Question
Need to be answered in Java, thanks a lot. Create, save and compile the following interface definition in a file called Carryable.java: public interface Carryable
Need to be answered in Java, thanks a lot.
Create, save and compile the following interface definition in a file called Carryable.java:
public interface Carryable {
public String getContents();
public String getDescription();
public float getPrice();
}
Starting with your GroceryItem code from the previous assignment, make adjustments so that the class implements the Carryable interface. You will need to write a getContents(), getDescription() and getPrice() method. By default, the contents should return an empty String. The description should be the item name. The price should be the item price.
Have the GroceryBag class also implement this interface. The getContents() method should return a line-by-line inventory of items in the bag as shown here, for example. Note that each line begins with three spaces (provides indentation when printed):
" Smart-Ones Frozen Entrees weighing 0.311 kg with price $1.99
SnackPack Pudding weighing 0.396 kg with price $0.99
Breyers Chocolate Icecream weighing 2.27 kg with price $2.99
Nabob Coffee weighing 0.326 kg with price $3.99
Gold Seal Salmon weighing 0.213 kg with price $1.99"
The getDescription() method should return "GROCERY BAG (xxx kg)" where xxx is the weight of the bag. The getPrice() method should return the total price of all items in the bag.
In the Shopper class, change the type of the cart attribute to be Carryable instead of GroceryItem. This means that the shopping cart will now be allowed to contain both GroceryItem and GroceryBag objects. Properly make any needed changes to the class so that it will compile (do not add additional attributes). You will likely need to use typecasting somewhere.
Create a subclass of GroceryItem called PerishableItem that represents an item that can spoil. Implement a public toString() method that returns a String representation of the PerishableItem as follows (making sure to make full use of inheritance):
Smart-Ones Frozen Entrees weighing 0.311kg with price $1.99 (perishable)
Remove the perishable attribute from the GroceryItem class. You will need to modify the constructors (and delete one of them) as well as remove the get method.
Adjust the ShopperTestProgram (from the last assignment) to make use of the PerishableItem class now for all items that were perishable. You will see a need to create a constructor in the PerishableItem class that makes full use of inheritance.
Adjust your unpackPerishables() method in the GroceryBag class so that it works properly again by making proper use of inheritance. The method MUST return an array of PerishableItem objects now instead of GroceryItem objects.
Create two subclasses of PerishableItem called RefigeratorItem and FreezerItem. Make PerishableItem to be an abstract class. Add any needed constructors to these new classes. Create toString() methods that make proper use of inheritance to display these items with additional instructions as shown below:
Smart-Ones Frozen Entrees weighing 0.311kg with price $1.99 (perishable) [keep frozen] Sealtest Milk weighing 2.06kg with price $2.99 (perishable) [keep refrigerated]
Adjust the ShopperTestProgram so that frozen items (i.e., all perishable items except for the ground beef) are FreezerItem objects. The ground beef should be a RefrigeratorItem along with these, which you should add to the test program:
g12 = new RefrigeratorItem("2L Sealtest Milk",2.99f,2.06f);
g13 = new RefrigeratorItem("Extra-Large Eggs",1.79f,0.77f);
g14 = new RefrigeratorItem("Yoplait Yogurt 6-pack",4.74f,1.02f);
Make sure to add one of each of these to the cart, after the last g5 item is added.
We will now make the code more realistic by allowing the GroceryBag objects to be placed back into the shopping cart. Adjust the addItem() and removeItem() methods in the Shopper class to allow GroceryBag objects to be added to (and removed from) the cart, along with GroceryItem objects.
Modify the packBags() method so that it has a void return type. Instead of returning an array of GroceryBag objects, the method returns nothing. However, the method should ensure that, before returning, all packed GroceryBag objects are added to the cart.
Write a method in the Shopper class called displayCartContents() that displays the description and contents of the Shopper's cart nicely with proper indentation as shown below (although your output may vary). Remember, there are GroceryItems and GroceryBags in the cart now. You ARE NOT allowed to use instanceof in your solution, and you cannot use the getClass() method.
