Question
Need Vector.cpp code for this code: Main.cpp #include #include #include Matrix.h #include Vector.h #include Vector3D.h using namespace std; using namespace UT_315; void print_menu(); void matrix_addition();
Need Vector.cpp code for this code:
Main.cpp
#include
using namespace std; using namespace UT_315;
void print_menu(); void matrix_addition(); void matrix_substraction(); void matrix_multiplication(); void matrix_scalar_mult(); void matrix_transpose(); void vector_length(); void vector_inner_prod(); void vector_cross_prod(); void vector_angle();
int main(){ int opt = 0; cout << "Welcome to the Matrix Test Program" << endl; do{ print_menu(); cout << "Enter option: "; cin >> opt; cout << "Option entered: " << opt << endl; switch(opt){ case 1: matrix_addition(); break; case 2: matrix_substraction(); break; case 3: matrix_multiplication(); break; case 4: matrix_scalar_mult(); break; case 5: matrix_transpose(); break; case 6: vector_length(); break; case 7: vector_inner_prod(); break; case 8: vector_cross_prod(); break; case 9: vector_angle(); break; case 10: opt = -1; break; default: cout << "Illegal option: " << opt << endl; } } while(opt != -1); cout << "Thanks for using the Matrix Test Program." << endl; exit(0); }
void print_menu(){ cout << "***************************" << endl; cout << "Available Operations:"<< endl; cout << "\t 1. Matrix Addition" << endl; cout << "\t 2. Matrix Substraction" << endl; cout << "\t 3. Matrix Multiplication" << endl; cout << "\t 4. Matrix Scalar Multiplication" << endl; cout << "\t 5. Matrix Transpose" << endl; cout << "\t 6. Vector lenght" << endl; cout << "\t 7. Vector Inner (dot) Product" << endl; cout << "\t 8. Vector Cross Product" << endl; cout << "\t 9. Vector angle " << endl; cout << "\t 10. Exit" << endl; }
void matrix_addition(){ Matrix M1, M2, M3; cout << "Enter the first matrix: " << endl; cin >> M1; cout << "Enter the second matrix: " << endl; cin >> M2; cout << "Result: " << endl; cout << M1; cout << "\t + " << endl; cout << M2; cout << "\t = " << endl; M3 = M1 + M2; cout << M3; }
void matrix_substraction(){ Matrix M1, M2, M3; cout << "Enter the first matrix: " << endl; cin >> M1; cout << "Enter the second matrix: " << endl; cin >> M2; cout << "Result: " << endl; cout << M1; cout << "\t - " << endl; cout << M2; cout << "\t = " << endl; M3 = M1 - M2; cout << M3; }
void matrix_multiplication(){ Matrix M1, M2, M3; cout << "Enter the first matrix: " << endl; cin >> M1; cout << "Enter the second matrix: " << endl; cin >> M2; cout << "Result: " << endl; cout << M1; cout << "\t * " << endl; cout << M2; cout << "\t = " << endl; M3 = M1 * M2; cout << M3; }
void matrix_scalar_mult(){ Matrix M1; int scalar = 0; cout << "Enter the matrix: " << endl; cin >> M1; cout << "Enter the scalar value: " << endl; cin >> scalar; cout << "Result: " << endl; cout << M1; cout << "\t * " << endl; cout << scalar << endl; cout << "\t = " << endl; M1*=scalar; cout << M1; }
void matrix_transpose(){ Matrix M1, M2; cout << "Enter the matrix: " << endl; cin >> M1; cout << "The tranpose of matrix: " << endl; cout << M1; cout << "is the matrix: " << endl; M2 = M1.transpose(); cout << M2; }
void vector_length(){ Vector V1; cout << "Enter the vector: " << endl; cin >> V1; cout << "The length of the vector: " << endl; cout << V1; cout << "is: " << V1.length() << endl; }
void vector_inner_prod(){ Vector V1, V2; double result = 0.0; cout << "Enter the first vector: " << endl; cin >> V1; cout << "Enter the second vector: " << endl; cin >> V2; cout << "Result: " << endl; cout << V1; cout << " | " << endl; cout << V2; cout << " = "; result = V1 | V2; cout << result << endl; }
void vector_cross_prod(){ Vector3D V1, V2, V3; cout << "Enter the first vector: " << endl; cin >> V1; cout << "Enter the second vector: " << endl; cin >> V2; cout << "Result: " << endl; cout << V1; cout << " & " << endl; cout << V2; cout << " = " << endl; V3 = V1 & V2; cout << V3; }
void vector_angle(){ Vector V1, V2; double result = 0.0;
cout << "Enter the first vector: " << endl; cin >> V1; cout << "Enter the second vector: " << endl; cin >> V2; cout << "The angle between: " << endl; cout << V1; cout << " and " << endl; cout << V2; cout << " is " << endl; result = V1.angle(V2); cout << result << endl; }
Matrix.cpp
#include "Matrix.h"
