Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Need help with the code must be in c++ Ill post pictures from the book, and the requirements it needs to fulfill also the code
Need help with the code must be in c++ Ill post pictures from the book, and the requirements it needs to fulfill also the code will be provide from the book. Please help instructions are below please help and thank you.
Its up to you to define the intArray class, but it should fulfill the following requirements:
1) with a single integer giving the number of elements in which case that indices will run from 0 to one less than the number of elements.
2) with two integers which will be taken as a lower and upper indices. it is an error for the first index to be greater than the second index but having both indices the same specifies a valid single element array
3) with no integers which creates a ten-element array who indices run from 0 to 9.
4) with another object of type intarray. the new object will copy of the old one with each entry in the index range duplicated.
*except in case 4 the initial array elements are undefined once an intArray object has been created its size cannot be changed in each object reviews just enough storage to hold its elements plus a fixed amount of space for bookkeeping information
*elements of an intArray are accessed using the standard c style array indexing notation. If the index falls outside the legal range, an error message should be printed, and the program should simulate a halt
* intArray objects may be assigned to each other if they have the same number of elements. the indices need not match and the indices of the target object will not change only the elements will be copied if the sizes of the objects been assigned dont match and error message should be printed and the program should stimulate a halt
* two intArray objects can be compared using the == and != operators. If the arrays have the same number of elements and each of the corresponding elements match the objects are equal and the comparison operator (==) should return a non-zero value, even if their indices are different. Comparing intArray objects with different number of elements is legal, and the results is always zero. The != operator should return zero whenever == would return non-zero, and vice versa.
* class intArray should also overload operators below. An error should be generated if the array are of a different size.
[ ] - allows index range checking
+ - allows the sum of two range to be assigned to a third array
+= - allows the sum of two arrays to be assigned to the first array
*The intArray class should have high() and low() member functions that return the maximum and minimum legal index for a given array as defined by the driver (user).
* the intArray class should also contain a private data memeber that will hold the name of the intArray object when instantiated. This data member should be initialized with a call to a setname() memeber function.
I will supply a driver program, iadrv.cpp that demonstrates the use of the intArray class. The driver will demonstrate all operation and error condition features separately. Each test will note what feature is being demonstrated and output each array name, index, and corresponding element using the overloaded
**note that for array test data, the driver will simply multiply the array index by 10 immediately after each array is initialized or modified and output its contents. When your program encounters a run time error, you should simulate a half with appropriate diagnostics rather than actually halting the program. Be sure that that all error messages sent to the terminal window are also sent to the csis.txt output file.
When the elements of an array are output, the array name should be output, a left bracket, an index value, a right bracket, an equal sign, and finally a value. For example
a [3] = 30
***Note that the driver program outputs array elements vertically down the page. This behavior should not be changed!!!! Be sure that you include a destructor for the intArray class. The driver program will include the follow test




The driver program is below
-------------------------------------------------------------------------------------------------------------------------
// iadrv.h
#ifndef _IADRV_H
#define _IADRV_H
#include "intarray.h"
int main();
void test1();
void test2();
void test3();
void test4();
void test5();
void test6();
void test7();
void test8();
void test9();
void test10();
void test11();
void test12();
void test13();
void test14();
void test15();
void test16();
void test17();
void test18();
void test19();
void test20();
void wait();
#endif
-------------------------------------------------------------------------------------------------------------------------
// iadrv.cpp - driver program for testing IntArray class
#include
#include
#include
#include
#include "iadrv.h"
