Question
Objectivehow to define a package so it can be used by different applications. As well as writing / reading data to different types of files.
Objectivehow to define a package so it can be used by different applications. As well as writing / reading data to different types of files.
I will be providing the following templates below:
Employee.java HourlyEmployee.java SalariedEmployee.java CommissionEmployee.java BasePlusCommissionEmployee.java OutputBinary.java InputBinary.java
Please create the following classes:
1.Create a new application, calledTextOutput, which will use the same base code as BinaryOutput but output the data to EmployeeData.txt. Use PrintWriter should be used instead of ObjectOutputStream.
2. Create a new application, calledTextInput, which will read the newly created EmployeeData.txt and display the information to the display.
3. Create a new application, calledArrayOutput, which will use the same base code as BinaryOutput but output the complete employee array as a single element to EmployeeData.ary, instead of outputting each element individually.
4. Create a new application, calledArrayInput, which will read the newly created EmployeeData.ary as a single element and display the information to the display.
Please add these templates to your Java Application in order to fully test your code for the 4 tasks above:
Employee.java
package Employee;
import java.io.Serializable;
public abstract class Employee implements Serializable //New Modification for Task 9 { private String firstName; private String lastName; private String socialSecurityNumber;
// three-argument constructor public Employee( String first, String last, String ssn ) { firstName = first; lastName = last; socialSecurityNumber = ssn; } // end three-argument Employee constructor
// set first name public void setFirstName( String first ) { firstName = first; } // end method setFirstName
// return first name public String getFirstName() { return firstName; } // end method getFirstName
// set last name public void setLastName( String last ) { lastName = last; } // end method setLastName
// return last name public String getLastName() { return lastName; } // end method getLastName
// set social security number public void setSocialSecurityNumber( String ssn ) { socialSecurityNumber = ssn; // should validate } // end method setSocialSecurityNumber
// return social security number public String getSocialSecurityNumber() { return socialSecurityNumber; } // end method getSocialSecurityNumber
// return String representation of Employee object public String toString() { return String.format( "%s %s social security number: %s", getFirstName(), getLastName(), getSocialSecurityNumber() ); } // end method toString
// abstract method overridden by subclasses public abstract double earnings(); // no implementation here } // end abstract class Employee
HourlyEmployee.java
package Employee;
// HourlyEmployee.java // HourlyEmployee class extends Employee. public class HourlyEmployee extends Employee { private double wage; // wage per hour private double hours; // hours worked for week
// five-argument constructor public HourlyEmployee( String first, String last, String ssn, double hourlyWage, double hoursWorked ) { super( first, last, ssn ); setWage( hourlyWage ); // validate and store hourly wage setHours( hoursWorked ); // validate and store hours worked } // end five-argument HourlyEmployee constructor
// set wage public void setWage( double hourlyWage ) { wage = ( hourlyWage < 0.0 ) ? 0.0 : hourlyWage; } // end method setWage
// return wage public double getWage() { return wage; } // end method getWage
// set hours worked public void setHours( double hoursWorked ) { hours = ( ( hoursWorked >= 0.0 ) && ( hoursWorked <= 168.0 ) ) ? hoursWorked : 0.0; } // end method setHours
// return hours worked public double getHours() { return hours; } // end method getHours
// calculate earnings; override abstract method earnings in Employee public double earnings() { if ( getHours() <= 40 ) // no overtime return getWage() * getHours(); else return 40 * getWage() + ( getHours() - 40 ) * getWage() * 1.5; } // end method earnings
