Question
ONLY EDITABLE FILE ACCORDING TO DIRECTIONS transcript: 1 /* This class counts the occurances of the vowels, i.e. characters: A,a,E,e,I,i,O,o,U,u. 2 * The vowel counts
ONLY EDITABLE FILE ACCORDING TO DIRECTIONS
transcript:
1 /* This class counts the occurances of the vowels, i.e. characters: A,a,E,e,I,i,O,o,U,u. 2 * The vowel counts are case-insensitive. 3 * For example, 'A' and 'a' are both counted as the same character. 4 * Users may add a specific character, any letter or punctuation mark, of their choice to be counted. 5 * The user-specified character is optional, and if that option has been requested, the count 6 * is case-sensitive. 7 * By default, the user character is initialized to the minimum possible char value by referencing 8 * the public constant MIN_VALUE in the Character class. However, the method userCharSet returns false 9 * unless the user character has been requested- by a call to setUserChar. 10 * The count of all characters is also maintained. Note that space charcters are counted. 11 * The current counts are provided on demand by "get" methods. 12 */ 13 public class VowelCounter { 14 // the user-specific character. 15 private char userChar; 16 17 // TODO: Declare any other member variables (private) below. 18 19 20 /* Constructor, where all variables are initialized to default values. 21 */ 22 public VowelCounter(){ 23 //default value for user char- this is just a placeholder. 24 userChar = Character.MIN_VALUE; 25 26 //TODO: initialize the other variables you have declared. 27 28 } 29 30 /* This method counts the vowels in a line of text passed in. 31 * It also checks if a user-specified character has been set and will 32 * count any occurences of that character. 33 * This method also updates the total number of characters, including spaces, 34 * that have been processed. 35 * Note that no counts are incremented if the line passed in has zero length. 36 */ 37 public void processLine(String line) { 38 //TODO: implement this method. 39 40 } 41 42 /* This method allows a user to set an arbitrary character to be counted. 43 * This character is treated as case sesitive. 44 * The user's character is passed in as a String, which is converted 45 * to a single char data type. 46 */ 47 public void setUserChar(String userCharStr) { 48 //TODO: implement this method. 49 50 } 51 52 /* Returns true if the user has set a special character to count, 53 * false otherwise. 54 */ 55 public boolean userCharSet(){ 56 //TODO: implement this method. 57 return true; 58 } 59 60 /* Returns the user-specified character. */ 61 public char getUserChar(){ 62 //TODO: implement this method. 63 return Character.MAX_VALUE; 64 } 65 66 /* Returns the count of vowel A. */ 67 public int getCountA(){ 68 //TODO: implement this method. 69 return -1; 70 } 71 72 /* Returns the count of vowel E. */ 73 public int getCountE(){ 74 //TODO: implement this method. 75 return -1; 76 } 77 78 /* Returns the count of vowel I. */ 79 public int getCountI(){ 80 //TODO: implement this method. 81 return -1; 82 } 83 84 /* Returns the count of vowel O. */ 85 public int getCountO(){ 86 //TODO: implement this method. 87 return -1; 88 } 89 90 /* Returns the count of vowel U. */ 91 public int getCountU(){ 92 //TODO: implement this method. 93 return -1; 94 } 95 96 /* Returns the count of all characters processed. */ 97 public int getTotalCharCount(){ 98 //TODO: implement this method. 99 return -1; 100 } 101 102 /* Returns the count of the user's character. */ 103 public int getUserCharCount(){ 104 //TODO: implement this method. 105 return -1; 106 } 107 }
