Question
Now I have the code for the program which I will list below however my main problem is that the code has problems with recognizing
Now I have the code for the program which I will list below however my main problem is that the code has problems with recognizing my definitions within the Wallet class and won't compile. I need some help with that if possible. PLEASE DO NOT MAKE NEW CODE AND ONLY USE THE CODE BELOW:
currency.h
#include #include
#ifndef CURRENCY_H #define CURRENCY_H class Currency { protected: int whole; std::string wholeName; int fractional; std::string fractionalName; int fractionalPerWhole;
public: Currency::Currency(); Currency::Currency(int initWhole, int initFractional); double getAmount(); void addInt(int addAmount); void addDouble(double addAmount); void addValue(int wholeAdd, int fractionalAdd); virtual void setUp();
std::string getWholeName(); int Currency::getWhole(); int Currency::getFractional(); std::string Currency::getFractionalName();
operator double(); // Currency + Currency friend Currency operator+(const Currency &cM1, const Currency &cM2); // Currency + int friend Currency operator+(const Currency &cM, int nValue); friend Currency operator+(int nValue, const Currency &cM); // Currency + double friend Currency operator+(Currency &cM, double nValue); friend Currency operator+(double nValue, Currency &cM); friend Currency operator+=(Currency &cM, double nValue);
// cout currency.cpp
#include "currency.h" #include #include
using namespace std;
// class constructor (no argument version)
Currency::Currency() { wholeName = ""; whole = 0; fractional = 0; fractionalName = ""; }
// class constructor
Currency::Currency(int initWhole, int initFractional) { whole = initWhole; fractional = initFractional; }
// getAmount // returns the currrency whole and fractional as a // double
double Currency::getAmount() { return whole + static_cast(fractional) / fractionalPerWhole; }
// addInt // addition operator overloading for int
void Currency::addInt(int addAmount) { whole += addAmount; }
//meant to be used by operator overloading void Currency::addDouble(double addAmount) { whole += addAmount; fractional += static_cast(addAmount * fractionalPerWhole) % fractionalPerWhole; if (fractional >= fractionalPerWhole) { whole += fractional / fractionalPerWhole; fractional = fractional % fractionalPerWhole; } }
//adding value by the whole and fractional numbers void Currency::addValue(int wholeAdd, int fractionalAdd) { whole += wholeAdd; fractional += fractionalAdd % fractionalPerWhole; whole += fractionalAdd / fractionalPerWhole; if (fractional >= fractionalPerWhole) { whole += fractional / fractionalPerWhole; fractional = fractional % fractionalPerWhole; } }
// setUp // this method is overriden in test for polymorphism
void Currency::setUp() { wholeName = "Rupee"; fractionalName = "Paise"; fractionalPerWhole = 100; }
/**************************** *********** GETTERS ********* *****************************/ string Currency::getWholeName() { return wholeName; }
int Currency::getWhole() { return whole; }
int Currency::getFractional() { return fractional; }
string Currency::getFractionalName() { return fractionalName; }
/***************************************** *********** OPERATOR OVERLOADING ********* ******************************************/ Currency::operator double() { return getAmount(); }
Currency operator+(Currency &cM1, Currency &cM2) { cM1.addDouble(cM2.getAmount()); return cM1; }
Currency operator+(Currency &cM, int nValue) { cM.addInt(nValue); return cM; }
Currency operator+(int nValue, Currency &cM) { // call operator+(Currency, nValue) return (cM + nValue); }
Currency operator+(Currency &cM, double nValue) { cM.addDouble(nValue); return cM; }
Currency operator+(double nValue, Currency &cM) { // call operator+(Currency, nValue) return (cM + nValue); }
Currency operator+=(Currency &cM, double nValue) { cM.addDouble(nValue); return cM; }
ostream& operatorWallet.cpp
#include "Wallet.h" #include #include using namespace std;
