Question
Now it's your turn. Define a Circle class. A circle is created similarly to a square, specifying center and radius, as in: Circle((2,3),4) (a circle
Now it's your turn. Define a Circle class. A circle is created similarly to a square, specifying center and radius, as in:
Circle((2,3),4)
(a circle of radius 4 with center in (2, 3)).
For a circle c, you should implement the following methods:
- c.contains_point(p): returns True/False according to whether the circle contains a given point p, specified as a pair (x, y) of coordinates, as in p = (2, 3).
- c.contains_square(s): returns True/False according to whether the square s is completely contained in the circle c.
- contains(x): returns True/False according to whether the circle contains the argument x, which can be either a square a point, or another Circle.
A little geometry reminder:
- A circle contains a square iff it contains all four corners of the square.
- A circle 1 contains a circle 2
- if the distance between the centers of 1 and 2 plus the radius of 2 is smaller than the radius of 1
here is my code so far import math # In case you need it.
class Circle(object):
# YOUR CODE HERE
def __init__(self, center, radius):
assert isinstance(center, tuple)
assert len(center) == 2
self.center = center
self.radius = radius
def radius(self):
return self.radius
def px(self):
"""Returns the coordinates of the positive x axis."""
(x, y) = self.center
return x + self.radius
def py(self):
"""Returns the coordinates of the positive y axis."""
(x, y) = self.center
return y + self.radius
def nx(self):
"""Returns the coordinates of the nagative x axis."""
(x, y) = self.center
return x - self.radius
def ny(self):
"""Returns the coordinates of the negative y axis."""
(x, y) = self.center
return y - self.radius
def contains_point(self, point):
"""Returns whether the point is in the square."""
assert isinstance(point, tuple)
assert len(point) == 2
x, y = point
cx, cy = self.center
Step by Step Solution
There are 3 Steps involved in it
Step: 1
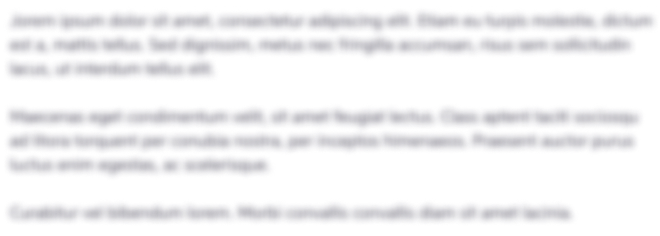
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started