Question
Objectives In this assignment you will: Demonstrate skills/techniques learned from earlier assignments. Demonstrate use of a conditional statement, document.readyState, and a DOMContentLoaded event listener to
Objectives
In this assignment you will:
Demonstrate skills/techniques learned from earlier assignments.
Demonstrate use of a conditional statement, document.readyState, and a DOMContentLoaded event listener to wait for the DOM to load before running the program.
Demonstrate the use of document.getElementsByClassName to read elements from the DOM.
Demonstrate looping through a node list to process each node.
Demonstrate the use of the textContent property to output values to the DOM.
Solve this Problem
Write a JavaScript program that solves the following problem:
Youie buys 3 items at the campus bookstore. A backpack, a calculator, and a textbook.
The backpack costs $56.99. The calculator costs $104.89. The textbook costs $51.97.
The bookstore charges 13% sales tax.
What is the subtotal amount for the 3 items?
What is the sales tax amount for the 3 items?
What is the total cost for the 3 items after the sales tax is added to the subtotal?
Set Up This Assignment
You will create new files for this assignment.
Follow these steps to create the files for this assignment:
Create a "classname.html" file in the "week5" folder. This file will be the main page for this assignment.
Create a "classname.js" file in the "week5/js" folder. This file will contain all the JavaScript for this assignment.
HTML for this Assignment
Use this HTML for this assignment. Copy this HTML and paste it into your HTML file.
Replace "Your name here" (highlighted in yellow below) with your name.
Changing the DOM by Class: getElementsClassName Changing the DOM by ID: getElementById
Introduction
In this assignment you will use JavaScript to read 3 item prices from the DOM.
Calculate a subtotal, the sales tax amount and the total cost. Then you will update the DOM with the calculated values.In this assignment you will:
Demonstrate skills/techniques learned from earlier assignments. Demonstrate the use of document.getElementsByClassName to read the prices, and getElementById to change elements from the DOM. Demonstrate the use of textContent to read and change the text contents of an element in the DOM. Purchased Items
Item Name Quantity Item Price Backpack 1 56.99 Calculator 1 104.89 Textbook 1 51.97 Subtotal Tax Total Cost
Instructions
In this assignment, you will read several HTML elements from the provided HTML file using getElementsByClassName method - which reads several elements at the same time.
You will loop through the elements, use textContent to get the text content from each element and convert the text to numbers.
Like in the previous assignments, you will do some calculations.
You will NOT output anything to the console. You will use "textContent" to change the text contents of the DOM elements.
This program needs to be written as small reusable functions. There must be no variables declared or used in the global scope.
The program must meet these criteria:
Must follow the standards followed in previous programs: include meaningful comments. Use strict mode to enforce good coding practices. Variables are initialized at the beginning. Uses "const" and "let" correctly. It does not use "var" to initialize variables. Variables are kept out of global scope by compartmentalizing them inside functions. The program is well-formatted and easy to read. The program uses small reusable functions.
The program must wait until the DOM is loaded using code based on the example given on this page: Events: Waiting for the Page to Load - link in resources listed below.
Use document.getElementsByClassName to read the 3 elements that have a class attribute of "price" from the DOM.
Loop through the elements and use the textContent property of each element to read the text content.
Convert the text content from the 3 DOM elements into numbers using the Number() method.
Calculate the sum of the item prices.
Calculate the amount of sales tax.
Calculate the total price.
Use getElementById and the textContent property to output the results to the DOM:
Output the subtotal into the text content of the element with the id of sub-total,
Output the sales tax amount into the text content of the element with the id of tax-amount,
Output the total cost into the text content of the element with the id of total.
This is my code for this assignment, but when I return it into DOM it's shows "Nah" instead of the number. Can you fix it for me? Don't change the whole code, it's must be written with functions like this.
"use strict";
// Check to see if page is still loading.
if (document.readyState == 'loading') {
// Still loading, so add an event listener to wait for it to finish.
document.addEventListener('DOMContentLoaded', showItems);
} else {
// DOM is already ready, so run the program!
showItems();
}
// Read the prices of the 3 elements from the DOM: back-price, calc-price, and text-price.
function showItems() {
const backPrice = document.getElementById("back-price");
const calcPrice = document.getElementById("calc-price");
const textPrice = document.getElementById("text-price");
// Read the text contents from the 3 DOM elements.
const backpackPrice = backPrice.textContent;
const calculatorPrice = calcPrice.textContent;
const textbookPrice = textPrice.textContent;
// Store the prices in an array
const prices = document.getElementsByClassName("price");
return prices;
}
// Function to add prices
function pricesAdd(prices) {
let sum = 0;
for (let i = 0; i < prices.length; i++) {
sum += Number(prices[i]);
}
return sum;
}
// Calculate the total price of items
function calcSum() {
const prices = showItems();
const subTotal = pricesAdd(prices);
return subTotal;
}
// Declare the tax rate
function taxRate() {
const taxRate = 0.13; // The bookstore charges 13% sales tax.
return taxRate;
}
// Calculate the sales tax
function calcTax(sum) {
let taxAmount = 0;
const rate = taxRate();
taxAmount = sum * rate;
return taxAmount;
}
// Calculate the final cost
function calcFinalCost(sum, tax) {
const finalCostAmount = sum + tax;
// Output the results
document.getElementById("sub-total").textContent = sum.toFixed(2);
document.getElementById("tax-amount").textContent = tax.toFixed(2);
document.getElementById("total").textContent = finalCostAmount.toFixed(2);
}
(function () {
const sum = calcSum();
const tax = calcTax(sum);
calcFinalCost(sum, tax);
})();
Step by Step Solution
There are 3 Steps involved in it
Step: 1
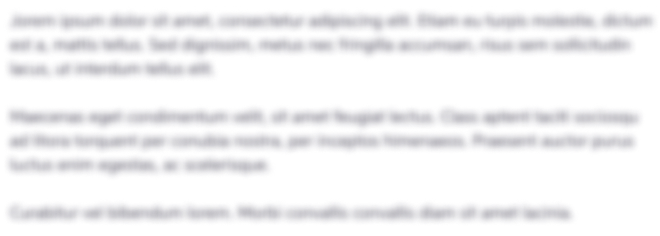
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started