Question
Need help with this java coding assingment Using the operations defined for a singly linked List data structure, given in the MyLinkedList interface on our
Need help with this java coding assingment
Using the operations defined for a singly linked List data structure, given in the MyLinkedList interface on our ecampus page. Write a NEW instance method called replace that replaces the contents of a specific element in the list. This method receives 2 parameters: an element index, and the value to be placed into the node. It is added to the implementation of a singly linked list provided in the linked list folder on ecampus. you will only turn in the code for this method.
Using the operations defined for a singly linked List data structure, given in the MyLinkedList interface on our ecampus page. Write a NEW instance called find, which overloads the find method. This version offindbegins searching for a node containing a specified value at a particular position in the list. If NO node is found containing the value this method should return -1. It is added to the implementation of a singly linked list provided in the linked list folder on ecampus. you will only turn in the code for this method.
Using the operations defined for a singly linked List data structure, given in the MyLinkedList interface on our ecampus page. Write a NEW instance called sortAscending. This method performs the bubble sort and sorts the elements in the list in ascending order, it has no parameters. It is added to the implementation of a singly linked list provided in the linked list folder on ecampus. you will only turn in the code for this method. DIFFICULT
Using the operations defined for a doubly linked List data structure, given in the MyLinkedList interface on our ecampus page. Write a NEW instance called toStringReverse(). This method is specific to a doubly linked list and is not part of the MyLinkedList interface. This method creates a comma delimited list of the lists contents in reverse. It has NO parameters and returns a value of type String.
Using the operations defined for a doubly linked List data structure, given in the MyLinkedList interface on our ecampus page. Write a NEW instance called addAscending. This method is specific to our doubly linked list and is not part of the MyLinkedList interface. This method receives a single parameter which is the value to be inserted into the list. Since this is a generic list we may ASSUME that the class on which the list is instantiated implements the Comparable interface [thus contains a compareTo method].
What changes would we make to the generics in the first line of the doubly linked list class to insure that any class it is instantiated MUST implement the Comparable interface?
public class SLinkedList implements MyLinkedList{ // this is an "inner" class that is contained // inside sLinkedList and represents a "contained" // node class that is totally private to // SLinkedList private static class Node { S item; Node next; public Node (S newItem) { item = newItem; next=null; } public Node (S newItem, Node nextNode) { item = newItem; next = nextNode; } }// end class node // Data members of SLinkedList private Node head; private int currentSize; public SLinkedList(){ currentSize =0; head = null; } public boolean isEmpty(){ return (currentSize==0); }// end isempty public int size(){ return currentSize; }// end size /** * This method deletes all of the list's contents. * * @author Camille Hayhurst * */ public void clear(){ head = null; currentSize =0; }// end clear /** * This method searches the list for the * specified value and returns the index * number of the first element containing * the value or -1 if the value is * not found. * @param value: the search value * @return index of element containing value or -1 * */ public int find( S value){ int position = 0; int returnValue = -1; Node nextNode; nextNode = head; while ( nextNode != null){ if( nextNode.item.equals( value)) { returnValue = position; break; } position++; nextNode = nextNode.next; } return returnValue; } public S get (int position) { // This method retrieves the value stored in a node in the list // returns the value, but does not unlink the node. The method // receives the logical position of the node in the list. S returnValue=null; Node nextNode; // inchworm variable if(position > currentSize-1 || position < 0) throw new ListException("No such position"); else{ nextNode = head; for(int i=0; i< position; i++){ nextNode = nextNode.next; } // after the loop nextNode points to the node // containing the value we want. returnValue = (S)nextNode.item; }// end else return returnValue; }// end getvalue /** * This method inserts the value at the given position. * @param position location where new value is to be inserted, 0<=position<=current size * @param value new value to be added to the list * */ public void add( int position, S value){ Node currentNode; if (position < 0 || position > currentSize) throw new ListException("Invalid position given"); currentNode = head; if (position == 0) head = new Node( value, head); else{ for ( int i=0; i < position-1; i++){ currentNode = currentNode.next; } //I will be at the new node's predecessor currentNode.next = new Node( value, currentNode.next); /* more readable solution * Node newNode = new Node( value, currentNode.next); * currentNode.next = newNode;*/ } currentSize++; } /** * This method inserts the value at the end of the list. * @param value new value to be added to the list * */ public void add( S value){ this.add( this.currentSize, value); } /** * This method removes and RETURNS the value at the location * indicated by position. * @param position location of value to remove from the list 0<=position currentNode; if (position < 0 || position >= this.currentSize) { throw new ListException("error"); } if (position == 0){ returnValue = (S) head.item; head = head.next; } else{ currentNode = head; for ( int currentPos = 0; currentPos < position - 1; currentPos++) { currentNode = currentNode.next; } Node nodeToDelete = currentNode.next; returnValue = (S) nodeToDelete.item; currentNode.next = nodeToDelete.next; } this.currentSize--; return returnValue; } /** * This method removes all occurrences of elements in the list argument from the list * */ public void removeAll(MyLinkedList list){ // iterate through the list parameter removing each occurrence of // the values it contains boolean result=false; S temp; int pos=-1; for (int i=0; i < list.size(); i++){ temp = list.get(i); // retrieve the ith element pos = this.find(temp); while(pos != -1){ result = true; this.remove(pos); // remove the element pos = find( temp ); }// end while }// end for } // to string method public String toString(){ String returnValue=""; Node current =head; while(current != null){ returnValue = returnValue + current.item + " "; current = current.next; } return returnValue; } }// end class
public class ListException extends RuntimeException{ public ListException ( String message){ super(message); } }
public interface MyLinkedList{ /** * This method returns true if the current * size of the list is zero. * */ public boolean isEmpty(); /** * This method returns the current number * of elements in the list. * */ public int size(); /** * This method searches the list for the * specified value and returns the index * number of the first element containing * the value or -1 if the value is * not found. * @param value: the search value * @return index of element containing value or -1 * */ public int find( S value); /** * This method returns the value at a specific * position in the list. * @param position: location of element to return 0<=positionlist); /** * This method deletes all of the list's contents. * */ public void clear(); /** * This method produces a comma separated list of the list elements * */ public String toString(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
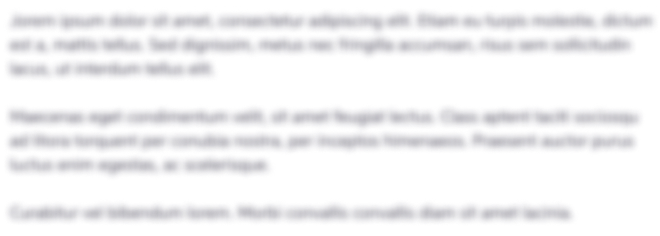
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started