Answered step by step
Verified Expert Solution
Question
1 Approved Answer
objective: trie (prefix tree) in c++ Here is the header file here is the .cpp file here is the instructions #ifndef BOGGLE_DICTIONARY_H #define BOGGLE_DICTIONARY_H 2
objective: trie (prefix tree) in c++
Here is the header file
here is the .cpp file
here is the instructions
Step by Step Solution
There are 3 Steps involved in it
Step: 1
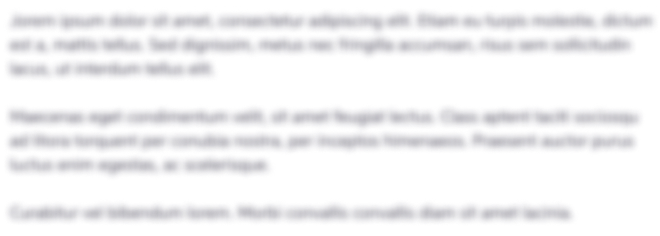
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started