Question
Objective: Your program will organize employees for each branch location and add a manager for each branch. In Java code can someone fix these errors
Objective: Your program will organize employees for each branch location and add a manager for each branch.
In Java code can someone fix these errors to make it run smoothly
import java.util.Date; import java.util.Scanner;
class Employee { private String firstName; String lastName; String fullName; Date dateOfHire; Date terminationDate; int unscheduledDays; int scheduledDays; String lastAnnualReview; int employeeId; public static int totalActiveEmployee=0; Employee() { firstName = ""; lastName = ""; fullName= firstName + " " + lastName; unscheduledDays = 0; scheduledDays = 0; lastAnnualReview = ""; dateOfHire = new Date(); terminationDate = new Date(); Employee.totalActiveEmployee++; } public Employee(String firstName, String lastName, int dd, int mm, int yy) { this.firstName=firstName; this.lastName=lastName; this.fullName=firstName+" "+lastName; this.dateOfHire=new Date(dd,mm,yy); this.terminationDate=new Date(); Employee.totalActiveEmployee++; } void setfirstName(String firstName){ this.firstName = firstName;} void setlastName(String lastName){ this.lastName = lastName;} void setfullName(String fullName){ this.fullName = fullName;} void setdateOfHire(Date dateOfHire){ this.dateOfHire = dateOfHire;} void setterminationDate(Date terminationDate){ this.terminationDate = terminationDate;} void setunscheduledDays(int unscheduledDays){ this.unscheduledDays = unscheduledDays;} void setscheduledDays(int scheduledDays){ this.scheduledDays = scheduledDays;} void setlastAnnualReview(String lastAnnualReview){ this.lastAnnualReview = lastAnnualReview;} void setemployeeId(int employeeId){this.employeeId=employeeId;}
String getfirstName(){return this.firstName;} String getlastName(){return this.lastName;} String getfullName(){return this.fullName;} Date getdateOfHire(){return this.dateOfHire;} Date getterminationDate(){return this.terminationDate;} int getunscheduledDays(){return this.unscheduledDays;} int getscheduledDays(){return this.scheduledDays;} String getlastAnnualReview(){return this.lastAnnualReview;} int getemployeeId(){return this.employeeId;} public String toString() { String s; s=this.fullName+" "; s+="Date of hire : "+this.dateOfHire+" "; s+="Scheduled Days : "+this.scheduledDays+" "; s+="UnScheduled Days : "+this.unscheduledDays; return s; } } class Branch { Manager SuperVisor; Employee []team; String locationId; String locationName; String branchId; String address;
Branch() { this.locationId = ""; this.locationName = ""; this.branchId = ""; this.address = ""; this.SuperVisor=new Manager(); } Branch (String locationId,String locationName,String branchId,String address){ this.locationId = locationId; this.locationName = locationName; this.branchId = branchId; this.address = address; this.SuperVisor=new Manager(); }
void setlocationId(String locationId){ this.locationId = locationId;} void setlocationName(String locationName){ this.locationName = locationName;} void setbranchId(String branchId){ this.branchId = branchId;} void setaddress(String address){ this.address = address;} void setsuperVisor(Manager m){this.SuperVisor=m;} String getlocationId(){return this.locationId;} String getlocationName(){return this.locationName;} String getbranchId(){return this.branchId;} String getaddress(){return this.address;} public String toString() { String s; s=this.branchId+" "; s+=this.locationName+" "; s+=this.locationId+" "; s+=this.address; return s; } void addToTeam(Employee emp) {
Employee []team=new Employee[Employee.totalActiveEmployee+1]; int i=0; for(i=0;i { team[i]=this.team[i]; } team[i]=new Employee(emp.getfirstName(),emp.getlastName(),emp.dateOfHire.getDate(),emp.dateOfHire.getMonth(),emp.dateOfHire.getYear()); this.team=team; } void removeFromTeam(Employee emp) { Employee e1; int i=0; for(i=0;i { e1=this.team[i]; if(e1.fullName==emp.fullName) { Employee.totalActiveEmployee--; } } for(int j=i;j { this.team[j]=this.team[j+1]; }
} void displayBranchInfo() { String s; s=this.SuperVisor.toString(); System.out.println(s); System.out.println("Total number of Employee is : "+Employee.totalActiveEmployee); for(int i=0;i { s=this.team[i].toString(); System.out.println(s); } }
}
public class Manager {
String firstName; String lastName; String fullName; String branchId; Manager() { this.firstName=""; this.lastName=""; this.fullName=""; this.branchId="9999"; } Manager (String firstName,String lastName,String branchId){ this.firstName = firstName;
this.lastName = lastName;
this.fullName = firstName+" "+lastName;
this.branchId = branchId;
} void setfirstName(String firstName){ this.firstName = firstName;} void setlastName(String lastName){ this.lastName = lastName;} void setbranchId(String branchId){ this.branchId = branchId;}
String getfirstName(){return this.firstName;} String getlastName(){return this.lastName;} String getfullName(){return this.fullName;} String getbranchId(){return this.branchId;} public static void main(String[] args) { // TODO code application logic here Manager m=new Manager("John","Doe","1624"); int totalBranch; System.out.println("Enter total number of branch"); Scanner sc=new Scanner(System.in); totalBranch=sc.nextInt(); Branch []branchArr=new Branch[totalBranch]; String empFirstName,empLastName; Date d=new Date(); Employee emp; int branch=0; String s; while(true) { System.out.println("1. Assign a manager to supervise the branch"); System.out.println("2. Assign an employee to the branch team"); System.out.println("3. Remove an employee from the branch team"); System.out.println("4. View a report of manager and employees for a specific lcoation"); System.out.println("5. View a cumulative report of all employees sorted by branch location,"); int choice; System.out.println("Enter your choice : "); choice=sc.nextInt(); switch(choice) { case 1: System.out.println("Enter branch id : "); s =sc.nextLine(); branchArr[branch]=new Branch(); branchArr[branch].setbranchId(s); branchArr[branch].setsuperVisor(m); branch++; break; case 2: System.out.println("Enter branch id : "); s =sc.nextLine(); System.out.println("Enter Employee first name"); empFirstName=sc.nextLine(); System.out.println("Enter Employee last name"); empLastName=sc.nextLine(); emp=new Employee(empFirstName,empLastName,d.getDay(),d.getMonth(),d.getYear()); for(int i=0;i { if(s.equals(branchArr[i].getBranchId())) branchArr[i].addToTeam(emp); } break; case 3: System.out.println("Enter branch id : "); s =sc.nextLine(); System.out.println("Enter Employee first name"); empFirstName=sc.nextLine(); System.out.println("Enter Employee last name"); empLastName=sc.nextLine(); emp=new Employee(empFirstName,empLastName,d.getDay(),d.getMonth(),d.getYear()); for(int i=0;i { if(s.equals(branchArr[i].getBranchId())) branchArr[i].removeFromTeam(emp); } break; case 4: for(int i=0;i branchArr[branch].displayBranchInfo(); break; case 5: for(int i=0;i branchArr[branch].displayBranchInfo(); } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
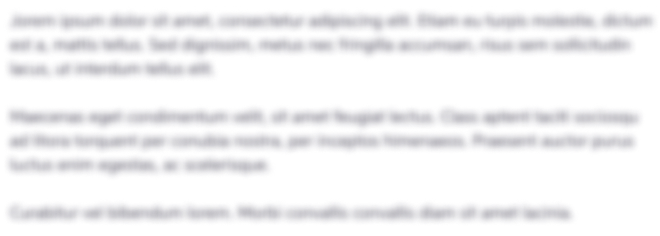
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started