THIS IS THE MAIN FILE
transcript:
import java.util.Scanner; 2 /* This class runs a console interface between a user and 3 * a VowelCounter object. 4 */ 5 public class VowelCounterMain { 6 7 public static void main(String[] args){ 8 9 System.out.println("Vowel Counter"); 10 Scanner scan = newScanner(System.in); 11 VowelCounter counter = newVowelCounter(); 12 boolean keepGoing = true; 13 String inputStr = ""; 14 15 while(keepGoing) { 16 System.out.println("Main Menu:"); 17 System.out.println("Enter A to add a special character to be counted."); 18 System.out.println("Enter E to enter a line of text."); 19 System.out.println("Enter P to print the report."); 20 System.out.println("Enter X to quit."); 21 System.out.println(""); 22 inputStr = scan.nextLine(); 23 24 if (inputStr.equalsIgnoreCase("E")){ 25 System.out.println("Enter a line of text: "); 26 inputStr = scan.nextLine(); 27 counter.processLine(inputStr); 28 } 29 else if(inputStr.equalsIgnoreCase("A")){ 30 System.out.println("Enter a single character to count."); 31 String userCharStr = scan.nextLine(); 32 counter.setUserChar(userCharStr); 33 } 34 else if(inputStr.equalsIgnoreCase("P")){ 35 printReport(counter); 36 } 37 elseif(inputStr.equalsIgnoreCase("X")){ 38 keepGoing = false; 39 } 40 else 41 System.out.println("Unrecognized input."); 42 System.out.println(""); 43 } 44 System.out.println("Bye for now."); 45 scan.close(); 46 } 47 48 /* Using the data stored in a VowelCounter object, this method prints a report 49 * of all vowel and special character counts. 50 * The counts as a percentage of the total character count are also printed. 51 */ 52 public static voidprintReport(VowelCounter counter){ 53 System.out.println("Frequency Report:"); 54 System.out.println("A count: "+counter.getCountA()); 55 System.out.println("E count: "+counter.getCountE()); 56 System.out.println("I count: "+counter.getCountI()); 57 System.out.println("O count: "+counter.getCountO()); 58 System.out.println("U count: "+counter.getCountU()); 59 if(counter.userCharSet()) 60 System.out.println(""+counter.getUserChar()+" count: "+counter.getUserCharCount()); 61 System.out.println(counter.getTotalCharCount()+" total characters counted."); 62 int totalCharCount = counter.getTotalCharCount(); 63 System.out.printf("%n"); 64 if(totalCharCount>0){ 65 System.out.printf("percent A: %4.2f%n",(counter.getCountA()/(double)totalCharCount)*100); 66 System.out.printf("percent E: %4.2f%n",(counter.getCountE()/(double)totalCharCount)*100); 67 System.out.printf("percent I: %4.2f%n",(counter.getCountI()/(double)totalCharCount)*100); 68 System.out.printf("percent O: %4.2f%n",(counter.getCountO()/(double)totalCharCount)*100); 69 System.out.printf("percent U: %4.2f%n",(counter.getCountU()/(double)totalCharCount)*100); 70 if(counter.userCharSet()){ 71 System.out.print("percent "+counter.getUserChar()); 72 System.out.printf(": %4.2f%n",(counter.getUserCharCount()/(double)totalCharCount)*100); 73 } 74 } 75 } 76 }
THIS IS THE TEST FILE
transcript:
