Question
Now write the writePayroll() method for Payroll. The method should be public, take no arguments, and return no value. The method should sort the receivers
Now write the writePayroll() method for Payroll. The method should be public, take no arguments, and return no value.
The method should sort the receivers list of programmers from smallest to largest pay. The resulting sorted programmer list should be written to the text file "payrollResults.txt", which should be saved in the project folder for this question. The program user will need to identify the appropriate location to store the file. The output for each programmer to be written to the file is its string representation as produced by toString().
Payroll code
public class Payroll { // instance variables private List
/** * Constructor for objects of class Payroll */ public Payroll() { // initialise instance variables programmers = new ArrayList<>(); }
/** * A method that returns the programmer's grade * multiplied by the number of hours they worked. */ private int computePay(Programmer p) { return p.getGrade() * p.getHours(); } /** * 4biii * Instance method reads from the file employees.txt, making use of * BufferedReader and Scanner objects to read the file line by line, * creating a new Programmer object. */ public void readEmployeeData() { String pathname = OUFileChooser.getFilename(); File aFile = new File(pathname); BufferedReader bufferedFileReader = null; try { String programmerName; int programmerGrade; int programmerPay; int programmerHour; Scanner lineScanner; bufferedFileReader = new BufferedReader(new FileReader(aFile));
String currentLine = bufferedFileReader.readLine(); while (currentLine != null) { lineScanner = new Scanner(currentLine); lineScanner.useDelimiter(","); programmerName = lineScanner.next(); programmerGrade = lineScanner.nextInt(); programmerPay = lineScanner.nextInt(); programmerHour = lineScanner.nextInt(); Programmer programmerObject = new Programmer(programmerName); programmerObject.setGrade(programmerGrade); programmerObject.setPay(programmerPay); programmerObject.setHours(programmerHour); programmers.add(programmerObject); currentLine = bufferedFileReader.readLine(); //get the next line } } catch (Exception anException) { System.out.println("Error: " + anException); }
finally { try { bufferedFileReader.close(); } catch (Exception anException) { System.out.println("Error: " + anException); } } }
/** * 4bv * A method that iterates over the programmers, and prints * out the string representation of each programmer. */ public void showPayroll() { for(Programmer p:programmers) { System.out.println("Programmer Name = "+p.getName()+", Programmer Grade = "+p.getGrade()+ ", Programmer ID = "+p.getId()+ ", Programmer Hours = "+p.getHours()+ ", Programmer Pay = "+p.getPay()); } } /** * 4cii * Organises the receivers list of programmers from smallest to largest pay. * */ public void writePayroll() { for (Programmer eachProgrammer : programmers) { } }
Programmer code
public class Programmer { /* static variables */ private static int nextId = 1; /* instance variables */ private int id; // programmer's id private String name; // programmer's name private int grade; // Determines pay; 1, 2 or 3 private int pay; // total pay in gold coins private int hours; // total hours worked
/** * Constructor for objects of class programmer */ public Programmer(String aName) { super(); this.name = aName; this.id = nextId ++; }
public int getId() { return this.id; }
public String getName() { return name; }
public int getGrade() { return grade; }
public void setGrade(int aGrade) { if (aGrade >= 1 && aGrade <= 3) { this.grade = aGrade; } else { throw new IllegalArgumentException("The grade " + aGrade + " does not exist"); } }
public int getPay() { return pay; }
public void setPay(int pay) { this.pay = pay; }
public int getHours() { return hours; }
public void setHours(int hours) { this.hours = hours; } /** * 4iv * Returns a string describing each programmer. */ @Override public String toString() { { return "Name" + " (" + "Grade" + "," + " ID" + " " + id +") " + "Hours" + " " + "Pay" + " "; } }
/** * 4ci) * Implements the Comparable interface, where * instances of Programmer are sorted by ascending * order of the value stored by their pay instance variable. */ public int compareTo (Programmer anotherProgrammer) { return this.getPay() - (anotherProgrammer.getPay()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
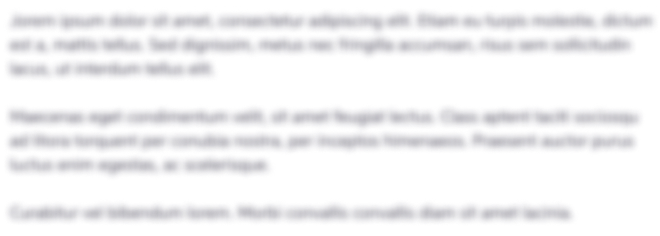
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started