Question
Notes: To break up a string into tokens, consider using the str.split method. To convert a token (string) into a number (float), consider using try/except
Notes:
To break up a string into tokens, consider using the str.split method.
To convert a token (string) into a number (float), consider using try/except blocks along with the float function.
Your functions will support the mathematical operations of +, -, *, /, //, and ^.
That last one, ^, means exponentiation. It has higher precedence than the other operators (e.g., 2 * 4 ^ 3 == 2 * 64 == 128) and is right-associative (e.g., 2 ^ 3 ^ 2 == 2 ^ (3 ^ 2) == 2 ^ 9 == 512). (Note that ^ is not how Python does exponentiation. Thats OK! This is our calculator, we can do what we want!)
All the other operators are left-associative (e.g.,2 * 3 // 4 == (2 * 3) // 4 == 6 // 4 == 1).
At no point should you ever be using the Python builtin function eval.
please use the given outline below for exp_eval.py
please contain docstrings explaining purposes
#exp_eval.py
from __future__ import annotations
def infix_to_postfix(input_string: str) -> str: """Convert the given infix string to RPN.
Args: input_string: an infix expression
Returns: The equivalent expression in RPN """
4 Converting Infix Expressions to Postfix In a file called exp_eval.py, you will implement this algorithm as a function called infix_to_postfix. We can (and you will!) also use a stack to convert an infix expression to an RPN expression via the Shunting-yard algorithm. The steps are: - Process the expression from left to right. - When you encounter a value: - Append the value to the RPN expression - When you encounter an opening parenthesis: - Push it onto the stack - When you encounter a closing parenthesis: - Until the top of the stack is an opening parenthesis, pop operators off the stack and append them to the RPN expression - Pop the opening parenthesis from the stack (but don't put it into the RPN expression) - When you encounter an operator, o1 : - While there is an operator, o2 on the top of the stack and either: * o2 has greater precedence than o1, or * they have the same precedence and o1 is left-associative Pop O2 from the stack into the RPN expression. - Then push o1 onto the stack - When you get to the end of the infix expression, pop (and append to the RPN expression) all remaining operators. The following table summarizes the operator precedence, from the highest precedence at the top to the lowest precedence at the bottom. Operators in the same box have the same precedence. For example, given the expression 3+42/(15)23 You may (and should) assume that a well formatted, correct infix expression containing only numbers, the specified operators, and parentheses will be passed to your function. You may also assume that all tokens are separated by exactly one space. You may (and should) use the Python string methods str.split (to split the string into token) and str . join (to join the RPN expression when you're done)Step by Step Solution
There are 3 Steps involved in it
Step: 1
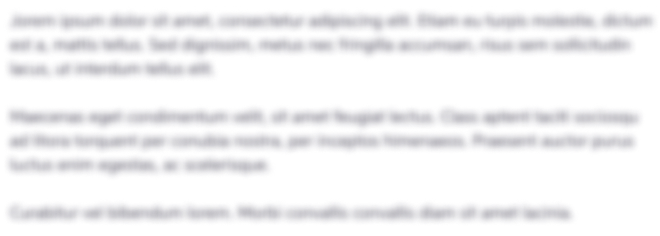
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started