Question
NEED JAVA HELP PLEASE ! Getting Started To begin this lab, download the Eclipse project file here: abc123-lab4.zip This JavaFX project consists of the following,
NEED JAVA HELP PLEASE !
Getting Started
To begin this lab, download the Eclipse project file here: abc123-lab4.zip This JavaFX project consists of the following, and follows the MVC design pattern:
Lab4.java - runs the application
MainController.java - controller
Board.java - model (represents the game board)
Square.java - model (represents a square on the board)
board.fxml - view
Begin by downloading the code and becoming familiar with each of these classes.
If you run the code as given, the following application will be presented:
Each of the gray squares is hiding a color. When one of the gray squares is clicked, it will reveal the color underneath. At this time, this is all the application does. Time for you to implement the game play!
Well use the following terms in game play: A square is revealed when the user clicks on it - the color of the square (red, green, blue, or yellow) is then shown. Squares are gray when hidden.
Rules of the game:
When the user clicks a gray square, its color should be revealed.
If the user clicks a square that is already revealed, the click should be ignored, and the square should not change.
The user is allowed to reveal two squares. If the two colors match, the squares remain revealed and the user gets another turn.
If the user chooses 2 squares that do not match, both of those squares are hidden again.
The game is won when the user has matched all squares, and the board appears as follows:
You may implement game play by adding class variables and/or object methods to the given classes. Any changes must follow the MVC design pattern - for example, any modification to the model should be made in the model classes.
Hints & Examples
There are a few hints and //TODO comments included in the given code. For this assignment, it will not be necessary to alter the fxml file. If you run the application within Eclipse, youll notice information is printed to the console whenever the user clicks on the board. This state information will help you debug as you implement changes to the game.
Here are a few example states the user could find themselves in during game play:
In the following image, the user has chosen two squares and they are matching colors. After this, these two green squares should remain revealed and there user should get the opportunity to choose 2 more squares.
In the following image, the user had previously revealed two matching squares, both were green. These squares remained revealed and the user chose another square, which turned out to be yellow. The user now has an opportunity to choose 1 more square and its color will be compared to the yellow square.
In the following image, the user has chosen two squares and they are not matching colors. After showing the user the two non-matching colors, both squares should be hidden again (turning them gray). Then the user should be allowed to take another turn, choosing 2 more squares.
Extra Help There are many ways to display the color of a square briefly then hide it again. If you're stuck, try out the following code and incorporate it into your solution. Place the sleep method code provided below in the MainController.java class provided.
/** * Sleep method handles waiting for some period of time before changing * the color of the given Button back to default (light gray color). * * @param selected Button clicked by the user * @param seconds Integer value of number of seconds to sleep */ public void sleep( Button selected, int seconds ) { Task sleeper = new Task() { @Override protected Void call() throws Exception { try { Thread.sleep(seconds*1000); } catch (InterruptedException e) { e.printStackTrace(); } return null; } }; EventHandler e = new EventHandler() { @Override public void handle(WorkerStateEvent event) { selected.setStyle(""); } }; sleeper.setOnSucceeded( e ); new Thread(sleeper).start(); }
To call this new method, try this example (within the handle method in MainController).
sleep( selected, 1 );
This example call sends the selected button, provided in the handle method code, to the sleep method and sleeps for 1 second.
Test the code to see how this works!
