Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Objectives To practice function implementation, stack usage, and memory operations Description Create a program that draws a stick figure using the Bresenham line and circle
Objectives
To practice function implementation, stack usage, and memory operations
Description
Create a program that draws a stick figure using the Bresenham line and circle algorithms. The pseudocode for the algorithms is provided below. These drawing functions are interesting in the fact that they only use integer operations and do not require any fractional math.
In your main program, execute the following commands to draw a stick figure:
Circle #head
Line #body
Line #left leg
Line #right leg
Line #left arm
Line #right arm
Circle #left eye
Circle #right eye
Line #mouth center
Line #mouth left
Line #mouth right
Requirements
Enable the bitmap display under ToolsBitmap Display
Click Connect to Program
Set the display width and height to x
Starting in the upper left, the display layout is rowbyrow:
Address x is row column
Address x is row column
Address x is row column and so on
Each word holds a byte RGB value and you can make a pixel white with the value xFFFFFF
All pixel values should be xFFFFFF
Your code should have the following functions
plot
abs absolute value
circle
line
swap does not have to be a function
Your main should have calls to circle and line to draw the figure as listed above
If you need more space to hold local variables, you can store them on the stack
Static data should only be used for the graphics display
You do not need to handle invalid arguments to any of the functions
You may have additional files, but your main program should be contain in stickfigure.asm.
For full credit, your stick figure must appear upright and not upside down.
The pseudocode for the line algorithm is:
def Line x y x y
if absy y absx x
st
else
st
if st
swapx y
swapx y
if x x
swapx x
swapy y
deltax x x
deltay absy y
error
y y
if y y
ystep
else
ystep
for x from x to xinclude x and x
if st
plotyx
else
plotxy
error error deltay
if error deltax
y y ystep
error error deltax
end if
end for loop
end function
The pseudocode for the circle algorithm is:
def Circlexc yc rxc center x coordinate, yc center y coordinate, r radius
x
y r
g r
diagonalInc r
rightInc
while x y
plot xcx ycyplot the circle points
plot xcx ycy
plot xcx ycy
plot xcx ycy
plot xcy ycx
plot xcy ycx
plot xcy ycx
plot xcy ycx
if g
g diagonalInc
diagonalInc
y
else
g rightInc
diagonalInc
rightInc
x
end while loop
end function
Step by Step Solution
There are 3 Steps involved in it
Step: 1
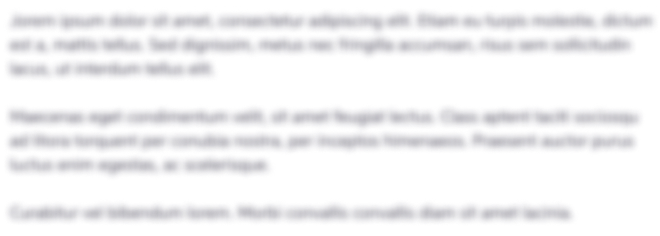
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started