Question
Need help with this c++ course project. Add arrays to your program to handle more data. Examples: In the programming tutorial, add a tutorial on
Need help with this c++ course project.
Add arrays to your program to handle more data.
Examples: In the programming tutorial, add a tutorial on working with arrays. Then use arrays to store the answers to multiple questions so that you can compute a total score.
Here is my code:
#include "stdafx.h"
#include
#include
using namespace std;
int nMonths = 0;
float loanAmount;
float totalPaid = 0.0f;
//process function
void process() {
float interestRate;
float monthlyPayment;
cout << "What is the amount of the loan?:$";
cin >> loanAmount;
cin.ignore();
//validation amount should be greater than 0
while (loanAmount<0)
{
cout << " loan amount should be greater than 0..What is the amount of the loan?:$";
cin >> loanAmount;
}
cout << "What is the interest rate on the loan?:$";
cin >> interestRate;
interestRate /= 100;
cin.ignore();
cout << "What is the desired monthly payment?:$";
cin >> monthlyPayment; cin.ignore();
while (monthlyPayment<0) {
cout << " Monthly payment should be greater than 0..What is the desired monthly payment?:$";
cin >> monthlyPayment;
}
while (monthlyPayment < loanAmount * interestRate / 12) {
cout << "Not a valid amount, Amount must be greater than $";
cout << (loanAmount * interestRate / 12) << endl;
cout << "What is the desired monthly payment amount? $";
cin >> monthlyPayment; cin.ignore();
}
float interestToAdd = 0.0f;
float remaining = loanAmount;
float toAdd = 0.0f;
cout << endl;
cout << "Month" << " (Interest Added)" << " (Amount Paid)" << " (Debt Remaining)" << endl;
while (remaining > monthlyPayment) {
interestToAdd = remaining * interestRate / 12;
nMonths++;
remaining += interestToAdd;
if (remaining > monthlyPayment) {
toAdd = monthlyPayment;
}
else {
toAdd = remaining;
}
cout << nMonths << " $" << interestToAdd << " $" << toAdd
<< " $" << remaining << endl;
totalPaid += toAdd;
remaining -= toAdd;
}
}
//display the result function
void displayResult() {
cout << endl << "Statistics about loan. " << endl;
cout << "Initial loan amount: $" << loanAmount << endl;
cout << "Total amount paid: $" << totalPaid << endl;
cout << "Time to pay off loan: " << nMonths << endl;
cout << "Overpay percentage: " << (totalPaid / loanAmount) << endl;
cin.ignore();
}
//choose letterA function
void chooseletterA() {
float Amount, rateOfIntrest, monthlyAmountToPay, totalAmount;
int lengthOfLoan;
cout << "What is the amount of the loan?:$";
cin >> Amount;
cin.ignore();
while (Amount<1) //validate
{
cout << " Amount should be greater than 0..What is the amount of the loan?:$";
cin >> Amount;
}
cout << "What is the interest rate on the loan?";
cin >> rateOfIntrest;
rateOfIntrest /= 100;
cin.ignore();
cout << "What is the length of loan?:$";
cin >> lengthOfLoan;
while (lengthOfLoan<1)
{
cout << "length of loan should be greater than 0..What is the length of loan?:$";
cin >> lengthOfLoan;
}
monthlyAmountToPay = (Amount*rateOfIntrest*pow((1 + rateOfIntrest), lengthOfLoan)) / (pow((1 + rateOfIntrest), lengthOfLoan - 1));
cout << "Your monthly loan payment amount = $" << monthlyAmountToPay << endl;
system("pause");
}
//chooseletterB function
void chooseletterB() {
float Amount, rateOfIntrest, monthlyAmountToPay, totalAmount;
