Question
Objectives of this lab: Populating a single array Checking for bounds of an array Implementing a mouse event Paint with Dots For Lab 2, you're
Objectives of this lab:
Populating a single array
Checking for bounds of an array
Implementing a mouse event
Paint with Dots
For Lab 2, you're modifying the Dots and DotsPanel classes from your text to make a simple Paint program. Modify the program to
Paint with continuous dots by adding a MouseMotionListener that adds points to the pointsList when the mouseDragged event is triggered.
Add some color to the dots by changing the page.setColor(...). A hint is given below.
/* HINTS: For random-colored dots, generate three random numbers R, G, and B between 0 and 1.0 (or 0 and 255 integer) and use:
page.setColor(new Color(R,G,B)); //before drawing each dot, individually
For something more fluid, use a counter/iterator variable, and change the color based on that iterator, multiplying or adding it and modding by 256 (for each Red, Green, Blue component):
page.setColor(new Color(i%255, (i*2)%255, (i+128)%255); // really slick :) - play with multiples/adds */
//******************************************************************** // Dots.java Author: Lewis/Loftus // // Demonstrates mouse events. //********************************************************************
import javax.swing.JFrame;
public class Dots { //----------------------------------------------------------------- // Creates and displays the application frame. //----------------------------------------------------------------- public static void main(String[] args) { JFrame frame = new JFrame("Dots"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(new DotsPanel());
frame.pack(); frame.setVisible(true); } }
//******************************************************************** // DotsPanel.java Author: Lewis/Loftus // // Represents the primary panel for the Dots program. //********************************************************************
import java.util.ArrayList; import javax.swing.JPanel; import java.awt.*; import java.awt.event.*;
public class DotsPanel extends JPanel { private final int SIZE = 6; // radius of each dot
private ArrayList
//----------------------------------------------------------------- // Constructor: Sets up this panel to listen for mouse events. //----------------------------------------------------------------- public DotsPanel() { pointList = new ArrayList
addMouseListener (new DotsListener());
setBackground(Color.black); setPreferredSize(new Dimension(300, 200)); }
//----------------------------------------------------------------- // Draws all of the dots stored in the list. //----------------------------------------------------------------- public void paintComponent(Graphics page) { super.paintComponent(page);
page.setColor(Color.green);
for (Point spot : pointList) page.fillOval(spot.x-SIZE, spot.y-SIZE, SIZE*2, SIZE*2);
page.drawString("Count: " + pointList.size(), 5, 15); }
//***************************************************************** // Represents the listener for mouse events. //***************************************************************** private class DotsListener implements MouseListener { //-------------------------------------------------------------- // Adds the current point to the list of points and redraws // the panel whenever the mouse button is pressed. //-------------------------------------------------------------- public void mousePressed(MouseEvent event) { pointList.add(event.getPoint()); repaint(); }
//-------------------------------------------------------------- // Provide empty definitions for unused event methods. //-------------------------------------------------------------- public void mouseClicked(MouseEvent event) {} public void mouseReleased(MouseEvent event) {} public void mouseEntered(MouseEvent event) {} public void mouseExited(MouseEvent event) {} } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
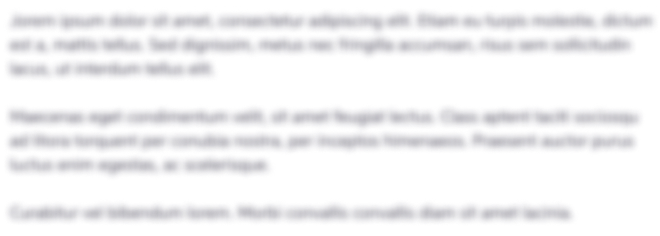
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started