Question
// Need help with this, Thanks Needs to be written in C. Synchronization with Semaphores Producer-Consumer Problem: We will deal with a simple version of
// Need help with this, Thanks
Needs to be written in C.
Synchronization with Semaphores Producer-Consumer Problem: We will deal with a simple version of producer-consumer problem. We will be focusing on the bounded buffer version of the problem. So, assume that the common buffer that the producer and the consumer share is an array of some fixed size (say 10). You shall implement the producer and consumer as two threads of the same process.
The producer will be generating x number of random numbers, where x is a command line parameter to your program. The producer will simply place the random number generated into the buffer. The producer should also write the number generated into a file called production. The producer should also write each number produced on the terminal with the prefix producer. The random number generated should be an integer between 1 and 100.
Note that random numbers can be produced in C using rand function. In order to generate a different sequence of random numbers every time you run the program, you can use the srand function to seed the random number generator with a value that will be different in each run (for example, you can seed with the current time obtained by calling the time function).
The consumer will simply pick up an item from the buffer and determine its square. The consumer should also write the number picked up and its square into a file called consumption. The consumer should also write each number consumed and its square on the terminal with the prefix consumer. The consumer should consume the items in exactly the same order in which they are produced.
The buffer array and any other variables used by both threads can be declared as global variables. Make sure to synchronize the producer thread and the consumer thread properly using semaphores. Note that x has to be much larger in comparison to buffer size to test the synchronization of the threads.
Warning: Do not use mutex locks and condition variables as discussed in the textbook. Instead use general semaphores in the implementation.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
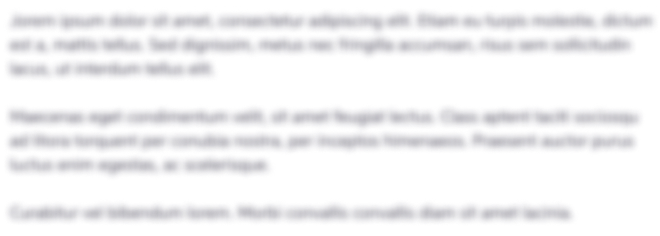
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started