Question
Notice javadoc comments that describe operations purpose, parameters and return values. In addition to these basic operations, the following are included: union operation that combines
Notice javadoc comments that describe operations purpose, parameters and return values.
In addition to these basic operations, the following are included:
union operation that combines the contents of two bags into a third bag
intersection operation that creates a third bag of only those items that occur in both two bags
difference operation that creates a third bag of the items that would be left in the given bag after removing those that also occur in another bag (see Exercise 7 in Chapter 1)
equals - returns true if the content of two bags are the same. Note that two equal bags contain the same number of entries, each entry occurs in each bag the same number of times and in the same position in the collection of objects
display - outputs the content of the bag (implemented for testing only)
getMax() - finds the largest item in the given bag
removeMin removes and returns the smallest element from the bag
moveLastToFront - moves the last item in the bag to be the first item in the same bag
findMiddleElementInOnePass finds the element in the middle of chain-of-nodes in one pass (HINT: uses two pointers that move at different speed MUST NOT rely on the number of entries)
checkIfLoopExists checks in one pass if the chain-of-nodes has a loop (HINT: uses two pointers that move at different speed)
Your Task:
1. Implement the ADT bag as the class LinkedBag. The class LinkedBag should implement the interface BagInterface. Represent the bag as a chain of linked nodes.
2. Analyze provided interface including javadoc comments that describe the purpose of each method, its parameters and return values. UML diagram is also provided for your reference.
3. Analyze the implementation of the methods provided in the LinkedBag class. Note that the main is also provided.
4. Implement the remaining methods that are stubs at this moment. Your code must not assume that the bag is not empty:
a. public boolean equals(BagInterface
b. public void removeEvery(T anEntry);
c. public T replace(T replacement);
d. public BagInterface
e. public BagInterface
f. public BagInterface
g. public T getMax();
h. public void moveLastToFront()
i. public T removeMin();
j. public boolean checkIfLoopExists();
k. public T findMiddleElementInOnePass();
5. Make sure that the output is correct (see Sample Run below).
//One return statement per method please!!!! public class LinkedBag> implements BagInterface { private Node firstNode; // reference to first node public LinkedBag() { this.firstNode = null; } // end default constructor /** * Adds a new entry to this bag. * * @param newEntry the object to be added as a new entry * @return true */ public boolean add(T newEntry) // OutOfMemoryError possible { // add to beginning of chain: Node newNode = new Node(newEntry); newNode.next = this.firstNode; // make new node reference rest of chain // (firstNode is null if chain is empty) this.firstNode = newNode; // new node is at beginning of chain return true; } // end add /** * Retrieves all entries that are in this bag. * * @return a newly allocated array of all the entries in the bag */ public T[] toArray() { int counter = 0; Node currentNode = this.firstNode; while (currentNode != null) { counter++; currentNode = currentNode.next; } // end while // the cast is safe because the new array contains null entries @SuppressWarnings("unchecked") T[] result = (T[]) new Comparable>[counter]; // unchecked cast int index = 0; currentNode = this.firstNode; while (currentNode != null && index /** * Sees whether this bag is empty. * * @return true if the bag is empty, or false if not */ public boolean isEmpty() { return this.firstNode == null; } // end isEmpty /** * Gets the number of entries currently in this bag. * * @return the integer number of entries currently in the bag */ public int getCurrentSize() { throw new UnsupportedOperationException(); } // end getCurrentSize /** * Counts the number of times a given entry appears in this bag. * * @param anEntry the entry to be counted * @return the number of times anEntry appears in the bag */ public int getFrequencyOf(T anEntry) { int frequency = 0; Node currentNode = this.firstNode; while (currentNode != null) { if (anEntry.equals(currentNode.data)) { frequency++; } // end if currentNode = currentNode.next; } // end while return frequency; } // end getFrequencyOf /** * Tests whether