Toilet Paper - 48 pack
Coca-Cola 12-pack
Coca-Cola 12-pack
GROCERY BAG (4.3739996kg)
Smart-Ones Frozen Entrees weighing 0.311kg with price $1.99 (perishable)[keep frozen]
Yoplait Yogurt 6-pack weighing 1.02kg with price $4.74 (perishable)[keep refrigerated]
Extra-Large Eggs weighing 0.77kg with price $1.79 (perishable)[keep refrigerated]
2L Sealtest Milk weighing 2.06kg with price $2.99 (perishable)[keep refrigerated]
Gold Seal Salmon weighing 0.213kg with price $1.99
GROCERY BAG (4.987kg)
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Heinz Beans Original weighing 0.477kg with price $0.79
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
GROCERY BAG (3.3049998kg)
Gold Seal Salmon weighing 0.213kg with price $1.99
5-Alive Frozen Juice weighing 0.426kg with price $0.75 (perishable)[keep frozen]
SnackPack Pudding weighing 0.396kg with price $0.99
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
GROCERY BAG (4.54kg)
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
GROCERY BAG (4.54kg)
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
GROCERY BAG (3.0619998kg)
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
SnackPack Pudding weighing 0.396kg with price $0.99
SnackPack Pudding weighing 0.396kg with price $0.99
GROCERY BAG (4.55kg)
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
Smart-Ones Frozen Entrees weighing 0.311kg with price $1.99 (perishable)[keep frozen]
Nabob Coffee weighing 0.326kg with price $3.99
5-Alive Frozen Juice weighing 0.426kg with price $0.75 (perishable)[keep frozen]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Heinz Beans Original weighing 0.477kg with price $0.79
GROCERY BAG (2.473kg)
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
Gold Seal Salmon weighing 0.213kg with price $1.99
Alter the unpackPerishables() method in the GroceryBag class to return PerishableItem objects instead of GroceryItem objects.
Write a removePerishables() method in the Shopper class that removes all PerishableItem objects from the cart. It should return an array of all PerishableItem objects that have been removed from the GroceryBags as well as any that were not packed in bags.
Modify the code near the end of the ShopperTestProgram so that it calls removePerishables() in the Shopper class and displays those unpacked items. Also, replace this code:
System.out.println(" REMAINING BAG CONTENTS:");
for (int i=0; i
System.out.println(" BAG " + (i+1) + " (Total Weight = " + packedBags[i].getWeight() + "kg) CONTENTS:");
for (int j=0; j
System.out.println(" " + packedBags[i].getItems()[j]);
}
}
with the following:
System.out.println(" REMAINING CART CONTENTS:");
c.displayCartContents();
Add this item to the cart (after g14 was added):
g15 = new FreezerItem("Mega-Sized Chocolate Icecream",67.93f,15.03f);
Test everything by running the code. You should see the following output:
INITIAL CART CONTENTS:
Smart-Ones Frozen Entrees weighing 0.311kg with price $1.99 (perishable)[keep frozen]
SnackPack Pudding weighing 0.396kg with price $0.99
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Nabob Coffee weighing 0.326kg with price $3.99
Gold Seal Salmon weighing 0.213kg with price $1.99
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
Heinz Beans Original weighing 0.477kg with price $0.79
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
5-Alive Frozen Juice weighing 0.426kg with price $0.75 (perishable)[keep frozen]
Coca-Cola 12-pack weighing 5.112kg with price $3.49
Smart-Ones Frozen Entrees weighing 0.311kg with price $1.99 (perishable)[keep frozen]
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
SnackPack Pudding weighing 0.396kg with price $0.99
SnackPack Pudding weighing 0.396kg with price $0.99
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Coca-Cola 12-pack weighing 5.112kg with price $3.49
Toilet Paper - 48 pack weighing 10.89kg with price $40.96
5-Alive Frozen Juice weighing 0.426kg with price $0.75 (perishable)[keep frozen]
Gold Seal Salmon weighing 0.213kg with price $1.99
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
Heinz Beans Original weighing 0.477kg with price $0.79
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Gold Seal Salmon weighing 0.213kg with price $1.99
2L Sealtest Milk weighing 2.06kg with price $2.99 (perishable)[keep refrigerated]
Extra-Large Eggs weighing 0.77kg with price $1.79 (perishable)[keep refrigerated]
Yoplait Yogurt 6-pack weighing 1.02kg with price $4.74 (perishable)[keep refrigerated]
Mega-Sized Chocolate Icecream weighing 15.03kg with price $67.93 (perishable)[keep frozen]
CART CONTENTS:
Mega-Sized Chocolate Icecream
Toilet Paper - 48 pack
Coca-Cola 12-pack
Coca-Cola 12-pack
GROCERY BAG (4.77kg)
Smart-Ones Frozen Entrees weighing 0.311kg with price $1.99 (perishable)[keep frozen]
