Question
NewCar.java class coding here: public class NewCar { String year; String make; String model; double price; double monthPay; String desc; String abb; NewCar(String year, String
NewCar.java class coding here:
public class NewCar { String year; String make; String model; double price; double monthPay; String desc; String abb; NewCar(String year, String make, String model) { this.year = year; this.make = make; this.model = model; this.price = 0; this.monthPay = 0; this.desc = this.year+" "+make+" "+ model; this.abb = this.year.substring(2,4)+make.charAt(0)+model.charAt(0); } double calFinalPrice(double sPrice, double discount, double salesTax) { double temp = sPrice-discount; double tax = (double)(temp*salesTax)/100; this.price = temp+tax; return this.price; } double calZeroPctMonPayt(int month) { this.monthPay = (double)this.price/month; return this.monthPay; } public String toString() { String des = this.desc ; String ab = this.abb; return "You want to purchase a " + des + " Abbreveiation: " + ab; } }
NewCarTester.java coding here:
import java.util.Scanner;
public class NewCarTester { public static void main(String[] args) { Scanner s = new Scanner(System.in); System.out.print("Enter the car's Year, Make and Model: "); String desc = s.nextLine(); String[] words = desc.split("\\s"); String year = words[0]; String make = words[1]; String model = words[2]; System.out.print("Enter the sticker price: "); double price = s.nextDouble(); System.out.print("Enter the discount: "); double discount = s.nextDouble(); System.out.print("Enter the Sales Tax Rate: "); double rate = s.nextDouble(); System.out.print("Enter the Number of Months at Zero Percent Interest Rate: "); int months = s.nextInt(); NewCar obj = new NewCar(year,make,model); double totalPrice = obj.calFinalPrice(price,discount,rate); double monthlyPrice = obj.calZeroPctMonPayt(months); System.out.println(); System.out.println(obj.toString()); System.out.println("Final Price: "+String.format("%.2f",totalPrice)); System.out.println("Monthly Payment at Zero Percent: "+String.format("%.2f",monthlyPrice)); } }
PROBLEM 1 - Arrays (Note: There are two separate problems in this HW.) Write a Java application to simulate coin tosses and compute the fraction of heads and fraction of tails. As a challenge, determine the longest run of one outcome; that is, the most consecutive heads or consecutive tails (which ever is longer) To simulate the toss of a coin, use the random method in the Math utility class, that generates a number greater than or equal to 0 and less than 1 . O. The notation for this range is [0 , 1.0), where the square bracket means to include the 0 in the range and the rounded parenthesis means to exclude the 1.0 from the range. Since there are two outcomes of equal probability in the toss of a "fair" coin, divide the range of numbers between [0 ,1.0) into two roughly equal size sub-ranges. Assume any number less than 0.5 will be considered as a tail while any number greater than or equal to 0.5 as a head Program Organization Requirements: CoinToss.iava A parameterized constructor that accepts an integer parameter for the number of tosses. This value will be used to set the size of an array that will hold the coin toss results. A loop is used to fill an array with the characters 'h' (head) and t' (tail). As described above, use a random number to determine if each "coin toss" is a head or a tail. This array initialization is accomplished in the constructor (HINT you can keep a count of the number of heads and tails as attributes as you fill the array) Create methods that return the number of heads and the number of tails in the coin toss array (these are accessors) Create a method that returns the total number of coin tosses. Create a method that computes the longest (consecutive) number of heads or tails in the coin tosses array. This method stores this information in two attributes: one for the length of the longest run, and one for the outcome of that . PROBLEM 1 - Arrays (Note: There are two separate problems in this HW.) Write a Java application to simulate coin tosses and compute the fraction of heads and fraction of tails. As a challenge, determine the longest run of one outcome; that is, the most consecutive heads or consecutive tails (which ever is longer) To simulate the toss of a coin, use the random method in the Math utility class, that generates a number greater than or equal to 0 and less than 1 . O. The notation for this range is [0 , 1.0), where the square bracket means to include the 0 in the range and the rounded parenthesis means to exclude the 1.0 from the range. Since there are two outcomes of equal probability in the toss of a "fair" coin, divide the range of numbers between [0 ,1.0) into two roughly equal size sub-ranges. Assume any number less than 0.5 will be considered as a tail while any number greater than or equal to 0.5 as a head Program Organization Requirements: CoinToss.iava A parameterized constructor that accepts an integer parameter for the number of tosses. This value will be used to set the size of an array that will hold the coin toss results. A loop is used to fill an array with the characters 'h' (head) and t' (tail). As described above, use a random number to determine if each "coin toss" is a head or a tail. This array initialization is accomplished in the constructor (HINT you can keep a count of the number of heads and tails as attributes as you fill the array) Create methods that return the number of heads and the number of tails in the coin toss array (these are accessors) Create a method that returns the total number of coin tosses. Create a method that computes the longest (consecutive) number of heads or tails in the coin tosses array. This method stores this information in two attributes: one for the length of the longest run, and one for the outcome of thatStep by Step Solution
There are 3 Steps involved in it
Step: 1
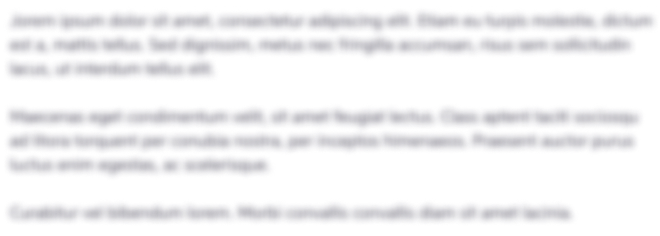
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started