this bag contains a given entry. * * @param anEntry the entry to locate * @return true if the bag contains anEntry, or false otherwise */ public boolean contains(T anEntry) { return getReferenceTo(anEntry) != null; } // end contains // Locates a given entry within this bag. // Returns a reference to the node containing the entry, if located, // or null otherwise. private Node getReferenceTo(T anEntry) { boolean found = false; Node currentNode = this.firstNode; while (!found && (currentNode != null)) { if (anEntry.equals(currentNode.data)) found = true; else currentNode = currentNode.next; } // end while return currentNode; } // end getReferenceTo /** * Removes all entries from this bag. */ public void clear() { while (!isEmpty()) remove(); } // end clear /** * Removes one unspecified entry from this bag, if possible. * * @return either the removed entry, if the removal * was successful, or null */ public T remove() { T result = null; if (this.firstNode != null) { result = this.firstNode.data; this.firstNode = this.firstNode.next; // remove first node from chain } // end if return result; } // end remove /** * Removes one occurrence of a given entry from this bag, if possible. * * @param anElement the entry to be removed * @return true if the removal was successful, or false otherwise */ public boolean removeElement(T anElement) { boolean result = false; Node nodeN = getReferenceTo(anElement); if (nodeN != null) { nodeN.data = this.firstNode.data; // replace located entry with entry in first node this.firstNode = this.firstNode.next; // remove first node from chain result = true; } // end if return result; } // end remove /** * Displays all the elements in the bag */ public void display() { int counter = 0; if (this.firstNode != null) { Node currentNode = this.firstNode; while (currentNode != null) { System.out.print(currentNode.data + " "); currentNode = currentNode.next; counter++; } System.out.println(); System.out.print("There are " + counter + " element(s) in the bag."); System.out.println(); } else System.out.println("The bag is empty."); } // end display // ****** IMPLEMENT THE FOLLOWING METHODS ******** /** * Checks if the given bag called other is the same as the bag * * @param o the other bag to be compared with * @return true both bags are the same */ public boolean equals(Object o) { //TODO Project3 - implement this method first, show the instructor your finished code // see lab2 implementation for guidance return false; // THIS IS A STUB } /** * Gets the largest value in this bag. * * @returns a reference to the largest object, or null if the bag is empty */ public T getMax() { //TODO Project3 - implement this method second, show the instructor your finished code return null; //THIS IS A STUB } // end getMax /** * Removes the smallest element from the bag * * @return - null if the element was not found or the element * * NOTE: the method must traverse the data with a while loop; calls remove() */ public T removeMin() { //TODO Project3 return null; //THIS IS A STUB } // end removeMin /** * Removes every occurrence of a given entry from this bag. * For efficiency it traverses the data and removes entries as it traverses the list * without calling any other method * * @param anEntry the entry to be removed */ public void removeEvery(T anEntry) { //TODO Project3 // use only one while loop, change appropriate pointers, no calls to other methods } // end removeEvery /** * Creates a new bag that combines the contents of this bag and a * second given bag without affecting the original two bags. * * @param otherBag the given bag * @return a bag that is the union of the two bags */ public BagInterface union(BagInterface otherBag) { LinkedBag other = (LinkedBag ) otherBag; LinkedBag unionBag = new LinkedBag(); //TODO Project3 - implement this method third, show the instructor your finished code return unionBag; } // end union /** * Creates a new bag that contains those objects that occur in both this * bag and a second given bag without affecting the original two bags. * * @param otherBag the given bag * @return a bag that is the intersection of the two bags */ public BagInterface intersection(BagInterface otherBag) { LinkedBag other = (LinkedBag ) otherBag; LinkedBag intersectionBag = new LinkedBag(); LinkedBag copyOfOtherBag = new LinkedBag(); //TODO Project3 // do not call contains, call removeElement(anElement) instead return intersectionBag; } // end intersection /** * Creates a new bag of objects that would be left in this bag * after removing