// return String representation of HourlyEmployee object public String toString() { return String.format( "hourly employee: %s %s: $%,.2f; %s: %,.2f", super.toString(), "hourly wage", getWage(), "hours worked", getHours() ); } // end method toString } // end class HourlyEmployee
SalariedEmployee.java
package Employee;
// SalariedEmployee.java // SalariedEmployee class extends Employee. public class SalariedEmployee extends Employee { private double weeklySalary;
// four-argument constructor public SalariedEmployee( String first, String last, String ssn, double salary ) { super( first, last, ssn ); // pass to Employee constructor setWeeklySalary( salary ); // validate and store salary } // end four-argument SalariedEmployee constructor
// set salary public void setWeeklySalary( double salary ) { weeklySalary = salary < 0.0 ? 0.0 : salary; } // end method setWeeklySalary
// return salary public double getWeeklySalary() { return weeklySalary; } // end method getWeeklySalary
// calculate earnings; override abstract method earnings in Employee public double earnings() { return getWeeklySalary(); } // end method earnings
// return String representation of SalariedEmployee object public String toString() { return String.format( "salaried employee: %s %s: $%,.2f", super.toString(), "weekly salary", getWeeklySalary() ); } // end method toString } // end class SalariedEmployee
CommissionEmployee.java
package Employee;
// CommissionEmployee.java // CommissionEmployee class extends Employee. public class CommissionEmployee extends Employee { private double grossSales; // gross weekly sales private double commissionRate; // commission percentage
// five-argument constructor public CommissionEmployee( String first, String last, String ssn, double sales, double rate ) { super( first, last, ssn ); setGrossSales( sales ); setCommissionRate( rate ); } // end five-argument CommissionEmployee constructor
// set commission rate public void setCommissionRate( double rate ) { commissionRate = ( rate > 0.0 && rate < 1.0 ) ? rate : 0.0; } // end method setCommissionRate
// return commission rate public double getCommissionRate() { return commissionRate; } // end method getCommissionRate
// set gross sales amount public void setGrossSales( double sales ) { grossSales = ( sales < 0.0 ) ? 0.0 : sales; } // end method setGrossSales
// return gross sales amount public double getGrossSales() { return grossSales; } // end method getGrossSales
// calculate earnings; override abstract method earnings in Employee public double earnings() { return getCommissionRate() * getGrossSales(); } // end method earnings
// return String representation of CommissionEmployee object public String toString() { return String.format( "%s: %s %s: $%,.2f; %s: %.2f", "commission employee", super.toString(), "gross sales", getGrossSales(), "commission rate", getCommissionRate() ); } // end method toString } // end class CommissionEmployee
BasePlusCommissionEmployee.java
package Employee;
// BasePlusCommissionEmployee.java // BasePlusCommissionEmployee class extends CommissionEmployee. public class BasePlusCommissionEmployee extends CommissionEmployee { private double baseSalary; // base salary per week
// six-argument constructor public BasePlusCommissionEmployee( String first, String last, String ssn, double sales, double rate, double salary ) { super( first, last, ssn, sales, rate ); setBaseSalary( salary ); // validate and store base salary } // end six-argument BasePlusCommissionEmployee constructor
// set base salary public void setBaseSalary( double salary ) { baseSalary = ( salary < 0.0 ) ? 0.0 : salary; // non-negative } // end method setBaseSalary
// return base salary public double getBaseSalary() { return baseSalary; } // end method getBaseSalary
// calculate earnings; override method earnings in CommissionEmployee public double earnings() { return getBaseSalary() + super.earnings(); } // end method earnings
// return String representation of BasePlusCommissionEmployee object public String toString() { return String.format( "%s %s; %s: $%,.2f", "base-salaried", super.toString(), "base salary", getBaseSalary() ); } // end method toString } // end class BasePlusCommissionEmployee
OutputBinary.java