1 import org.junit.Assert; 2 import static org.junit.Assert.*; 3 import org.junit.Before; 4 import org.junit.Test; 5 6 7 public class VowelCounterTest {//expected, actual 8 9 VowelCounter counter; 10 int count; 11 12 /** Fixture initialization (common initialization 13 * for all tests). **/ 14 @Before public void setUp() { 15 counter = new VowelCounter(); 16 } 17 18 /** Test count initializations. **/ 19 20 @Test public void initCountATest() { 21 count = counter.getCountA(); 22 assertEquals("Test 1: Count A initialization.", 0, count); 23 } 24 25 @Test public void initCountETest() { 26 count = counter.getCountE(); 27 assertEquals("Test 2: Count E initialization.", 0, count); 28 } 29 30 @Test public void initCountITest() { 31 count = counter.getCountI(); 32 assertEquals("Test 3: Count I initialization.", 0, count); 33 } 34 35 @Test public void initCountOTest() { 36 count = counter.getCountO(); 37 assertEquals("Test 4: Count O initialization.", 0, count); 38 } 39 40 @Test public void initCountUTest() { 41 count = counter.getCountU(); 42 assertEquals("Test 5: Count U initialization.", 0, count); 43 } 44 45 @Test public voidinitCountUserCharTest() { 46 count = counter.getUserCharCount(); 47 assertEquals("Test 6: Count userChar initialization.", 0, count); 48 } 49 50 @Test public void initCharCountTest() { 51 count = counter.getTotalCharCount(); 52 assertEquals("Test 7: Character count initialization.", 0, count); 53 } 54 55 @Test public void initUserCharTest() { 56 char userChar = counter.getUserChar(); 57 assertEquals("Test 8: User character default initialization.", Character.MIN_VALUE, userChar); 58 } 59 60 @Test public voidinitUserCharSetTest() { 61 boolean actualBool = counter.userCharSet(); 62 assertEquals("Test 9: Has user character default initialization.", false, actualBool); 63 } 64 65 /** processLine tests **/ 66 67 @Test public void emptyProcLineTest() { 68 counter.processLine(""); 69 count = counter.getTotalCharCount(); 70 assertEquals("Test 10: Char count on zero length line.", 0, count); 71 } 72 73 @Test public voidincrementLineCtProcLineTest() { 74 counter.processLine("test"); 75 count = counter.getTotalCharCount(); 76 assertEquals("Test 11: Line count increments correctly.", 4, count); 77 } 78 79 @Test public void countAProcLineTest() { 80 counter.processLine("As the TA said, let's all be happy."); 81 count = counter.getCountA(); 82 assertEquals("Test 12: Count A increments correctly.", 5, count); 83 } 84 85 @Test public void countEProcLineTest() { 86 counter.processLine("Everybody expects a nice evening."); 87 count = counter.getCountE(); 88 assertEquals("Test 13: Count E increments correctly.", 7, count); 89 } 90 91 @Test public void countIProcLineTest() { 92 counter.processLine("I'm instantiating a very interesting class."); 93 count = counter.getCountI(); 94 assertEquals("Test 14: Count I increments correctly.", 6, count); 95 } 96 97 @Test public void countOProcLineTest() { 98 counter.processLine("Who doesn't object to observing Object-oriented programers at work?."); 99 count = counter.getCountO(); 100 assertEquals("Test 15: Count O increments correctly.", 9, count); 101 } 102 103 @Test public void countUProcLineTest() { 104 counter.processLine("Usually, ululating Ural owls roost under the cedar boughs."); 105 count = counter.getCountU(); 106 assertEquals("Test 16: Count U increments correctly.", 7, count); 107 } 108 109 /* multiple process line tests */ 110 111 @Test public voidvowelMultipleProcLineTest() { 112 int[] correctCts = {9,7,7,4,2}; 113 int[] studentCts = new int [5]; 114 counter.processLine("You are getting practice with conditionals."); 115 counter.processLine("Please, call me a taxi."); 116 counter.processLine("You're a taxi!"); 117 studentCts[0] = counter.getCountA(); 118 studentCts[1] = counter.getCountE(); 119 studentCts[2] = counter.getCountI(); 120 studentCts[3] = counter.getCountO(); 121 