those that also occur in a second given bag * without affecting the original two bags. * * @param otherBag the given bag * @return a bag that is the difference of the two bags */ public BagInterface difference(BagInterface otherBag) { LinkedBag other = (LinkedBag ) otherBag; LinkedBag differenceBag = new LinkedBag(); //TODO Project3 // do not call contains, call removeElement(anElement) instead return differenceBag; } // end difference public void moveLastToFront() { //TODO Project3 // do not create a new Node object, just utilize reference variables to change appropriate pointers // DOES NOT rely on the number of elements } // end moveLastTofront /** * Replaces an entry in this bag with a given object. * * @param replacement the given object * @return the original entry in the bag that was replaced, or null if the bag is empty */ public T replace(T replacement) { //TODO Project3 // do not create a new Node object, do not call any methods - just change the data at the first node return null; //THIS IS A STUB } // end replace /** * This method finds the data in the middle node in one pass. * uses two pointers - DOES NOT rely on the number of elements * * @return returns tha data in the middle node */ public T findMiddleElementInOnePass() { //TODO Project3 return null; //THIS IS A STUB } /** * Check if the linked list has loop in one pass * uses two pointers - DOES NOT rely on the number of elements * * @return returns true as soon as the first loop is found */ public boolean checkIfLoopExists() { //TODO Project3 return false; //THIS IS A STUB } /** * This method is created to test loop detection */ private void createALoop() { // starting with a chain: A-> B-> C-> D-> E-> F-> G-> H-> I-> null Node last = this.firstNode; while (last.next != null) last = last.next; last.next = this.firstNode.next.next.next; // hardcoded a loop in the chain: A-> B-> C-> D-> E-> F-> G-> H-> I-> D } private class Node { private S data; // entry in bag private Nodenext; // link to next node private Node(S dataPortion) { this(dataPortion, null); } // end constructor private Node(S dataPortion, NodenextNode) { this.data = dataPortion; this.next = nextNode; } // end constructor } // end Node public static void main(String[] args) { System.out.println("RUNNING TEST CASES"); BagInterfacebag1 = new LinkedBag(); BagInterface bag2 = new LinkedBag(); BagInterface testBag = new LinkedBag(); BagInterface emptyBag = new LinkedBag(); bag1.add("A"); bag1.add("B"); bag1.add("A"); bag1.add("C"); bag1.add("B"); // testing display System.out.println(" ***Testing display method***"); System.out.println("emptyBag:"); emptyBag.display(); System.out.println(" bag1:"); bag1.display(); System.out.println(" bag2:"); bag2.display(); System.out.println(" After removing the first element \"" + bag1.remove() + "\" from bag1, it contains"); bag1.display(); // testing equals System.out.println(" ***Testing equals method***"); System.out.println("bag1:"); bag1.display(); System.out.println("Are bag1 and emptyBag equal? --> " + (bag1.equals(emptyBag) ? "YES" : "NO")); System.out.println("Are emptyBag and emptyBag equal? --> " + (emptyBag.equals(emptyBag) ? "YES" : "NO")); System.out.println("Are emptyBag and bag1 equal? --> " + (emptyBag.equals(bag1) ? "YES" : "NO")); bag2.add("A"); bag2.add("C"); bag2.add("A"); bag2.add("B"); bag2.add("X"); System.out.println(" bag2:"); bag2.display(); System.out.println("Are bag1 and bag2 equal? --> " + (bag1.equals(bag2) ? "YES" : "NO")); System.out.println(" Removed \"" + bag2.remove() + "\" from bag2."); bag2.display(); System.out.println("Are bag1 and bag2 equal now? --> " + (bag1.equals(bag2) ? "YES" : "NO")); LinkedBag bagCopyOfBag1 = new LinkedBag (); bagCopyOfBag1.add("A"); bagCopyOfBag1.add("B"); bagCopyOfBag1.add("A"); bagCopyOfBag1.add("C"); System.out.println(" Created bagCopyOfBag1:"); bagCopyOfBag1.display(); System.out.println("Are bag1 and bagCopyOfBag1 equal? --> " + (bag1.equals(bagCopyOfBag1) ? "YES" : "NO")); LinkedBag bagCopyOfBag1PlusOne = new LinkedBag (); bagCopyOfBag1PlusOne.add("D"); bagCopyOfBag1PlusOne.add("A"); bagCopyOfBag1PlusOne.add("B"); bagCopyOfBag1PlusOne.add("A"); bagCopyOfBag1PlusOne.add("C"); System.out.println(" Created bagCopyOfBag1PlusOne:"); bagCopyOfBag1PlusOne.display(); System.out.println("Are bagCopyOfBag1PlusOne and bagCopyOfBag1 equal? --> " + (bagCopyOfBag1PlusOne.equals(bagCopyOfBag1) ? "YES" : "NO")); System.out.println("Are bagCopyOfBag1 and bagCopyOfBag1PlusOne equal? --> " + (bagCopyOfBag1.equals(bagCopyOfBag1PlusOne) ? "YES" : "NO")); // testing