SnackPack Pudding weighing 0.396kg with price $0.99
Yoplait Yogurt 6-pack weighing 1.02kg with price $4.74 (perishable)[keep refrigerated]
Extra-Large Eggs weighing 0.77kg with price $1.79 (perishable)[keep refrigerated]
2L Sealtest Milk weighing 2.06kg with price $2.99 (perishable)[keep refrigerated]
Gold Seal Salmon weighing 0.213kg with price $1.99 GROCERY BAG (4.987kg)
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Heinz Beans Original weighing 0.477kg with price $0.79
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
GROCERY BAG (3.235kg)
Gold Seal Salmon weighing 0.213kg with price $1.99
5-Alive Frozen Juice weighing 0.426kg with price $0.75 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Nabob Coffee weighing 0.326kg with price $3.99
GROCERY BAG (4.54kg)
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
GROCERY BAG (4.54kg)
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
GROCERY BAG (3.0619998kg)
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
SnackPack Pudding weighing 0.396kg with price $0.99
SnackPack Pudding weighing 0.396kg with price $0.99
GROCERY BAG (4.4370003kg)
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
Smart-Ones Frozen Entrees weighing 0.311kg with price $1.99 (perishable)[keep frozen]
Gold Seal Salmon weighing 0.213kg with price $1.99
5-Alive Frozen Juice weighing 0.426kg with price $0.75 (perishable)[keep frozen]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Heinz Beans Original weighing 0.477kg with price $0.79
GROCERY BAG (2.26kg)
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
REMAINING CART CONTENTS:
Mega-Sized Chocolate Icecream weighing 15.03kg with price $67.93 (perishable)[keep frozen]
Toilet Paper - 48 pack weighing 10.89kg with price $40.96
Coca-Cola 12-pack weighing 5.112kg with price $3.49
Coca-Cola 12-pack weighing 5.112kg with price $3.49
A 4.77kg grocery bag with 6 items
A 4.987kg grocery bag with 5 items
A 3.235kg grocery bag with 4 items
A 4.54kg grocery bag with 2 items
A 4.54kg grocery bag with 2 items
A 3.0619998kg grocery bag with 3 items
A 4.4370003kg grocery bag with 6 items
A 2.26kg grocery bag with 1 items
UNPACKING PERISHABLES:
Mega-Sized Chocolate Icecream weighing 15.03kg with price $67.93 (perishable)[keep frozen]
Smart-Ones Frozen Entrees weighing 0.311kg with price $1.99 (perishable)[keep frozen]
Yoplait Yogurt 6-pack weighing 1.02kg with price $4.74 (perishable)[keep refrigerated]
2L Sealtest Milk weighing 2.06kg with price $2.99 (perishable)[keep refrigerated]
Extra-Large Eggs weighing 0.77kg with price $1.79 (perishable)[keep refrigerated]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
5-Alive Frozen Juice weighing 0.426kg with price $0.75 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Breyers Chocolate Icecream weighing 2.27kg with price $2.99 (perishable)[keep frozen]
Smart-Ones Frozen Entrees weighing 0.311kg with price $1.99 (perishable)[keep frozen]
5-Alive Frozen Juice weighing 0.426kg with price $0.75 (perishable)[keep frozen]
Lean Ground Beef weighing 0.75kg with price $4.94 (perishable)[keep refrigerated]
REMAINING CART CONTENTS:
GROCERY BAG (2.26kg)
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
Toilet Paper - 48 pack
Coca-Cola 12-pack
Coca-Cola 12-pack
GROCERY BAG (0.6090002kg)
Gold Seal Salmon weighing 0.213kg with price $1.99
SnackPack Pudding weighing 0.396kg with price $0.99
GROCERY BAG (2.737kg)
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
Heinz Beans Original weighing 0.477kg with price $0.79
GROCERY BAG (0.53900003kg)
Gold Seal Salmon weighing 0.213kg with price $1.99
Nabob Coffee weighing 0.326kg with price $3.99
GROCERY BAG (0.0kg)
GROCERY BAG (0.0kg)
GROCERY BAG (0.7919998kg)
SnackPack Pudding weighing 0.396kg with price $0.99
SnackPack Pudding weighing 0.396kg with price $0.99
GROCERY BAG (2.9500003kg)
Ocean Spray Cranberry Cocktail weighing 2.26kg with price $2.99
Heinz Beans Original weighing 0.477kg with price $0.79
Gold Seal Salmon weighing 0.213kg with price $1.99
Create a computeFreezerItemCost() method in the Shopper class that will return the total price of all FreezerItems in the cart. Note that some items will be packed and some will be loose in the cart.
Create a computeTotalCost() method in the Shopper class that will return the total price of all items in the cart. Note that some items will be packed and some will be loose in the cart. Your code must not use instanceof nor getClass() for this method.
Insert the following code into the ShopperTestProgram after the bags are packed but before the unperishables are unpacked:
System.out.println(" TOTAL FREEZER ITEM COST: $" + c.computeFreezerItemCost());
System.out.println(" TOTAL CART CONTENTS COST: $" + c.computeTotalCost());
The code should produce an answers of $91.35 and $192.05 when you run it.