studentCts[4] = counter.getCountU(); 122 assertArrayEquals("Test 17: Vowel counts on multiple lines.", correctCts, studentCts); 123 } 124 125 /* more proc line tests */ 126 127 @Test public void vowelProcLineTest1() { 128 int[] correctCts = {3,2,2,2,2}; 129 int[] studentCts = new int [5]; 130 counter.processLine("AeIoUaOEuai"); 131 studentCts[0] = counter.getCountA(); 132 studentCts[1] = counter.getCountE(); 133 studentCts[2] = counter.getCountI(); 134 studentCts[3] = counter.getCountO(); 135 studentCts[4] = counter.getCountU(); 136 assertArrayEquals("Test 18: Line count increments.", correctCts, studentCts); 137 } 138 139 @Test public void vowelProcLineTest2() { 140 int[] correctCts = {3,2,2,2,2}; 141 int[] studentCts = new int [5]; 142 counter.processLine("AeIoUaOEuai"); 143 studentCts[0] = counter.getCountA(); 144 studentCts[1] = counter.getCountE(); 145 studentCts[2] = counter.getCountI(); 146 studentCts[3] = counter.getCountO(); 147 studentCts[4] = counter.getCountU(); 148 assertArrayEquals("Test 19: Line count increments.", correctCts, studentCts); 149 } 150 151 /* Test if user character gets set */ 152 @Test public void setUserCharTest() { 153 char testChar = 'm'; 154 String testCharStr = "m"; 155 counter.setUserChar(testCharStr); 156 char userChar = counter.getUserChar(); 157 assertTrue("Test 20: User character was set correctly.", Character.toLowerCase(userChar) == testChar); 158 } 159 160 @Test public voidsetUserCharNonAlphaTest() { 161 char testChar = '!'; 162 String testCharStr = "!"; 163 counter.setUserChar(testCharStr); 164 char userChar = counter.getUserChar(); 165 assertTrue("Test 21: User character was set correctly.", userChar == testChar); 166 } 167 168 @Test public voidsetUserCharNumberTest() { 169 char testChar = '9'; 170 String testCharStr = "9"; 171 counter.setUserChar(testCharStr); 172 char userChar = counter.getUserChar(); 173 assertTrue("Test 22: User character was set correctly.", userChar == testChar); 174 } 175 176 @Test public void setHasUserCharTest() { 177 counter.setUserChar("M"); 178 boolean actualBool = counter.userCharSet(); 179 assertEquals("Test 23: Has user character boolean set correctly.", true, actualBool); 180 } 181 182 @Test public voidcountUserCharProcLineTest() { 183 counter.setUserChar("m"); 184 counter.processLine("Mostly, I do mind if you mention logical operators."); 185 count = counter.getUserCharCount(); 186 assertEquals("Test 24: User char increments correctly.", 2, count); 187 } 188 189 @Test public voidcountUserCharProcLineTest1() { 190 counter.setUserChar("M"); 191 counter.processLine("Mostly, I do mind if you mention logical operators."); 192 count = counter.getUserCharCount(); 193 assertEquals("Test 25: User char increments correctly.", 1, count); 194 } 195 196 @Test public voidcountUserCharProcLineTest2() { 197 counter.setUserChar("m"); 198 counter.processLine("Mostly, I do mind if you mention logical operators."); 199 count = counter.getUserCharCount(); 200 assertEquals("Test 26: User char increments correctly.", 2, count); 201 } 202 203 @Test public voidcountUserCharProcLineTest3() { 204 counter.setUserChar("#"); 205 counter.processLine("If you dial # you mean # not ###."); 206 count = counter.getUserCharCount(); 207 assertEquals("Test 27: User char increments correctly.", 5, count); 208 } 209 210 211 } 212
Step by Step Solution
There are 3 Steps involved in it
Step: 1
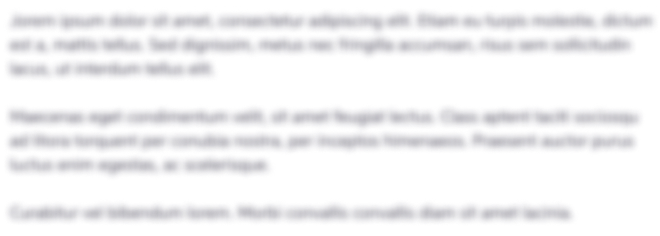
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started