Question
Need help writing Java code using Jcomponent. Some classes are given to help assist. public class GraphicView extends JPanel { /** * Informs the Easel
Need help writing Java code using Jcomponent. Some classes are given to help assist.
public class GraphicView extends JPanel {
/**
* Informs the Easel of what type of shape to generate.
*/
private class ShapeListener implements ActionListener {
@Override
public void actionPerformed(final ActionEvent e) {
final String type = ((JButton) e.getSource()).getText();
GraphicView.this.easel.defineShape(type);
}
}
/**
* @param args
*/
public static void main(final String[] args) {
final Model model = new Model();
final GraphicView view = new GraphicView(model);
final JFrame f = new JFrame("Draw Test");
f.setContentPane(view);
f.setSize(500, 300);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
private final JButton circle = new JButton("Circle");
private final Easel easel;
private final JButton line = new JButton("Line");
private Model model = null;
private final JButton rectangle = new JButton("Rectangle");
/**
* Lays out the view and registers the button listeners.
*
* @param newModel
* the model to view.
*/
public GraphicView(final Model model) {
this.model = model;
this.easel = new Easel(this.model);
this.layoutView();
this.registerListeners();
}
/**
* Lays out the graphic components.
*/
private void layoutView() {
final JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new GridLayout(3, 1));
buttonPanel.add(this.line);
buttonPanel.add(this.circle);
buttonPanel.add(this.rectangle);
this.setLayout(new BorderLayout());
this.add(buttonPanel, BorderLayout.WEST);
this.add(this.easel, BorderLayout.CENTER);
}
/**
* Registers the button listeners.
*/
private void registerListeners() {
this.line.addActionListener(new ShapeListener());
this.circle.addActionListener(new ShapeListener());
this.rectangle.addActionListener(new ShapeListener());
}
}
public class Easel extends JComponent {
/**
* Repaints the easel whenever the model is updated.
*/
private class ModelListener implements PropertyChangeListener {
@Override
public void propertyChange(final PropertyChangeEvent evt) {
Easel.this.repaint();
}
}
private Model model = null;
/**
* The Easel needs direct access to the Model.
*
* @param newModel
* the Model to access.
*/
public Easel(final Model model) {
this.model = model;
this.registerListeners();
}
/**
* Randomly defines a shape based upon the current size of the easel. The
* new shape is passed back to the model to be stored.
*
* @param t
* the type of shape to define.
*/
public void defineShape(final String t) {
final int x1 = (int) (Math.random() * this.getWidth());
final int y1 = (int) (Math.random() * this.getHeight());
final int x2 = (int) (Math.random() * this.getWidth());
final int y2 = (int) (Math.random() * this.getHeight());
if (t.equals("Rectangle")) {
this.model.addShape(new Rectangle(x1, y1, x2, y2));
} else if (t.equals("Line")) {
this.model.addShape(new Line2D.Double(x1, y1, x2, y2));
} else if (t.equals("Circle")) {
this.model.addShape(new Circle(x1, y1, x2));
}
}
/*
* (non-Javadoc)
*
* @see javax.swing.JComponent#paintComponent(java.awt.Graphics)
*/
@Override
public void paintComponent(final Graphics g) {
final Graphics2D g2d = (Graphics2D) g;
// Get a snapshot of the list of shapes in the model. Draw each in turn.
final Iterator i = this.model.getShapesIterator();
while (i.hasNext()) {
g2d.draw(i.next());
}
}
/**
* Registers the model listener.
*/
private void registerListeners() {
this.model.addPropertyChangeListener(new ModelListener());
}
}
class Circle extends Ellipse2D.Double {
public Circle(final double centerX, final double centerY, final double radius) {
// Call the Ellipse constructure with major and minor axes the same
// size.
super(centerX - radius, centerY - radius, 2.0 * radius, 2.0 * radius);
}
}
public class Model {
/**
* A list of Shapes
.
*/
private final ArrayList
/**
* Allows views to listen to generic changes in the model.
*/
private final PropertyChangeSupport pcs = new PropertyChangeSupport(this);
/**
* Adds a new shape to the model.
*
* @param s
* the new shape to add to the list of shapes.
*/
public void addShape(final Shape s) {
this.shapes.add(s);
this.pcs.firePropertyChange(null, true, false);
}
/**
* Attaches listeners to the model.
*
* @param listener
* The listener to attach to the model.
*/
public void addPropertyChangeListener(
final PropertyChangeListener listener) {
this.pcs.addPropertyChangeListener(listener);
}
/**
* Returns an iterator for the model's list of shapes.
*
* @return the iterator for Shapes.
*/
public Iterator
return this.shapes.iterator();
}
}
Fill every shape with a random color when it is displayed Add another button that allows polygons of 3 to 8 sides (choose randomly) to be added to the model and canvas. Add a textfield that allows the user to add text at random positions on the canvas. (Hint:strings are not shapes- you'll need a list of stringsStep by Step Solution
There are 3 Steps involved in it
Step: 1
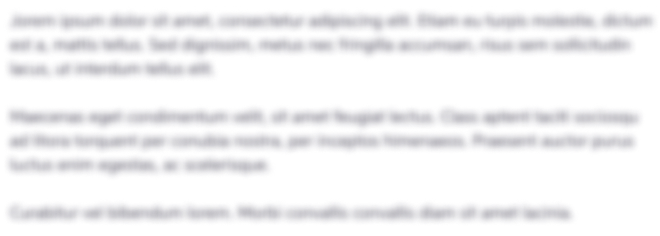
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started