Here is the code from last assignment.
public class GroceryBag { public static final float MAX_WEIGHT = 5; // max weight allowed (kg) public static final int MAX_ITEMS = 25; // max # items allowed private GroceryItem[] items; // actual GroceryItems in bag private int numItems; // # of GroceryItems in bag private float weight; // current weight of bag public GroceryBag() { items = new GroceryItem[MAX_ITEMS]; numItems = 0; weight = 0; } public GroceryItem[] getItems() { return items; } public int getNumItems() { return numItems; } public float getWeight() { return weight; } public String toString() { if (weight == 0) return "An empty grocery bag"; return ("A " + weight + "kg grocery bag with " + numItems + " items"); } public boolean canHold(GroceryItem g) { return (((weight + g.getWeight()) <= MAX_WEIGHT) && (numItems <= MAX_ITEMS)); } public void addItem(GroceryItem g) { if (canHold(g)) { items[numItems++] = g; weight += g.getWeight(); } } public void removeItem(GroceryItem item) { for (int i = 0; i < numItems; i++) { if (items[i] == item) { weight -= items[i].getWeight(); items[i] = items[numItems - 1]; numItems -= 1; return; } } } // Finds and returns the heaviest item in the shopping cart public GroceryItem heaviestItem() { if (numItems == 0) return null; GroceryItem heaviest = items[0]; for (int i=0; iif (items[i].getWeight() > heaviest.getWeight()) { heaviest = items[i]; } } return heaviest; } // Determines whether or not the given item in the shopping cart public boolean has(GroceryItem item) { for (int i = 0; i < numItems; i++) { if (items[i] == item) { return true; } } return false; } // Remove all perishables from the bag and return an array of them public GroceryItem[] unpackPerishables() { int perishableCount = 0; for (int i=0; iif (items[i].isPerishable()) perishableCount++; } GroceryItem[] perishables = new GroceryItem[perishableCount]; perishableCount = 0; for (int i=0; iif (items[i].isPerishable()) { perishables[perishableCount++] = items[i]; removeItem(items[i]); i--; } } return perishables; } }
public class GroceryItem { private String name; private float price; private float weight; private boolean perishable; public GroceryItem() { name = "?"; price = 0; weight = 0; perishable = false; } public GroceryItem(String n, float p, float w) { name = n; price = p; weight = w; perishable = false; } public GroceryItem(String n, float p, float w, boolean s) { name = n; price = p; weight = w; perishable = s; } public String getName() { return name; } public float getPrice() { return price; } public float getWeight() { return weight; } public boolean isPerishable() { return perishable; } public String toString () { return name + " weighing " + weight + "kg with price $" + price; } }
public class Shopper { public static final int MAX_CART_ITEMS = 100; // max # items allowed private GroceryItem[] cart; // items to be purchased private int numItems; // #items to be purchased public Shopper() { cart = new GroceryItem[MAX_CART_ITEMS]; numItems = 0; } public GroceryItem[] getCart() { return cart; } public int getNumItems() { return numItems; } public String toString() { return "Shopper with shopping cart containing " + numItems + " items"; } // Return the total cost of the items in the cart public float totalCost() { float total = 0; for (int i=0; ireturn total; } // Add an item to the shopper's shopping cart public void addItem(GroceryItem g) { if (numItems < MAX_CART_ITEMS) cart[numItems++] = g; } // Removes the given item from the shopping cart public void removeItem(GroceryItem g) { for (int i=0; iif (cart[i] == g) { cart[i] = cart[numItems - 1]; numItems -= 1; return; } } } // Go through the shopping cart and pack all packable items into bags public GroceryBag[] packBags() { GroceryBag[] packedBags = new GroceryBag[numItems]; int bagCount = 0; GroceryBag currentBag = new GroceryBag(); for (int i=0; iif (item.getWeight() <= GroceryBag.MAX_WEIGHT) { if (!currentBag.canHold(item)) { packedBags[bagCount++] = currentBag; currentBag = new GroceryBag(); } currentBag.addItem(item); removeItem(item); i--; } } // Check this in case there were no bagged items if (currentBag.getWeight() > 0) packedBags[bagCount++] = currentBag; // Now create a new bag array which is just the right size GroceryBag[] result = new GroceryBag[bagCount]; for (int i=0; ireturn result; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
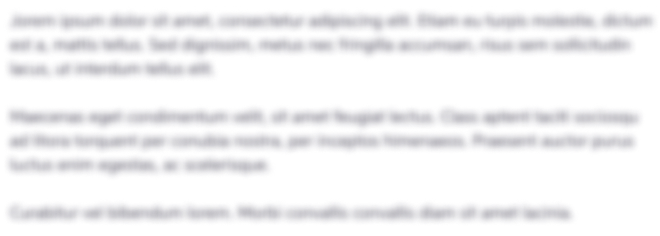
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started