Question
Need help. write a C program stack-ptr.c that implements a stack using a link list. Below is a skeleton code to start with.Jjust edit to
Need help. write a C program stack-ptr.c that implements a stack using a link list. Below is a skeleton code to start with.Jjust edit to make thread friendly.
examplpe:
push(5, &top);
push(10, &top);
push(15, &top);
int value = pop(&top);
value = pop(&top);
value = pop(&top);
this program currently has a race condition. use Pthread mutex locks to fix the race conditions. test you now thread safe stack by creating 200 concurrent threads in main() that push and pop values.
-use loop in main to create the threads
-write a teststack function, and use it as the entry point for each thread
-testStack function should intermis 3 push operations and 3 pop operations in a loop that executes 500 times
-all threads use the same stack
/* * Stack containing race conditions */
#include
#include
#include
// Linked list node
typedef int value_t;
typedef struct Node {
value_t data;
struct Node *next;
} StackNode;
// Stack function declarations
void push (value_t v, StackNode **top);
value_t pop ( StackNode **top);
int is_empty( StackNode *top);
int main(void) {
StackNode *top = NULL;
push(5, &top);
push(10,&top);
pop ( &top);
push(15,&top);
pop ( &top);
pop ( &top);
push(20,&top);
push(-5, &top);
pop ( &top);
push(-10,&top);
pop ( &top);
pop ( &top);
push(-15,&top);
pop ( &top);
push(-20,&top);
return 0;
}
// Stack function definitions
void push(value_t v, StackNode **top)
{
StackNode * new_node = malloc(sizeof(StackNode));
new_node->data = v;
new_node->next = *top;
*top = new_node;
}
value_t pop(StackNode **top)
{
if (is_empty(*top)) return (value_t)0;
value_t data = (*top)->data;
StackNode * temp = *top;
*top = (*top)->next;
free(temp);
return data;
}
int is_empty(StackNode *top) {
if (top == NULL) return 1;
else return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
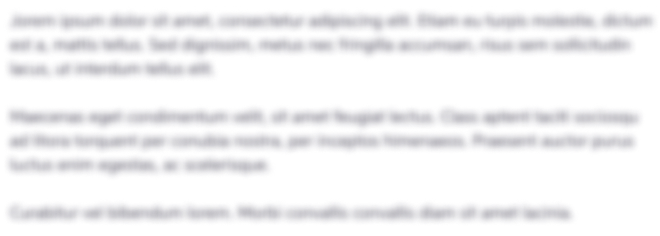
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started