Wallet::Wallet(const int CURRENCY_MAX) { currencyTypesNum = 0; //current number of currencies in the currencyObjs array currencyMax = CURRENCY_MAX; currencyObjs = new Currency*[currencyMax]; }
/* getCurrentSize get current number of currency types in the wallet */ int Wallet::getCurrentSize() { return currencyTypesNum; }
/* isEmpty is the wallet empty? */ bool Wallet::isEmpty() { if (currencyTypesNum > 0) { return false; } return true; }
/* add adds a new currency type to the wallet return: the currencyIndex for the new currency type. -1 means there is no more space. */ int Wallet::add(std::string wholeName, int whole, int fractional) { int currencyIndex = -1; if (currencyTypesNum
/* remove removes a specific currency type from the wallet */ bool Wallet::remove(int currencyIndex) { if (exist(currencyIndex)) { // shifts each index down one for (int i = currencyIndex; i addValue(wholeAdd, fractionalAdd); return true; } } /* removeValue removes whole and fractional integers to the currency type */ bool Wallet::removeValue(int currencyIndex, int wholeAdd, int fractionalAdd) { if (exist(currencyIndex)) { currencyObjs[currencyIndex]->addValue(-wholeAdd, -fractionalAdd); return true; } }
/* clear removes all currency types from the wallet */ bool Wallet::clear() { for (int i = 0; i
//finds the currency name in the wallet
bool Wallet::contains(std::string wholeNameFind) { for (int i = 0; i getWholeName() == wholeNameFind) { return true; } } return false; }
//determines if the currency index exists
bool Wallet::exist(int currencyIndex) { if (currencyIndex >= 0 && currencyIndex
Currency Wallet::operator[] (const unsigned int i) { return (*currencyObjs)[i]; } Wallet.h
#include #include "currency.h" #include "CurrencyDollar.h" #include "CurrencyEuro.h" #include "CurrencyRupee.h" #include "CurrencyYen.h" #include "CurrencyYuan.h" #ifndef WALLET_H #define WALLET_H
class Wallet { private: Currency **currencyObjs; int currencyTypesNum; int currencyMax; public: Wallet(const int CURRENCY_MAX); int getCurrentSize(); bool isEmpty(); int add(std::string initWholeName, int initWhole, int initFractional); bool remove(int currencyIndex); bool addValue(int currencyIndex, int wholeAdd, int fractionalAdd); bool removeValue(int currencyIndex, int wholeAdd, int fractionalAdd); bool clear(); bool contains(std::string initWholeName); bool exist(int currencyIndex);
Currency operator[] (const unsigned int i); }; #endif
PLEASE FIX THESE FILES ONLY and make sure there are no errors
Please program the following program in C++ language and include inheritance, overloaded addition, subtraction, comparison, input and output operators for Currency, polymorphic construction of Currency objects in the Wallet, overloaded subscript operator in Wallet and in a clear and intuitive user interactivity Please follow these instructions carefully. A. Create a currency class with four attributes -currency note, whole parts and fractional parts and currency coin such that 100 fractional parts equals 1 whole part B. Create 5 derived classes for the following currencies - Dollar, Euro, Yen, Rupee and Yuan. I know this program can be done without inheritance but it is a requirement for this lab. You will be adding and subtracting currency values using the form: Dollar, 1, 25, cent Euro, 3, 33, cent Yen, 100, 54, sen Rupee, 7, 11, paise Yuan, 50, 86, fen C. Define 3 or 4 overloaded operators to add, subtract and compare different currency objects-care should be taken that you can only perform these operation on objects of the same currency. Also, take care that fractional parts roll into whole parts. D. Define an overloaded input stream operator to accept values of any currency as well as an overloaded output stream operator to write out the value of the currency, E. When initializing currency objects, your code should demonstrate polymorphic construction. Your constructors should also use initialization listsStep by Step Solution
There are 3 Steps involved in it
Step: 1
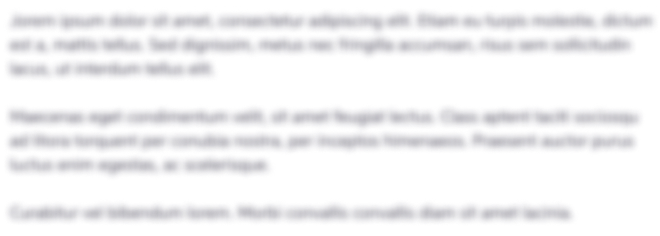
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started