getMax System.out.println(" ***Testing getMax method***"); System.out.println("The largest item in emptyBag is: " + emptyBag.getMax()); bag1.clear(); bag1.add("A"); bag1.add("A"); bag1.add("B"); bag1.add("X"); bag1.add("A"); bag1.add("C"); bag1.add("A"); System.out.println(" bag1: "); bag1.display(); System.out.println("The largest item in bag1 is: " + bag1.getMax()); bag2.clear(); bag2.add("A"); bag2.add("B"); bag2.add("B"); bag2.add("A"); bag2.add("C"); bag2.add("C"); bag2.add("D"); System.out.println(" bag2: "); bag2.display(); System.out.println("The largest item in bag2 is: " + bag2.getMax()); System.out.println(" ***Testing union, removeMin, intersection, difference and subset methods***"); System.out.println("bag1: "); bag1.display(); System.out.println("bag2: "); bag2.display(); // testing union System.out.println(" ***Testing union method***"); BagInterface everything = bag1.union(bag2); System.out.println("The union of bag1 and bag2 is "); everything.display(); everything = bag1.union(emptyBag); System.out.println(" The union of bag1 and emptyBag is "); everything.display(); everything = emptyBag.union(bag1); System.out.println(" The union of emptyBag and bag1 is "); everything.display(); // testing removeMin System.out.println(" ***Testing removeMin method***"); String smallest; while (!everything.isEmpty()) { smallest = everything.removeMin(); System.out.println("Removed the smallest element \"" + smallest + "\" from the union bag; the current content is:"); everything.display(); } smallest = everything.removeMin(); if (smallest == null) System.out.println(" The union bag is empty and removeMin returned null - CORRECT"); else System.out.println(" The union bag is empty but removeMin did not return null - INCORRECT"); // testing intersection System.out.println(" ***Testing intersection method***"); BagInterface commonItems = bag1.intersection(bag2); System.out.println("The intersection of bag1 and bag2 is "); commonItems.display(); commonItems = bag1.intersection(emptyBag); System.out.println(" The intersection of bag1 and emptyBag is "); commonItems.display(); commonItems = emptyBag.intersection(bag1); System.out.println(" The intersection of emptyBag and bag1 is "); commonItems.display(); // testing difference System.out.println(" ***Testing difference method***"); BagInterface leftOver = bag1.difference(bag2); System.out.println("The difference of bag1 and bag2 is "); leftOver.display(); leftOver = bag2.difference(bag1); System.out.println(" The difference of bag2 and bag1 is "); leftOver.display(); leftOver = bag1.difference(emptyBag); System.out.println(" The difference of bag1 and emptyBag is "); leftOver.display(); leftOver = emptyBag.difference(bag1); System.out.println(" The difference of emptyBag and bag1 is "); leftOver.display(); // testing replace System.out.println(" ***Testing replace method***"); bag1.clear(); bag1.add("A"); bag1.add("A"); bag1.add("B"); bag1.add("X"); bag1.add("A"); bag1.add("C"); bag1.add("A"); System.out.println("Bag1 contains:"); bag1.display(); System.out.println("Replaced \"" + bag1.replace("X") + "\" with \"X\""); System.out.println("Now bag1 contains:"); bag1.display(); System.out.println(" Calling replace on emptyBag"); String replaced = emptyBag.replace("X"); if (replaced == null) System.out.println("The bag is empty and replace returned null - CORRECT"); else System.out.println("The bag is empty but replace did not return null - INCORRECT"); System.out.println("Now emptyBag contains:"); emptyBag.display(); // testing removeEvery System.out.println(" ***Testing removeEvery method***"); System.out.println("Bag1 contains:"); bag1.display(); System.out.println("Removing all \"Z\""); bag1.removeEvery("Z"); System.out.println("After removing all \"Z\" bag1 contains:"); bag1.display(); System.out.println(" Removing all \"X\""); bag1.removeEvery("X"); System.out.println("After removing all \"X\" bag1 contains:"); bag1.display(); System.out.println(" After adding two \"A\" bag1 contains:"); bag1.add("A"); bag1.add("A"); bag1.display(); System.out.println("Removing all \"A\""); bag1.removeEvery("A"); System.out.println("After removing all \"A\" bag1 contains:"); bag1.display(); System.out.println(" Removing all \"B\""); bag1.removeEvery("B"); System.out.println("After removing all \"B\" bag1 contains:"); bag1.display(); System.out.println(" After removing all \"C\" emptyBag contains:"); emptyBag.display(); System.out.println(" *** TESTING moveLastToFront ***"); testBag.clear(); testBag.add("C"); testBag.add("B"); testBag.add("A"); System.out.println("List before:"); testBag.display(); testBag.moveLastToFront(); System.out.println("List after:"); testBag.display(); System.out.println(); System.out.println("Calling moveLastToFront three times"); testBag.clear(); testBag.add("B"); testBag.add("C"); testBag.add("A"); System.out.println("List before:"); testBag.display(); testBag.moveLastToFront(); testBag.moveLastToFront(); testBag.moveLastToFront(); System.out.println("List after:"); testBag.display(); System.out.println(); System.out.println("Calling moveLastToFront on a list of length 0"); testBag.clear(); System.out.println("List before:"); testBag.display(); testBag.moveLastToFront(); System.out.println("List after:"); testBag.display(); System.out.println(); System.out.println("Calling moveLastToFront on a list of length 1"); testBag.clear(); testBag.add("B"); System.out.println("List before:"); testBag.display(); testBag.moveLastToFront(); System.out.println("List after:"); testBag.display(); System.out.println(); System.out.println("Calling moveLastToFront on a list of length 2"); testBag.clear(); testBag.add("A"); testBag.add("B"); System.out.println("List before:"); testBag.display(); testBag.moveLastToFront(); System.out.println("List after:"); testBag.display(); System.out.println(); System.out.println(" *** TESTING findMiddleElementInOnePass ***"); testBag.clear(); testBag.display(); System.out.println("middle: " + testBag.findMiddleElementInOnePass()); testBag.add("A"); testBag.display(); System.out.println("middle: " + testBag.findMiddleElementInOnePass() + " "); testBag.add("B"); testBag.display(); System.out.println("middle: " + testBag.findMiddleElementInOnePass() + " "); testBag.add("C"); testBag.add("D"); testBag.add("E"); testBag.add("F"); testBag.display(); System.out.println("middle: " + testBag.findMiddleElementInOnePass() + " "); testBag.add("G"); testBag.display(); System.out.println("middle: " + testBag.findMiddleElementInOnePass() + " "); System.out.println(" *** TESTING checkIfLoopExists ***"); boolean result = testBag.checkIfLoopExists(); if (!result) System.out.println("testBag does not have a loop - CORRECT"); else System.out.println("testBag does have a loop - INCORRECT"); LinkedBag bagWithLoop = new LinkedBag(); bagWithLoop.add("I"); bagWithLoop.add("H"); bagWithLoop.add("G"); bagWithLoop.add("F"); bagWithLoop.add("E"); bagWithLoop.add("D"); bagWithLoop.add("C"); bagWithLoop.add("B"); bagWithLoop.add("A"); bagWithLoop.createALoop(); result = bagWithLoop.checkIfLoopExists(); if (result) System.out.println("bagWithLoop does have a loop - CORRECT"); else System.out.println("bagWithLoop does not have a loop - INCORRECT"); } } // end LinkedBag
Sample Run:
RUNNING TEST CASES
***Testing display method***
emptyBag:
The bag is empty.
bag1:
B C A B A
There are 5 element(s) in the bag.
bag2:
The bag is empty.
After removing the first element "B" from bag1, it contains
C A B A
There are 4 element(s) in the bag.
***Testing equals method***
bag1:
C A B A
There are 4 element(s) in the bag.
Are bag1 and emptyBag equal? --> NO
Are emptyBag and emptyBag equal? --> YES
Are emptyBag and bag1 equal? --> NO
bag2:
X B A C A
There are 5 element(s) in the bag.
Are bag1 and bag2 equal? --> NO
Removed "X" from bag2.
B A C A
There are 4 element(s) in the bag.
Are bag1 and bag2 equal now? --> NO
Created bagCopyOfBag1:
C A B A
There are 4 element(s) in the bag.
Are bag1 and bagCopyOfBag1 equal? --> YES
Created bagCopyOfBag1PlusOne:
C A B A D
There are 5 element(s) in the bag.
Are bagCopyOfBag1PlusOne and bagCopyOfBag1 equal? --> NO
Are bagCopyOfBag1 and bagCopyOfBag1PlusOne equal? --> NO
***Testing getMax method***
The largest item in emptyBag is: null
bag1:
A C A X B A A
There are 7 element(s) in the bag.
The largest item in bag1 is: X
bag2:
D C C A B B A
There are 7 element(s) in the bag.
The largest item in bag2 is: D
***Testing union, removeMin, intersection, difference and subset methods***
bag1:
A C A X B A A
There are 7 element(s) in the bag.
bag2:
D C C A B B A
There are 7 element(s) in the bag.
***Testing union method***
The union of bag1 and bag2 is
A B B A C C D A A B X A C A
There are 14 element(s) in the bag.
The union of bag1 and emptyBag is
A A B X A C A
There are 7 element(s) in the bag.
The union of emptyBag and bag1 is
A A B X A C A
There are 7 element(s) in the bag.
***Testing removeMin method***
Removed the smallest element "A" from the union bag; the current content is:
A B X A C A
There are 6 element(s) in the bag.
