Question
Option 1: Authentication System JAVA coding For security-minded professionals, it is important that only the appropriate people gain access to data in a computer system.
Option 1: Authentication System JAVA coding
For security-minded professionals, it is important that only the appropriate people gain access to data in a computer system. This is called authentication. Once users gain entry, it is also important that they only see data related to their role in a computer system. This is called authorization. For the zoo, you will develop an authentication system that manages both authentication and authorization. You have been given a credentials file that contains credential information for authorized users. You have also been given three files, one for each role: zookeeper, veterinarian, and admin. Each role file describes the data the particular role should be authorized to access. Create an authentication system that does all of the following:
Asks the user for a username
Asks the user for a password
Converts the password using a message digest five (MD5)hashoIt is not required that you write the MD5 from scratch. Use the code located in this document and follow the comments in it to perform this operation.
Checks the credentials against the valid credentials provided in the credentials file. Use the hashed passwords in the second column; the third column contains the actual passwords for testing and the fourth row contains the role of each user.
Limits failed attempts to three before notifying the user and exiting the program
Gives authenticated user access to the correct role file after successful authentication
The system information stored in the role file should be displayed. For example, if a zookeepers credentials is successfully authenticated, then the contents from the zookeeper file will be displayed. If an admins credentials is successfully authenticated, then the contents from the admin file will be displayed.
Allows a user to logout
Stays on the credential screen until either a successful attempt has been made, three unsuccessful attempts have been made, or a user chooses to exit
Uses two classes
Include purpose of comments at the very top of each class as well as documentation comments throughout your code.
1. Create a project called Authentication
2. A .java class called Authentication that contains a main() method.
- This .java class is console driven. That is to say that it will receive its user name and password from the end user via the console.
- It will instantiate (create) a ValidateCredentials object and call the method(s) in it.
Hint #1: Within the credentials file
- User name = field 1 (End user will supply this via the console)
- Encrypted password = field 2
- Unencrypted password = field 3 (End user will supply this via the console)
- The data file name = field 4
Hint #2: Use a try catch block of code within your loop
try
{
validPassWord = ValidateCredentials.isCredentialsValid(userName, passWord);
/* Check to see if validPassWord is successful. If it is then break out of the
loop and exit the program. If not then give the user up to 3 attempts to enter the correct user name and password combination then break out of the loop and exit the program. */
}
catch (exception e)
// Display to the screen the message that is in e.printStackTrace();
// Break out of loop and exit the program.
}
Credentials file :
griffin.keyes 108de81c31bf9c622f76876b74e9285f "alphabet soup" zookeeper
rosario.dawson 3e34baa4ee2ff767af8c120a496742b5 "animal doctor" admin
bernie.gorilla a584efafa8f9ea7fe5cf18442f32b07b "secret password" veterinarian
donald.monkey 17b1b7d8a706696ed220bc414f729ad3 "M0nk3y business" zookeeper
jerome.grizzlybear 3adea92111e6307f8f2aae4721e77900 "grizzly1234" veterinarian
bruce.grizzlybear 0d107d09f5bbe40cade3de5c71e9e9b7 "letmein" admin
zookeeper: Hello, Zookeeper! As zookeeper, you have access to all of the animals' information and their daily monitoring logs. This allows you to track their feeding habits, habitat conditions, and general welfare.
vet: Hello, Veterinarian! As veterinarian, you have access to all of the animals' health records. This allows you to view each animal's medical history and current treatments/illnesses (if any), and to maintain a vaccination log.
admin: Hello, System Admin! As administrator, you have access to the zoo's main computer system. This allows you to monitor users in the system and their roles.
One of the .java classes:
package authentication;
import java.security.MessageDigest;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Scanner;
public class ValidateCredentials {
private boolean isValid;
private String filePath;
private String credentialsFileName;
public ValidateCredentials() {
/* Note:
If you place your .txt datafiles on the same
level as your .java files are at then you will
not need to specify a 'filePath' like:
filePath = "C:\\Users\\...\\Authentication\\";
*/
filePath = "";
isValid = false;
credentialsFileName = "credentials";
}
public boolean isCredentialsValid(String userName, String passWord) throws Exception {
String original = passWord;
MessageDigest md = MessageDigest.getInstance("MD5");
md.update(original.getBytes());
byte[] digest = md.digest();
StringBuffer sb = new StringBuffer();
for (byte b : digest) {
sb.append(String.format("%02x", b & 0xff));
}
System.out.println("");
System.out.println("original:" + original);
System.out.println("digested:" + sb.toString()); //sb.toString() is what you'll need to compare password strings
isValid = readDataFiles(userName, sb.toString());
return isValid;
}
public boolean readDataFiles(String userName, String passWord) throws IOException {
FileInputStream fileByteStream1 = null; // File input stream
FileInputStream fileByteStream2 = null; // File input stream
Scanner inFS1 = null; // Scanner object
Scanner inFS2 = null; // Scanner object
String textLine = null;
String textFileName = null;
boolean foundCredentials = false;
// Try to open file
System.out.println("");
System.out.println("Opening file " + credentialsFileName + ".txt");
fileByteStream1 = new FileInputStream(filePath + "credentials.txt");
inFS1 = new Scanner(fileByteStream1);
System.out.println("");
System.out.println("Reading lines of text.");
while (inFS1.hasNextLine()) {
textLine = inFS1.nextLine();
//System.out.println(textLine);
String[] words = textLine.split("\\s");//splits the string based on whitespace
if (words[0].equals(userName) && textLine.contains(passWord)) {
foundCredentials = true;
int last = words.length - 1;
textFileName = words[last];
break;
}
}
// Done with file, so try to close it
System.out.println("");
System.out.println("Closing file " + credentialsFileName + ".txt");
if (textLine != null) {
fileByteStream1.close(); // close() may throw IOException if fails
}
if (foundCredentials == true) {
// Try to open file
System.out.println("");
System.out.println("Opening file " + textFileName + ".txt");
fileByteStream2 = new FileInputStream(filePath + textFileName + ".txt");
inFS2 = new Scanner(fileByteStream2);
System.out.println("");
while (inFS2.hasNextLine()) {
textLine = inFS2.nextLine();
System.out.println(textLine);
}
// Done with file, so try to close it
System.out.println("");
System.out.println("Closing file " + textFileName + ".txt");
if (textLine != null) {
fileByteStream2.close(); // close() may throw IOException if fails
}
}
return foundCredentials;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
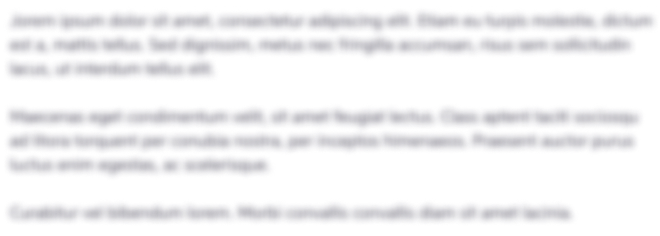
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started