:
printfAnnual Salary: $workergetannualsalary:f
printfAnnual Production Bonus: $workergetannualbonus:f
main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
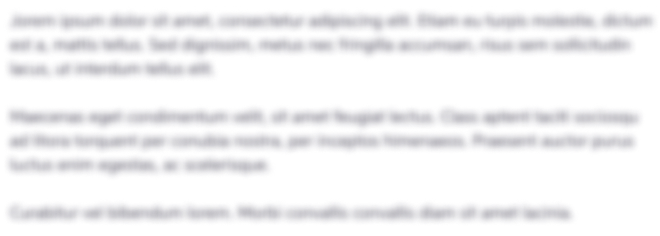
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started