Removed the smallest element "A" from the union bag; the current content is:
B X A C A
There are 5 element(s) in the bag.
Removed the smallest element "A" from the union bag; the current content is:
X B C A
There are 4 element(s) in the bag.
Removed the smallest element "A" from the union bag; the current content is:
B C X
There are 3 element(s) in the bag.
Removed the smallest element "B" from the union bag; the current content is:
C X
There are 2 element(s) in the bag.
Removed the smallest element "C" from the union bag; the current content is:
X
There are 1 element(s) in the bag.
Removed the smallest element "X" from the union bag; the current content is:
The bag is empty.
The union bag is empty and removeMin returned null - CORRECT
***Testing intersection method***
The intersection of bag1 and bag2 is
B A C A
There are 4 element(s) in the bag.
The intersection of bag1 and emptyBag is
The bag is empty.
The intersection of emptyBag and bag1 is
The bag is empty.
***Testing difference method***
The difference of bag1 and bag2 is
X A A
There are 3 element(s) in the bag.
The difference of bag2 and bag1 is
B C D
There are 3 element(s) in the bag.
The difference of bag1 and emptyBag is
A A B X A C A
There are 7 element(s) in the bag.
The difference of emptyBag and bag1 is
The bag is empty.
***Testing replace method***
Bag1 contains:
A C A X B A A
There are 7 element(s) in the bag.
Replaced "A" with "X"
Now bag1 contains:
X C A X B A A
There are 7 element(s) in the bag.
Calling replace on emptyBag
The bag is empty and replace returned null - CORRECT
Now emptyBag contains:
The bag is empty.
***Testing removeEvery method***
Bag1 contains:
X C A X B A A
There are 7 element(s) in the bag.
Removing all "Z"
After removing all "Z" bag1 contains:
X C A X B A A
There are 7 element(s) in the bag.
Removing all "X"
After removing all "X" bag1 contains:
C A B A A
There are 5 element(s) in the bag.
After adding two "A" bag1 contains:
A A C A B A A
There are 7 element(s) in the bag.
Removing all "A"
After removing all "A" bag1 contains:
C B
There are 2 element(s) in the bag.
Removing all "B"
After removing all "B" bag1 contains:
C
There are 1 element(s) in the bag.
After removing all "C" emptyBag contains:
The bag is empty.
*** TESTING moveLastToFront ***
List before:
A B C
There are 3 element(s) in the bag.
List after:
C A B
There are 3 element(s) in the bag.
Calling moveLastToFront three times
List before:
A C B
There are 3 element(s) in the bag.
List after:
A C B
There are 3 element(s) in the bag.c
Calling moveLastToFront on a list of length 0
List before:
The bag is empty.
List after:
The bag is empty.
Calling moveLastToFront on a list of length 1
List before:
B
There are 1 element(s) in the bag.
List after:
B
There are 1 element(s) in the bag.
Calling moveLastToFront on a list of length 2
List before:
B A
There are 2 element(s) in the bag.
List after:
A B
There are 2 element(s) in the bag.
*** TESTING findMiddleElementInOnePass ***
The bag is empty.
middle: null
A
There are 1 element(s) in the bag.
middle: A
B A
There are 2 element(s) in the bag.
middle: B
F E D C B A
There are 6 element(s) in the bag.
middle: D
G F E D C B A
There are 7 element(s) in the bag.
middle: D
*** TESTING checkIfLoopExists ***
testBag does not have a loop - CORRECT
bagWithLoop does have a loop - CORRECT
Process finished with exit code 0
Baginterface mgetCurrentSize0) misEmpty int boolean boolean add(T) mremove() mremoveElement(T) boolean void int boolean TO void boolean r clear() mgetFrequencyOf(T) 'b contains(T) mtoArray0 mdisplay0 ' equals(Object) &Node data f next NodeeS> Node(s) 8 Node(S, NodeStep by Step Solution
There are 3 Steps involved in it
Step: 1
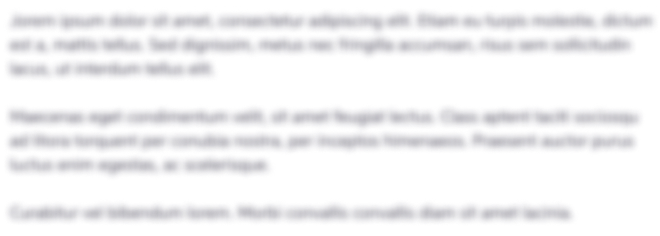
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started