package Employee;
import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectOutputStream;
public class OutputBinary { public static void main( String args[] ) throws IOException { // create subclass objects SalariedEmployee salariedEmployee = new SalariedEmployee( "John", "Smith", "111-11-1111", 800.00 ); HourlyEmployee hourlyEmployee = new HourlyEmployee( "Karen", "Price", "222-22-2222", 16.75, 40 ); CommissionEmployee commissionEmployee = new CommissionEmployee( "Sue", "Jones", "333-33-3333", 10000, .06 ); BasePlusCommissionEmployee basePlusCommissionEmployee = new BasePlusCommissionEmployee( "Bob", "Lewis", "444-44-4444", 5000, .04, 300 );
System.out.println( "Employees processed individually: " );
System.out.printf( "%s %s: $%,.2f ", salariedEmployee, "earned", salariedEmployee.earnings() ); System.out.printf( "%s %s: $%,.2f ", hourlyEmployee, "earned", hourlyEmployee.earnings() ); System.out.printf( "%s %s: $%,.2f ", commissionEmployee, "earned", commissionEmployee.earnings() ); System.out.printf( "%s %s: $%,.2f ", basePlusCommissionEmployee, "earned", basePlusCommissionEmployee.earnings() );
Employee employees[] = new Employee[ 4 ]; // initialize array with Employees employees[ 0 ] = salariedEmployee; employees[ 1 ] = hourlyEmployee; employees[ 2 ] = commissionEmployee; employees[ 3 ] = basePlusCommissionEmployee;
System.out.println( "Output the elements of the array: " );
/* Write code here that opens the file EmployeeData.ser for object output then writes all the elements of the array employees into the file */ /* Make sure you implement a try block to catch any IO Exceptions */ //Start for Task 10 FileOutputStream fout=null; ObjectOutputStream oos=null; File file = new File("D:\\Java Output\\EmployeeData.ser"); try { fout = new FileOutputStream(file); oos = new ObjectOutputStream(fout); // create four-element Employee array
for(Employee e:employees) oos.writeObject(e); oos.close(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } ////End for Task 10 } // end main } // end class OutputEmployees
InputBinary.java
package Employee;
import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.ObjectInputStream; import java.io.IOException; import java.lang.ClassNotFoundException;
// Lab Exercise 1, Follow-Up 1: InputEmployees.java // Employee hierarchy test program. public class InputBinary { public static void main( String args[] ) throws ClassNotFoundException { // create four-element Employee array Employee employees[] = new Employee[ 4 ];
/* Implement try block to catch possible IO Exceptions */
/* Open the ObjectInputStream from the FileInputStream */
/* Read each element from the object file */ //Start for Task 12 try { ObjectInputStream op=new ObjectInputStream(new FileInputStream("D:\\Java Output\\EmployeeData.ser"));
for(int i=0;i<4;i++) employees[i]=(Employee) op.readObject(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } //End for Task 12
System.out.println( "Employees processed polymorphically: " );
// generically process each element in array employees for ( Employee currentEmployee : employees ) { System.out.println( currentEmployee ); // invokes toString
// determine whether element is a BasePlusCommissionEmployee if ( currentEmployee instanceof BasePlusCommissionEmployee ) { // downcast Employee reference to // BasePlusCommissionEmployee reference BasePlusCommissionEmployee employee = ( BasePlusCommissionEmployee ) currentEmployee;
double oldBaseSalary = employee.getBaseSalary(); employee.setBaseSalary( 1.10 * oldBaseSalary ); System.out.printf( "new base salary with 10%% increase is: $%,.2f ", employee.getBaseSalary() ); } // end if
System.out.printf( "earned $%,.2f ", currentEmployee.earnings() ); } // end for
// get type name of each object in employees array for ( int j = 0; j < employees.length; j++ ) { //Task 12 Start System.out.println("Employee "+j+" is a Employee."+employees[j].getClass().getSimpleName()); //Task 12 Start /* Output each element Employee Type */ } } // end main } // end class InputEmployees
Step by Step Solution
There are 3 Steps involved in it
Step: 1
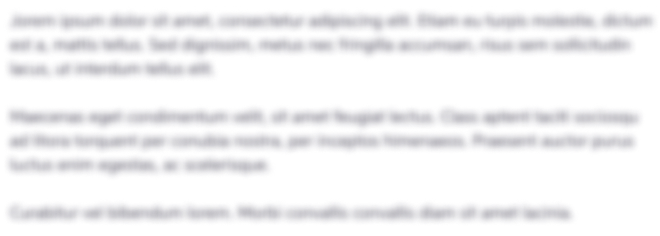
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started