int lengthOfLoan;
cout << "What is the monthly amount of the loan?:$";
cin >> monthlyAmountToPay;
cin.ignore();
cout << "What is the interest rate on the loan?";
cin >> rateOfIntrest;
rateOfIntrest /= 100;
cin.ignore();
cout << "What is the length of loan?:$";
cin >> lengthOfLoan;
cin.ignore();
Amount = monthlyAmountToPay * pow((1 + rateOfIntrest), lengthOfLoan - 1) / (rateOfIntrest*pow((1 + rateOfIntrest), lengthOfLoan));
cout << "Your total loan amount = $" << Amount << endl;
system("pause");
}
//chooseletterc function
void chooseletterC() {
float Amount, rateOfIntrest, monthlyAmountToPay, totalAmount;
int lengthOfLoan;
cout << "What is the amount of the loan?:$";
cin >> Amount;
cin.ignore();
cout << "What is the monthly amount of the loan?:$";
cin >> monthlyAmountToPay;
cin.ignore();
cout << "What is the length of loan?:$";
cin >> lengthOfLoan;
cin.ignore();
rateOfIntrest = ((monthlyAmountToPay *lengthOfLoan) - Amount) / lengthOfLoan;
cout << "Your monthly monthly rate of intrest = $" << rateOfIntrest / 12 << endl;
system("pause");
}
//chooseletter D function
void chooseletterD() {
float Amount, rateOfIntrest, monthlyAmountToPay, totalAmount;
int lengthOfLoan;
cout << "What is the amount of the loan?:$";
cin >> Amount;
cin.ignore();
cout << "What is the monthly amount of the loan?:$";
cin >> monthlyAmountToPay;
cin.ignore();
cout << "What is the interest rate on the loan?";
cin >> rateOfIntrest;
rateOfIntrest /= 100;
cin.ignore();
lengthOfLoan = (log(Amount) - log(monthlyAmountToPay)) / (log(1 + rateOfIntrest));
cout << "Your length of loan amount = $" << lengthOfLoan << endl;
system("pause");
}
//multiple choice function
void multipleChoice() {
cout << "Welcome to the multiple choice part of the program, please choose a letter: " << endl;
cout << "A: Monthly payment " << endl;
cout << "B: Loan amount " << endl;
cout << "C: Length of loan " << endl;
cout << "D: Interest rate " << endl;
char ch;
cin >> ch;
//validate the input
while (!(ch >= 'A' && ch <= 'D')) {
cout << " Invalid input..please choose a letter:(A - D): ";
cin >> ch;
}
switch (ch)
{
case 'A':
chooseletterA();
cout << "First question: Please solve for monthly payment. " << endl;
break;
case 'B':
chooseletterB();
cout << "Second question: Please solve for loan amount. " << endl;
break;
case 'C':
chooseletterC();
cout << "Third question: Please solve for length of loan. " << endl;
break;
case 'D':
chooseletterD();
cout << "Forth question: Please solve for interest rate. " << endl;
break;
}
}
int main() {
int ch;
do {
cout << endl << "************************MENU***************************" << endl;
cout << "1. Process Loan" << endl;
cout << "2. Display Result" << endl;
cout << "3. Multiple Choice" << endl;
cout << "4. Exit" << endl;
cout << "Enter your choice : ";
cin >> ch;
system("pause");
//validate the input
while (!(ch>0 && ch <5)) {
cout << "Please enter a valid choice : ";
cin >> ch;
}
switch (ch) {
case 1:
process();
break;
case 2:
displayResult();
break;
case 3:
multipleChoice();
break;
//exit function
case 4:
cout << " Thank you ";
return 1;
default:
cout << "Entered Wrong Keyword.... Re-enter Again";
break;
}
} while (ch<3 && ch> 0);
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
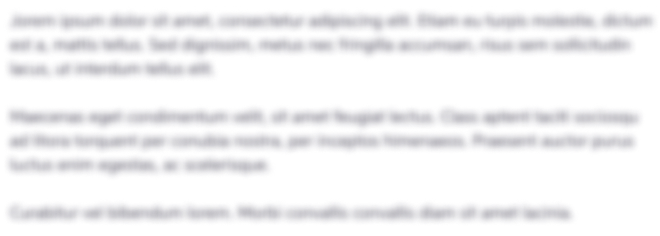
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started