Question
OOP, Class Hierarchies and Interfaces. PLEASE DO NOT CHANGE OTHER CODES EXCEPT CheeseFromager. public class Brie extends Soft { /** * @param name the
OOP, Class Hierarchies and Interfaces.
public class Brie extends Soft {
/**
* @param name the name.
* @param percentFat the fat.
*/
public Brie(String name, Double percentFat) {
super(name,CheeseType.FRESH, percentFat);
}
@Override
public String toString() {
return "Brie [name=" + getName() + ", type=" + getType() + ", percentFat()="
+ getPercentFat() + "]";
}
}
public class Cheddar extends Firm {
/**
* Creates a new Cheddar.
*
* @param name the name.
*/
public Cheddar(String name) {
super(name, CheeseType.FIRM, Math.random() * .55 + 0.5);
}
/**
* Creates a new Cheddar.
* @param name the name.
* @param percentFat the fat.
*/
public Cheddar(String name, Double percentFat) {
super(name, CheeseType.FIRM, percentFat);
}
@Override
public String toString() {
return "Cheddar [name=" + getName() + ", type=" + getType() + ", percentFat()="
+ getPercentFat() + "]";
}
}
public abstract class Cheese implements Comparable
private String name;
private CheeseType type;
private Double percentFat;
/**
* Creates a new Cheese.
*
* @param name the name.
* @param type the type.
* @param percentFat the percenFate.
*/
protected Cheese(String name, CheeseType type, Double percentFat) {
super();
this.name = name;
this.type = type;
this.percentFat = percentFat;
}
/**
* Returns the name.
*
* @return the name
*/
public String getName() {
return name;
}
/**
* Sets the name to name.
*
* @param name the name to set
*/
public void setName(String name) {
this.name = name;
}
/**
* @return the type
*/
public CheeseType getType() {
return type;
}
/**
* Returns the percenFat.
*
* @return the percentFat.
*/
public Double getPercentFat() {
return percentFat;
}
/**
* Returns the percenFat.
*
* @return the percentFat.
*/
public Double getFatPercentage() {
return percentFat;
}
@Override
public int compareTo(Cheese o) {
return this.name.compareTo(o.name);
}
@Override
public String toString() {
return "Cheese [name=" + name + ", type=" + type + ", percentFat=" + percentFat + "]";
}
}
public interface CheeseFromanger {
/**
* Makes a Cheese with the given name, type and fat percentage.
*
* @param name the name of the cheese.
* @param type the type of the cheese.
* @param fatPercentage the fat percentage of the cheese.
* @return a new instance of Cheese.
*/
Cheese makeCheese(String name, CheeseType type, Double fatPercentage);
/**
* Makes a Cheese with the given name, type and a random fat percentage.
*
* @param name the name of the cheese.
* @param type the type of the cheese.
* @return a new instance of Cheese.
*/
Cheese makeCheese(String name, CheeseType type);
/**
* Makes a Mozzarella with the given name and fat percentage.
*
* @param name the name of the mozzarella.
* @param fatPercentage the fat percentage
* @return a new instance of Mozzarella.
*/
Cheese makeMozzarella(String name, Double fatPercentage);
/**
* Makes a Mozzarella with the given name and random fat percentage.
*
* @param name the name of the mozzarella.
* @return a new instance of Mozzarella.
*/
Cheese makeMozzarella(String name);
/**
* Makes a Brie with the given name and fat percentage.
*
* @param name the name of the brie.
* @param fatPercentage the fat percentage
* @return a new instance of Brie.
*/
Cheese makeBrie(String name, Double fatPercentage);
/**
* Makes a Brie with the given name and random fat percentage.
*
* @param name the name of the brie.
* @return a new instance of Brie.
*/
Cheese makeBrie(String name);
/**
* Makes a Cheddar with the given name and fat percentage.
*
* @param name the name of the cheddar.
* @param fatPercentage the fat percentage
* @return a new instance of Cheddar.
*/
Cheese makeCheddar(String name, Double fatPercentage);
/**
* Makes a Cheddar with the given name and random fat percentage.
*
* @param name the name of the cheddar.
* @return a new instance of Cheddar.