using namespace std;
ofstream csis;
int main() {
csis.open("csis.txt");
test1();
test2();
test3();
test4();
test5();
test6();
test7();
test8();
test9();
test10();
test11();
test12();
test13();
test14();
test15();
test16();
test17();
test18();
test19();
test20();
csis.close();
}
void test1() {
cout
csis
IntArray a(10);
for(int i = a.low(); i
a[i] = i * 10;
a.setName("a");
cout
csis
wait();
}
void test2() {
cout
csis
IntArray b(-3, 6);
for(int i = b.low(); i
b[i] = i * 10;
b.setName("b");
cout
csis
wait();
}
void test3() {
cout
csis
IntArray c(6, 8);
for(int i = c.low(); i
c[i] = i * 10;
c.setName("c");
cout
csis
wait();
}
void test4() {
cout
csis
IntArray d(5, 5);
for(int i = d.low(); i
d[i] = i * 10;
d.setName("d");
cout
csis
wait();
}
void test5() {
cout
csis
IntArray z;
for(int i = z.low(); i
z[i] = i * 10;
z.setName("z");
cout
csis
wait();
}
void test6() {
cout
cout
csis
csis
IntArray c(6, 8);
for(int i = c.low(); i
c[i] = i * 10;
c.setName("c");
cout
csis
IntArray e(c);
e.setName("e");
cout
csis
wait();
}
void test7() {
cout
cout
cout
csis
csis
csis
IntArray f(1, 4);
for(int i = f.low(); i
f[i] = i * 10;
f.setName("f");
cout
csis
IntArray g(5, 8);
for(int i = g.low(); i
g[i] = i * 10;
g.setName("g");
cout
csis
wait();
f = g;
cout
cout
csis
csis
wait();
}
void test8() {
cout
cout
cout
cout
csis
csis
csis
csis
IntArray j(3, 6);
for(int i = j.low(); i
j[i] = i * 10;
j.setName("j");
cout
csis
IntArray k(6, 9);
for(int i = k.low(); i
k[i] = i * 10;
k.setName("k");
cout
csis
IntArray l(1, 4);
for(int i = l.low(); i
l[i] = i * 10;
l.setName("l");
cout
csis
wait();
j = k = l;
cout
cout
cout
csis
csis
csis
wait();
}
void test9() {
cout
cout
cout
csis
csis
csis
IntArray m(3, 7);
for(int i = m.low(); i
m[i] = i * 10;
m.setName("m");
cout
csis
IntArray n(1, 5);
for(int i = n.low(); i
n[i] = i * 10;
n.setName("n");
cout
csis
wait();
m = n;
cout
cout
cout
csis
csis
csis
wait();
}
void test10() {
cout
cout
cout
csis
csis
csis
IntArray o(3, 7);
for(int i = o.low(); i
o[i] = i * 10;
o.setName("o");
cout
csis
IntArray p(1, 5);
for(int i = p.low(); i
p[i] = i * 10;
p.setName("p");
cout
cout
csis
csis
wait();
}
void test11() {
cout
cout
cout
csis
csis
csis
IntArray q(1, 3);
for(int i = q.low(); i
q[i] = i * 10;
q.setName("q");
cout
csis
IntArray r(1, 4);
for(int i = r.low(); i
r[i] = i * 10;
r.setName("r");
cout
cout
csis
csis
wait();
}
void test12() {
cout
cout
cout
csis
csis
csis
IntArray s(3, 7);
for(int i = s.low(); i
s[i] = i * 10;
s.setName("s");
cout
csis
IntArray t(1, 5);
for(int i = t.low(); i
t[i] = i * 10;
t.setName("t");
cout
csis
wait();
s = t;
cout
cout
cout
csis
csis
csis
wait();
}
void test13() {
cout
cout
cout
csis
csis
csis
IntArray u(3, 7);
for(int i = u.low(); i
u[i] = i * 10;
u.setName("u");
cout
csis
IntArray v(1, 5);
for(int i = v.low(); i
v[i] = i * 10;
v.setName("v");
cout
cout
csis
csis
wait();
}
void test14() {
cout
cout
cout
csis
csis
csis
IntArray w(1, 3);
for(int i = w.low(); i
w[i] = i * 10;
w.setName("w");
cout
csis
IntArray x(1, 4);
for(int i = x.low(); i
x[i] = i * 10;
x.setName("x");
cout
cout
csis
csis
wait();
}
void test15() {
cout
cout
cout
csis
csis
csis
IntArray a(1, 5);
for(int i = a.low(); i
a[i] = i * 10;
a.setName("a");
cout
csis
IntArray b(4, 8);
for(int i = b.low(); i
b[i] = i * 10;
b.setName("b");
cout
csis
wait();
IntArray c = a + b;
c.setName("c");
cout
csis
wait();
}
void test16() {
cout
cout
cout
csis
csis
csis
IntArray d(10, 13);
for(int i = d.low(); i
d[i] = i * 10;
d.setName("d");
cout
csis
IntArray e(30, 33);
for(int i = e.low(); i
e[i] = i * 10;
e.setName("e");
cout
csis
d += e;
cout
csis
wait();
}
void test17() {
cout
csis
IntArray f(5, 2);
for(int i = f.low(); i
f[i] = i * 10;
f.setName("f");
cout
csis
wait();
}
void test18() {
cout
cout
csis
csis
IntArray g(10);
for(int i = g.low(); i
g[i] = i * 10;
g.setName("g");
cout
csis
g[10] = 1;
wait();
}
void test19() {
cout
cout
cout
csis
csis
csis
IntArray m(1, 4);
for(int i = m.low(); i
m[i] = i * 10;
m.setName("m");
cout
csis
IntArray n(2, 4);
for(int i = n.low(); i
n[i] = i * 10;
n.setName("n");
cout
csis
wait();
m = n;
cout
cout
csis
csis
wait();
}
void test20() {
cout
cout
cout
csis
csis
csis
IntArray o(7, 8);
for(int i = o.low(); i
o[i] = i * 10;
o.setName("o");
cout
csis
o[7] = 25;
o[8] = o[7];
cout
csis
the De sure that you include a destru s. The driver program will include the following tests: he Test array declared with single integer: IntArray a (10); declared with two integers, one of which is negative: 2 Test array decl IntArray b (-3, 6); 3. Test array declared with two non-negative integers: IntArray c(6, 8) 4. Test array declared with two identical integers: IntArray d (5, 5) Page rocus on Object-Oriented Programming with C wait();
}
void wait() {
char buf;
cout
cin.get(buf);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
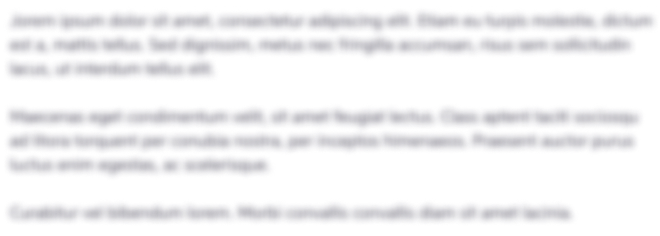
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started