Question
Objective: To use the e.printStackTrace() method to aide in locating the source of errors. Java keeps track of the program by managing a stack in
Objective: To use the e.printStackTrace() method to aide in locating the source of errors.
Java keeps track of the program by managing a stack in memory containing all processes going on in your program. The *e.printStackTrace() method can then be very useful. In this exercise, you will see how to use _e.printStackTrace(); to print out the stack history. | |
|
|
The stack trace is a very simple statement.
e.printStackTrace();
Follow this with the name of the program: StackTrace.
Use good comments in the body of the program.
Insert the e.printStackTrace() in each catch block of the try-catch framework.
Cause the program to catch an exception (of the type that the try-catch is set up for.).
You should see a listing of the lines executed just prior to the exception. It should look similar to the list below:
at java.util.Scanner.throwFor(Scanner.java:840)
at java.util.Scanner.next(Scanner.java:1461)
at java.util.Scanner.nextInt(Scanner.java:2091)
at java.util.Scanner.nextInt(Scanner.java:2050)
at C8PATryCatch.getInt(C8PATryCatch.java:26)
at C8PATryCatch.main(C8PATryCatch.java:14)
The program to use is below
--------------------------------------------------------------------
import javax.swing.JOptionPane;
public class StackTrace
{
public static void main(String[] args)
{
try
{
Divison divison = new Divison();
int firstNum = Integer.parseInt(JOptionPane.showInputDialog("Enter the first Number:"));
int secondNum = Integer.parseInt(JOptionPane.showInputDialog("Enter the second Number:"));
divison.setFirstNum(firstNum);
divison.setSecondNum(secondNum);
JOptionPane.showMessageDialog(null, divison);
}
catch (Exception e)
{
}
}
}
class Divison
{
//division setup
private int firstNum;
private int secondNum;
public Divison()
{
this.firstNum = 0;
this.secondNum = 1;
}
public Divison(int firstNum, int secondNum)
{
this.firstNum = firstNum;
this.secondNum = secondNum;
}
//return firstNum
public int getFirstNum()
{
return firstNum;
}
//return secondNum
public int getSecondNum()
{
return secondNum;
}
//set firstNum
public void setFirstNum(int firstNum)
{
this.firstNum = firstNum;
}
//set secondNum
public void setSecondNum(int secondNum)
{
this.secondNum = secondNum;
}
public double getDivison()
//math w/ if-else and try-catch
{
if (getSecondNum() != 0)
{
return (double) getFirstNum() / (double) getSecondNum();
}
else
{
try
{
throw new ArithmeticException("Cannot be zero");
} catch (ArithmeticException e)
{
System.out.println(e.getMessage());
}
}
return 0;
}
@Override
public String toString()
//solution output
{
return getFirstNum() + " Divided By " + getSecondNum()
+ " Equals: " + getDivison();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
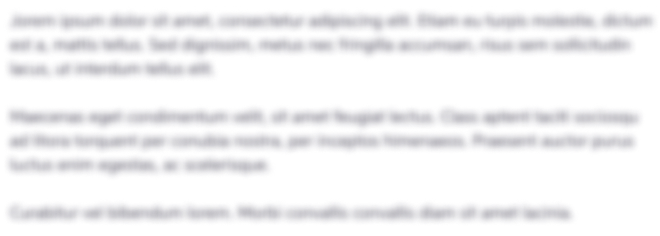
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started