code provided already:
----------------------------------------------------------
Lab4.java
------------------------------------------------------------
import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.stage.Stage; import javafx.scene.Parent; import javafx.scene.Scene;
public class Lab4 extends Application { @Override public void start(Stage primaryStage) { try { // Load the FXML file for the game board Parent root = FXMLLoader.load(getClass().getResource("/board.fxml")); // Set the scene onto the stage primaryStage.setScene(new Scene(root, 800, 400)); // Display the board to the user primaryStage.show(); } catch(Exception e) { e.printStackTrace(); } } public static void main(String[] args) { // Launches the application (calls start method in the process) launch(args); } }
---------------------------------------------------------------
Maincontroller.java
--------------------------------------------------------------------
import application.model.Board; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.fxml.FXML; import javafx.scene.control.Button;
public class MainController implements EventHandler
@FXML Button zero0, zero1, zero2, zero3, one0, one1, one2, one3; private Board gameBoard = new Board();
@Override public void handle(ActionEvent event) { Button selected = (Button) event.getSource(); System.out.println("User selected square: " + selected.toString()); String color = gameBoard.reveal(selected.getId()); if( color!=null ) selected.setStyle("-fx-background-color: #" + color ); System.out.println( gameBoard ); } }
------------------------------------------------------------
Borad.java
----------------------------------------------------------------------
import javafx.scene.paint.Color;
public class Board { private Square[][] squares; public Board() { this.squares = new Square[2][4]; squares[0][0] = new Square(Color.RED); squares[0][1] = new Square(Color.BLUE); squares[0][2] = new Square(Color.GREEN); squares[0][3] = new Square(Color.YELLOW); squares[1][0] = new Square(Color.GREEN); squares[1][1] = new Square(Color.RED); squares[1][2] = new Square(Color.YELLOW); squares[1][3] = new Square(Color.BLUE); } public void hide( int x, int y ) { // TODO: Hide the given square // Hint: call the corresponding method in the Square class to help you hide it. } public void hideBoth() { // TODO: Hide both squares - the user's 1st & 2nd choices this round // Hint: this should be called if no match is found // Hint 2: this method should call a hide method twice, one for each square. } public boolean matchFound() { // TODO: Check to see if a match is found, and return true if so return false; } public String reveal( String buttonName ) { int x = buttonName.startsWith("zero") ? 0 : 1; int y; if( buttonName.endsWith("zero") ) y = 0; else if( buttonName.endsWith("one") ) y = 1; else if( buttonName.endsWith("two") ) y = 2; else y = 3; return this.reveal(x,y); } public String reveal( int x, int y ) { squares[x][y].reveal(); return squares[x][y].getColorAsCode(); } /** * ToString method returns a String representation of the current state of the game board. * * @return String Board colors currently revealed. */ public String toString() { String ret = squares[0][0] + ", " + squares[0][1] + ", "; ret += squares[0][2] + ", " + squares[0][3] + " "; ret += squares[1][0] + ", " + squares[1][1] + ", "; ret += squares[1][2] + ", " + squares[1][3]; return ret; } }
-----------------------------------------------------------
Square.java
---------------------------------------------------------------
import javafx.scene.paint.Color;
public class Square {
private boolean isDisplayed; private Color color; private static final String BLUE = "0000FF"; private static final String GREEN = "00FF00"; private static final String RED = "FF0000"; private static final String YELLOW = "FFFF00"; public Square( Color c ) { this.color = c; this.isDisplayed = false; } public Color getDisplayedColor() { if( this.isDisplayed() ) return this.getColor(); else return Color.GRAY; } public Color getColor() { return this.color; } public boolean isDisplayed() { return isDisplayed; } public void hide() { this.isDisplayed = false; } public void reveal() { this.isDisplayed = true; } public String getColorAsCode() { if( !isDisplayed() ) return Color.GRAY.toString(); if( color.equals(Color.RED) ) return RED; else if( color.equals(Color.GREEN) ) return GREEN; else if( color.equals(Color.BLUE) ) return BLUE; else return YELLOW; } public String getColorAsString() { if( !isDisplayed() ) return "GRAY"; if( color.equals(Color.YELLOW) ) return "YELLOW"; else if( color.equals(Color.GREEN) ) return "GREEN"; else if( color.equals(Color.BLUE) ) return "BLUE"; else if( color.equals(Color.RED) ) return "RED"; else return "GRAY"; } public String toString() { return this.getColorAsString(); } }
If you run the code as given, the following application will be presented If you run the code as given, the following application will be presented
Step by Step Solution
There are 3 Steps involved in it
Step: 1
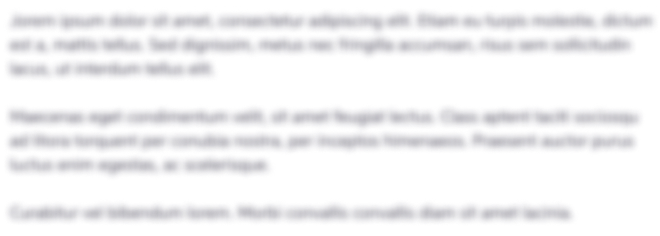
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started