Question
Only 3 methods in the ArrayBag program need to be completed: boolean remove (T item) , T removeRandom() . T getRandom() I am having a
Only 3 methods in the ArrayBag program need to be completed: boolean remove (T item) , T removeRandom() . T getRandom()
I am having a tough time wrapping my head around the logic anf necessary loops for each method.
Bags (ArrayBag)
To give users the ability to remove a particular item, or to remove or retrieve a random item, we will add the following methods to our Bag interface:
boolean remove(T item);
T removeRandom();
T getRandom();
Implement these three methods for ArrayBag. Then test them using the Bags drive program. Your output should look like this:
Here's what's in our bag
[orange, grape, kiwi, coconut, lime]
Does our bag contain the word 'kiwi'? yes
Does our bag contain the word 'mango'? no
Selecting an item (always same)
lime
lime
Selecting a random item (varies)
coconut
kiwi
Removing 'grape' from the bag
[orange, lime, kiwi, coconut]
Removing an item (always end one)
coconut [orange, lime, kiwi]
Removing a random item
lime
[orange, kiwi]
Let's empty the bag
[ ]
Trying to get a random item
null
Trying to remove a random item
null
Trying to remove kiwi
false
//Given interface
public interface Bag { void add (T item); T remove(); T get(); boolean contains (T item); int size(); public String toString(); boolean remove (T item); T removeRandom(); T getRandom(); }
//Given driver program (main method)
/* * Bags * * program for testing methods in ArrayBag and LinkedBag */ public class Bags { public static void main (String [] args) { try { Bag words = new ArrayBag(); //Bag words = new LinkedBag(); // adding to bag String [] fruits = {"orange", "grape", "kiwi", "coconut", "lime"}; for (int i = 0; i < fruits.length; i++) { words.add(fruits[i]); } System.out.println(" Here's what's in our bag " + words); // seeing if bag contains item boolean result = words.contains("kiwi"); System.out.println(" Does our bag contain the word 'kiwi'? " + (result ? "yes" : "no")); result = words.contains("mango"); System.out.println("Does our bag contain the word 'mango'? " + (result ? "yes" : "no")); // retrieving item System.out.println(" Selecting an item (always same)"); System.out.println(words.get()); System.out.println(words.get()); // retrieving random item System.out.println(" Selecting a random item (varies)"); System.out.println(words.getRandom()); System.out.println(words.getRandom()); // removing specific item words.remove("grape"); System.out.println(" Removing 'grape' from the bag " + words); // removing item System.out.println(" Removing an item (always end one)"); System.out.println(words.remove()); System.out.println(words); // removing random item System.out.println(" Removing a random item"); System.out.println(words.removeRandom()); System.out.println(words + " "); // testing methods on empty bag System.out.println("Let's empty the bag"); words.remove(); words.remove(); System.out.println(words); System.out.println("Trying to get a random item " + words.getRandom()); System.out.println("Trying to remove a random item " + words.removeRandom()); System.out.println("Trying to remove 'kiwi' " + words.remove("kiwi")); } catch (IllegalArgumentException e) { System.out.println(e.getMessage()); } } }
//ArrayBag implementation
/* * ArrayBag * * array-based implementation of a bag * * unordered collection with duplicates allowed */ public class ArrayBag implements Bag { public static final int DEFAULT_CAPACITY = 10; private T [] collection; private int size; /* * default constructor * * bag initially set to 10 items */ public ArrayBag() { this(DEFAULT_CAPACITY); } /* * argument constructor * * size of bag specified by user */ @SuppressWarnings("unchecked") public ArrayBag(int capacity) { if (capacity < 0) { throw new IllegalArgumentException("bag can't be negative size!"); } collection = (T []) new Object [capacity]; size = 0; } /* * adds item to bag * * if at capacity, bag is resized */ public void add (T item) { checkForNull(item); ensureSpace(); collection[size] = item; size++; } /* * removes item from bag * * returns removed item */ public T remove () { if (size == 0) { return null; } T removed = collection[size-1]; collection[size-1] = null; size--; return removed; } /* * returns item from bag */ public T get() { if (size == 0) { return null; } return collection[size-1]; } /* * returns true if item is in bag, false otherwise */ public boolean contains(T item) { for (int i = 0; i < size; i++) { if (collection[i].equals(item)) { return true; } } return false; } /* * returns size of bag */ public int size() { return size; } /* * returns string representation of contents of bag */ public String toString() { String s = "["; for (int i = 0; i < size; i++) { s+= collection[i]; if (i < size -1) { s+= ", "; } } return s + "]"; } /* methods to be added */ /* * removes specified item from bag * * returns false if item not present */ public boolean remove (T item) { return false; } /* * removes random item from bag * * returns null if bag is empty */ public T removeRandom () { return null; } /* * returns random item from bag * * returns null if bag is empty */ public T getRandom () { return null; } /* end of added methods */ /* * checks if item is null and throws exception if so */ private void checkForNull (T item) { if (item == null) { throw new IllegalArgumentException("null not a possible value!"); } } /* * doubles size of array if at capacity */ private void ensureSpace() { if (size == collection.length) { @SuppressWarnings("unchecked") T [] larger = (T []) new Object [size * 2]; for (int i = 0; i < size; i++) { larger[i] = collection[i]; } collection = larger; larger = null; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
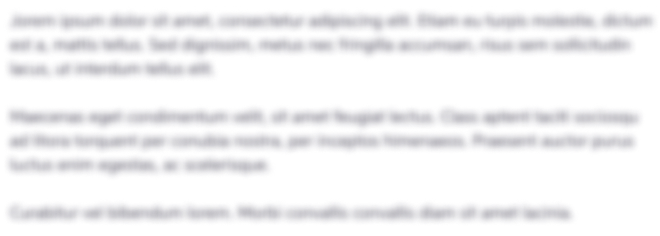
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started