*/
Cheese makeCheddar(String name);
/**
* Makes a Parmesan with the given name and fat percentage.
*
* @param name the name of the parmesan.
* @param fatPercentage the fat percentage
* @return a new instance of Parmesan.
*/
Cheese makeParmesan(String name, Double fatPercentage);
/**
* Makes a Parmesan with the given name and random fat percentage.
*
* @param name the name of the parmesan.
* @return a new instance of Parmesan.
*/
Cheese makeParmesan(String name);
}
import java.util.Random;
/**
* Represents a CheeseType.
*
* @author .
*
*/
public enum CheeseType {
FRESH, SOFT, FIRM;
/**
* Returns a random CheeseType.
*
* @return a random CheeseType.
*/
public static CheeseType getRandomCheeseType() {
Random random = new Random();
return values()[random.nextInt(values().length)];
}
}
public abstract class Firm extends Cheese {
/**
* Creates a new Fresh.
*
* @param name the name.
* @param type the type.
* @param percentFat the percentage.
*/
protected Firm(String name, CheeseType type, Double percentFat) {
super(name, type, percentFat);
}
}
public abstract class Fresh extends Cheese {
/**
* @param name the name.
* @param type the type.
* @param percentFat the percentage.
*/
protected Fresh(String name, CheeseType type, Double percentFat) {
super(name, type, percentFat);
}
}
public class ManoaCheeseFromager implements CheeseFromanger {
private static ManoaCheeseFromager instance;
/**
* Returns the singleton instance.
* @return the singleton instance.
*/
public static ManoaCheeseFromager getInstance() {
if (instance == null) {
instance = new ManoaCheeseFromager();
}
return instance;
}
/**
* Creates a new ManoaCheeseFromager.
*/
private ManoaCheeseFromager() {
}
@Override
public Cheese makeCheese(String name, CheeseType type, Double fatPercentage) {
return null;
}
@Override
public Cheese makeCheese(String name, CheeseType type) {
return null;
}
@Override
public Cheese makeMozzarella(String name, Double fatPercentage) {
return null;
}
@Override
public Cheese makeMozzarella(String name) {
return null;
}
@Override
public Cheese makeBrie(String name, Double fatPercentage) {
return null;
}
@Override
public Cheese makeBrie(String name) {
return null;
}
@Override
public Cheese makeCheddar(String name, Double fatPercentage) {
return null;
}
@Override
public Cheese makeCheddar(String name) {
return null;
}
@Override
public Cheese makeParmesan(String name, Double fatPercentage) {
return null;
}
@Override
public Cheese makeParmesan(String name) {
return null;
}
}
public class Mozzarella extends Fresh {
/**
* @param name the name.
* @param percentFat the fat.
*/
public Mozzarella(String name, Double percentFat) {
super(name,CheeseType.FRESH, percentFat);
}
@Override
public String toString() {
return "Mozzarella [name=" + getName() + ", type=" + getType() + ", percentFat()="
+ getPercentFat() + "]";
}
}
public class Parmesan extends Firm {
/**
* @param name the name.
* @param percentFat the fat.
*/
public Parmesan(String name, Double percentFat) {
super(name, CheeseType.FIRM, percentFat);
}
@Override
public String toString() {
return "Parmesan [name=" + getName() + ", type=" + getType() + ", percentFat()="
+ getPercentFat() + "]";
}
}
public abstract class Soft extends Cheese {
/**
* Creates a new Fresh.
*
* @param name the name.
* @param type the type.
* @param percenFate the percentage.
*/
protected Soft(String name, CheeseType type, Double percentFat) {
super(name,type,percentFat);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
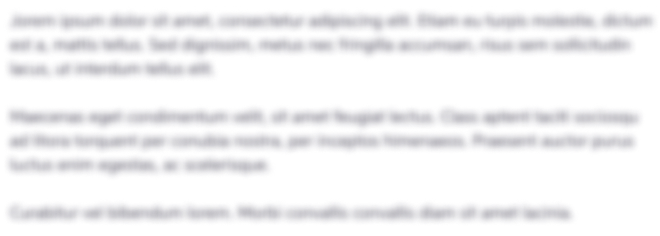
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started