Question
Note that the main function that I have provided does use as it constructs test strings to pass to your functions. However, your solutions for
Note that the main function that I have provided does use
-
Write a function called strcmp373. This function is passed two parameters, both of which are C strings. You should use array syntax when writing this function; that is, you may use [ ], but not * or &. The function should return an int as follows:
-
A negative number if the first string is alphabetically before the second string. In C, the return value of strcmp is a number which reflects the difference in the ASCII codes for the first 2 letters which are not the same. For example, a call to strcmp("programming", "project") returns -3, since 'g' - 'j' in ASCII is -3.
-
Zero if the strings are the same
-
A positive number if the second string is alphabetically before the first
string. Again, the number is the difference in ASCII codes between the first 2 letters which are not the same.
Here are some examples of how your code should work:
strcmp373("bin", "bag"); // returns 8 strcmp373("computer", "game"); // returns -4 strcmp373("computer", "computer"); // returns 0 strcmp373("are", "area"); // returns -97, because '\0'- a = 0-97 = - 97.
-
-
Write a function called strcat373. The function is passed two parameters, both of which are C strings. You should use pointer syntax when writing this function (in other words, you can use * and &, but not [ ]). The function should modify the first parameter (a char array, often called the destination array) so that it contains the concatenation of the two strings, and returns a pointer to the destination array. For example:
int main() { char str1[9] = "comp"; char str2[ ] = "uter"; char *str3; strcat373(str1, str2); // prints computer twice printf("str1 contains %s str3 contains %s ,
str1, str3);
Note that strcat373 is guaranteed to work properly only if str1's length is big enough to contain the concatenation of the two strings. In this case, "computer" takes up 9 bytes, and since str1 is 9 bytes, the function should run properly since the array is just long enough to contain computer (plus \0). On the other hand
:
char str1[] = "comp"; // only 5 bytes char str2[] = "uter"; strcat373(str1, str2); // takes up 9 bytes and
// therefore overflows str1
Upon execution, a runtime error may occur, or (even worse) no runtime error will occur, but some other variable(s) in your program may be overwritten.
3. Write a function called strchr373. It is passed 2 parameters: a string and a char Here is the prototype for the function:
char *strchr373(char str[], char ch); The function should return a pointer to the first instance of ch in str. For
example:
int main { char s[ ] = "abcbc"; printf("%s", strchr373(s, 'b')); printf("%s", strchr373(s, 'c'); printf("%d", strchr373(s, 'd');
// prints bcbc // prints cbc // prints 0
-
Write a function called strncpy373. It is passed 3 parameters: 2 strings and the length of the destination array (the first parameter). The intent of including a third parameter is to prevent overflow of the destination array in case the source array (the second parameter) contains a string that is too long to be stored in the destination. strncpy373 returns a pointer to the destination array.
For example:
char s1[] = "comp"; char s2[] = "systems"; strncpy373(s1, s2, 4); printf("%s ", s1);
The output of the above code should be syst.
-
Write a function called strncat373. It performs string concatenation (just like strcat373), except that it limits the number of characters that may be copied from src onto the end of dest.
The main function:
#include
#include "hw2.c"
int main() { char word1[7] = "bin", word2[] = "bag", word3[25] = "computer", word4[] = "game", word5[] = "area", word6[] = "area", word7[] = "are", word8[7] = "bo", word9[] = "ringer", word10[7] = "bo"; int cmp1 = strcmp373(word1, word2), cmp2 = strcmp373(word3, word4), cmp3 = strcmp373(word5, word6), cmp4 = strcmp373(word7, word6); printf("Test strcmp373 "); printf("%s %s = %d ", word1, word2, cmp1); printf("%s %s = %d ", word3, word4, cmp2); printf("%s %s = %d ", word5, word6, cmp3); printf("%s %s = %s ", word7, word6, cmp4); printf(" Test strcat373 "); printf("%s + %s = ", word1, word2); printf("%s ", strcat373(word1, word2)); printf("%s + \" \" + %s = ", word3, word4); strcat373(word3, " "); strcat373(word3, word4); printf("%s ", word3); printf("%s + %s = ", word8, word7+1); printf("%s ", strcat373(word8, word7+1)); printf(" Test strchr373 "); char s[ ] = "abcbc"; printf("strchr373(%s,%c) = ", s, 'b'); printf("%s", strchr373(s, 'b')); // prints bcbc printf(" "); printf("strchr373(%s,%c) = ", s, 'c'); printf("%s ", strchr373(s, 'c')); // prints cbc printf(" "); printf("strchr373(%s,%c) = ", s, 'd'); printf("%d", strchr373(s, 'd')); // prints 0 printf(" "); printf(" Test strncpy373 "); char s1[] = "comp"; char s2[] = "systems"; strncpy373(s1, s2, 4); printf("%s ", s1); printf(" Test strncat373 "); printf("%s + %s %d = ", word10, word9, 4 ); strncat373(word10, word9, 4); printf("%s ", word10); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
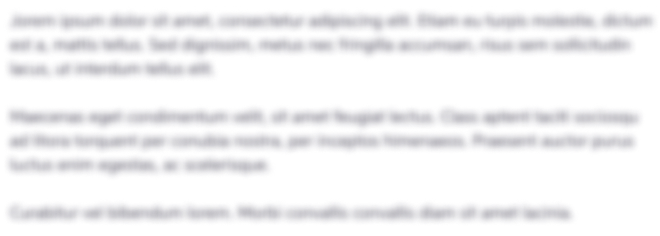
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started