namespace UT_315 {
/////////////////// DO NOT MODIFY THESE TWO FUNCTIONS/////////////
// Output operator (Friend function)
ostream& operator<<(ostream &out, const Matrix& M) {
int i = 0, j = 0, len1 = 0, len2 = 0;
len1 = M.get_rows();
len2 = M.get_cols();
for (i = 0; i < len1; ++i) {
for (j = 0; j < len2; ++j) {
out << M.get_value(i, j);
if (j != len2 - 1) {
out << " ";
}
}
out << " ";
}
return out;
}
// Input operator (Friend function)
istream& operator>>(istream &in, Matrix& M) {
int i = 0, j = 0, rows = 0, cols = 0;
int new_val;
in >> rows;
in >> cols;
M = Matrix(rows, cols);
for (i = 0; i < rows; ++i) {
for (j = 0; j < cols; ++j) {
in >> new_val;
M.set_value(i, j, new_val);
}
}
return in;
}
///////////////////////////////////////////////////////////////
// ADD YOUR CODE HERE
Matrix::Matrix(size_type rows, size_type cols)
{
set_rows(rows);
set_cols(cols);
}
void Matrix::set_rows(size_type newrow)
{
curr_rows = newrow;
}
void Matrix::set_cols(size_type newcolumn)
{
curr_cols = newcolumn;
}
bool Matrix::operator==(const Matrix & M) const
{
return false;
}
const Matrix & Matrix::operator+=(const Matrix & M)
{
return M;
}
const Matrix & Matrix::operator-=(const Matrix & M)
{
return M;
}
const Matrix & Matrix::operator*=(const Matrix & M)
{
return M;
}
Matrix::value_type Matrix::get_value(Matrix::size_type a, Matrix::size_type b)const
{
return elements[a][b];
}
void Matrix::set_value(Matrix::size_type a, Matrix::size_type b, Matrix::value_type c)
{
elements[a][b] = c;
}
Matrix::size_type Matrix::get_rows() const
{
return curr_rows;
}
Matrix::size_type Matrix::get_cols() const
{
return curr_cols;
}
Matrix operator*(const Matrix& M1, const Matrix& M2)
{
Matrix mult;
int k;
int res = 0;
if (M1.get_cols() == M2.get_rows())
{
mult = Matrix(M1.get_rows(), M2.get_cols());
for (Matrix::size_type i = 0; i < M1.get_rows(); ++i)
{
for (Matrix::size_type j = 0; j < M2.get_cols(); ++j)
{
for (k = 0; k < M2.get_rows(); ++k) {
res += M1.get_value(i, k) * M2.get_value(k, j);
}
mult.set_value(i, j, res);
res = 0;
}
}
}
else
{
cout << "Error, first matrix MUST have same amount of columns as second matrix rows." << endl;
}
return mult;
}
Matrix operator+(const Matrix& M1, const Matrix& M2)
{
Matrix sum;
if (M1.get_rows() == M2.get_rows() && M1.get_cols() == M2.get_cols())
{
sum = Matrix(M1.get_rows(), M1.get_cols());
for (Matrix::size_type i = 0; i < M1.get_rows(); ++i)
{
for (Matrix::size_type j = 0; j < M1.get_cols(); ++j)
{
sum.set_value(i, j, M1.get_value(i, j) + M2.get_value(i, j));
}
}
}
else
{
cout << "Error, to sum two matrix, both must be dimensionally equal.";
}
return sum;
}
Matrix operator-(const Matrix& M1, const Matrix& M2)
{
Matrix sub;
if (M1.get_rows() == M2.get_rows() && M1.get_cols() == M2.get_cols())
{
sub = Matrix(M1.get_rows(), M1.get_cols());
for (Matrix::size_type i = 0; i < M1.get_rows(); ++i)
{
for (Matrix::size_type j = 0; j < M1.get_cols(); ++j)
{
sub.set_value(i, j, M1.get_value(i, j) - M2.get_value(i, j));
}
}
}
else
{
cout << "Error, to substract two matrix, both must be dimensionally equal.";
}
return sub;
}
const Matrix& Matrix :: operator*=(int r)
{
for (size_t i = 0; i < get_rows(); ++i)
{
for (size_t j = 0; j < get_cols(); ++j)
{
set_value(i, j, r*get_value(i, j));
}
}
return *this;
}
Matrix Matrix::transpose() const
{
Matrix trans;
trans = *(new Matrix(get_cols(), get_rows()));
for (size_t i = 0; i < get_rows(); ++i)
{
for (size_t j = 0; j < get_cols(); ++j)
{
trans.set_value(j, i, get_value(i, j));
}
}
return trans;
}
}
Vector.h
#ifndef _VECTOR_ND_H #define _VECTOR_ND_H
#include
namespace UT_315{ class Vector : public Matrix { public: // Constructor Vector(int dimensions = 3);
// Return number of dimensions size_type get_dimensions() const {return this->get_rows();} // Returns a given coordinate value_type get_coord(int coord) const {return this->get_value(coord,0);} // Sets a given coordinate void set_coord(int coord, value_type new_val) {this->set_value(coord, 0, new_val);} // Vector Magnitude double length() const; // Vector Inner Product double operator|(const Vector& V) const;
// Angle between vectors double angle(const Vector& V) const; };
} #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
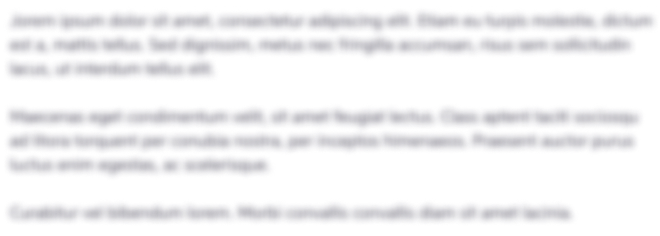
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started