Question
Objects Part 2: Inheritance (Please write the following classes in java(eclipse). Plesae keep them as simple as possible (like beginnner level) thank you!) 1) modify
Objects Part 2: Inheritance
(Please write the following classes in java(eclipse). Plesae keep them as simple as possible (like beginnner level) thank you!)
1) modify Sport2 as follows
add the following method and make the class abstract:
public abstract double computeCalories();
implement the Comparable interface, using calories to compare objects:
public int compareTo (Sport2 s)
2) create a TeamSport class that extends Sport and contains the following:
two instances variables
players: number of people on team
time: minutes of play
two constructors
no argument: default values of ice hockey, 544, 6, 60
argument: user-supplied values for activity, calories, players, time
two getters
two setters
toString() that prints values of instance variables
activity hockey
calories 544
players 6
time 60
override the equals() method from Object and have it return true if both TeamSport objects have the same number of players and minutes of play:
public boolean equals (Object o)
override the abstract method from Sport2:
public double computeCalories()
using the following formula:
calories = (minutes played + overtime) * calories / 60 where overtime = random number from 0 to 5 and each overtime is 5 m.
3) create an IndividualSport class that extends Sport2 and contains the following:
instances variable
enjoyability (1 = chore to do, 2 = indifferent, 3 = love)
two constructors
no argument: default value of 1 for enjoyability
argument: user-supplied values for activity, calories, enjoyability
getter
setter
toString() that prints values of instance variables
activity skiing
calories 654
enjoy 1
override the abstract method from Sport:
public double computeCalories()
using the following formula:
calories = minutes played * calories / 60 where time is based on enjoyability (1 = 15 minutes, 2 = half hour, 3 = hour)
Then compile and run SporProgram2.java. Your output should be similar to this
Sport s0 is skating and it burns 392 calories
The calories for s0 is being changed to 572 for a faster rate
Sport s0 now burns 572 calories per hour
Sport s3 is skiing and it burns 654 calories
Doing s0 or s3 will not burn the same number of calories
Sport s7 is sledding and it burns 572 calories
Doing s0 or s7 will burn the same number of calories
There are 8 Sport objects
Here are the properties of the Sport objects:
s0
activity skating
calories 572
enjoyability 1
s1
activity skiing
calories 654
enjoyability 3
s2
activity snowboarding
calories 429
enjoyability 1
s3
activity skiing
calories 654
enjoyability 2
s4
activity ice hockey
calories 544
players 6
time 60
s5
activity curling
calories 327
players 3
time 60
s6
activity ice hockey
calories 544
players 6
time 60
s7
activity sledding
calories 572
enjoyability 2
Does team sport s4 equal s5? no
Does s4 equal s6? yes
s6's time is being changed to 48 minutes
Does s4 equal s6? no
Seeing how sports compare caloriewise:
s1 compared to s4: s1 burns more
s6 compared to s7: s7 burns more
s0 compared to s7: both burn the same
A typical workout session for each sport:
skating 572
skiing 163
snowboarding 429
skiing 327
ice hockey 680
curling 408
ice hockey 661
sledding 286
(numbers may vary due to randomness)
The following is the Sport2 class I already wrote:
public class Sport2 {
private String activity;
private int calories;
public Sport2(){
activity="skating";
calories=392;
}
public Sport2(String act,int cals){
this.activity=act;
this.calories=cals;
}
public String getActivity(){
return activity;
}
public int getCalories(){
return calories;
}
public void setActivity(String act){
activity=act;
}
public void setCalories(int cals){
calories=cals;
}
public String toString(){
return "activty: "+activity+" calories: "+calories;
}
public boolean sameCaloriesExpended(Sport2 acts){
if(this.calories == acts.calories)
return true;
else {
return false;
}
}
static int sportObjects=0;
public static int sportObjects(){
return sportObjects;
}
}
The following is the Sportprogram class:
public class Sportprogram {
public static void main(String[] args) {
// create sport objects
Sport2 s0=new Sport2("skiing",612);
Sport2 s1=new Sport2("curling",272);
Sport2 s2=new Sport2();
Sport2 s3=new Sport2("hockey",544);
Sport2 s4=new Sport2("snowboarding",429);
// use static variable to get number of objects
System.out.println(" There are " + Sport2.sportObjects()
+ " Sport objects");
// print properties of the sport objects
System.out.println(" Here are their properties: ");
Sport2 [] sports = {s0, s1, s2, s3, s4};
for (int i = 0; i < sports.length; i++)
{
System.out.println("s" + i + " " + sports[i] + " ");
}
// use getters and setters
System.out.println("Sport s0 is " + s0.getActivity());
System.out.println("s0 is being changed to downhill skiing");
s0.setActivity("downhill skiing");
System.out.println("Sport s0 is now " + s0.getActivity());
System.out.println(" Doing s0 burns " + s0.getCalories()+ " calories");
System.out.println("s0's calories are being changed to 429 for a "
+ "slower skiing rate");
s0.setCalories(429);
System.out.println("Sport s0 now burns " + s0.getCalories() +
" calories per hour");
// compare calories burned for different sports
System.out.println(" Sport s3 is " + s3.getActivity());
String value = (s0.sameCaloriesExpended(s3)) ? "" : "not ";
System.out.println("Skiing and hockey will " + value
+ "burn the same number of calories");
System.out.println(" Sport s4 is " + s4.getActivity());
value = (s0.sameCaloriesExpended(s4)) ? "" : "not ";
System.out.println("Skiing and snowboarding will " + value
+ "burn the same number of calories ");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
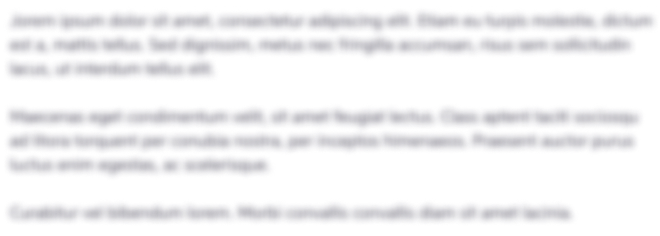
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started