Question
Object-Oriented Programming using python Below is a description of the problem and then there is a code that you work on to write all the
Object-Oriented Programming using python
Below is a description of the problem and then there is a code that you work on to write all the methods starting from the __add__ method.
Problem 1:
Create a simple class to represent a number as a fraction where your class will take the numerator and denominator as parameters.
Implement features to add, subtract, multiply, divide, return decimal value, and inverse your fraction object as indicated in the given file.
Create a CFraction class which has all the same features as the first fraction class (hint: subclass) but makes sure the fraction is in its most simplified form (e.g. 1/2 instead of 5/10).
Hint: to simplify fractions you will need the greatest common divisor, a non-recursive gcd() and recursive gcdr() functions are provided,
Please comment in a very brief explanation for gcd(), gcdr(), primeFactors(), and prod() on what the functions do. Refer to the wiki on Greatest Common Divisor.
Use the operations and print statements provided to test your code.
WORK ON THIS CODE:
PLEASE COMMENT IF YOU CAN, AS I WANT TO UNDERSTAND
from collections import Counter
#################
## EXAMPLE: simple class to represent fractions
## Try adding more built-in operations like multiply, divide
### Try adding a reduce method to reduce the fraction (use gcd)
#################
"""
class Fraction(object):
def __init__(self, num, denom):
""" num and denom are integers """
assert type(num) == int and type(denom) == int, "ints not used"
self.num = num
self.denom = denom
def __str__(self):
""" Returns a string representation of self """
return f"{self.num}/{self.denom}"
def __add__(self, other):
""" Returns a new fraction representing the addition """
return Fraction()
pass
def __sub__(self, other):
""" Returns a new fraction representing the subtraction """
pass
def __float__(self):
""" Returns a float value of the fraction """
pass
def inverse(self):
""" Returns a new fraction representing 1/self """
pass
def __mul__(self, other):
""" Returns a new fraction representing the multiplication """
pass
def _truediv_(self, other):
""" Returns a new fraction representing the division """
pass
def mult(self, other):
return self.__mul__(self, other)
////////////////////////////////////////////////
class CFraction():
def __init__(self, num, denom):
pass
def simplify(self):
pass
def __mul__(self, other):
pass
def __div__(self, other):
pass
////////////////////////////////////////////////
You will use these helper functions for the C fraction class
def prod(l): ## available in python 3.8
p = 1
for f in l:
p *= f
return p
def gcdr(a,b):
if b==0: return a
else:
return gcdr(b, a%b)
def primeFactors(num):
i = 2
if num == 1:
return []
while num%i != 0:
i+=1
return [i] + primeFactors(num//i)
def gcd(a,b):
common = Counter(primeFactors(a)) & Counter(primeFactors(b))
return prod([factor**common[factor] for factor in common])
#%%
THIS IS HOW THE OUTPUT SHOULD BE
## This is how we would like to use Fraction objects:
a = Fraction(2,3)
b = Fraction(3,4)
c = a + b
d = a - b
e = Fraction.__float__(b)
f = a.inverse()
g = a*b
h = a/b
print("Already simplified fraction: ")
print("a: " , a)
print("b: ", b)
print("a + b = " , c)
print("a - b = " , d)
print(b, " in decimal form is: " , e)
print("inverse of a is: ", f)
print("a * b = " , g)
print("a / b = " , h)
a2 = CFraction(48,60)
b2 = CFraction(120,72)
c2 = a2 + b2
d2 = a2 - b2
e2 = Fraction.__float__(b2)
f2 = a2.inverse()
g2 = a2*b2
h2 = a2/b2
print("Unsimplified fraction: ")
print("a: " , a2)
print("b: ", b2)
print("a + b = " , c2)
print("a - b = " , d2)
print(b, " in decimal form is: " , e2)
print("inverse of a is: ", f2)
print("a * b = " , g2)
print("a / b = " , h2)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
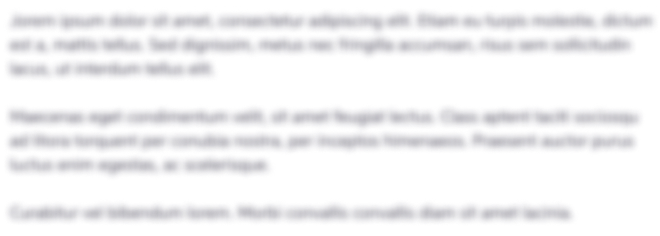
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started