Question
Ordered Linked List Project with insert/remove methods and no duplicate elements. This project is based on one I had finished earlier, it is referenced in
Ordered Linked List Project with insert/remove methods and no duplicate elements.
This project is based on one I had finished earlier, it is referenced in the following assignment paperwork as "Lab24a". It consists of Lab24a.java , MyLinkedList.java and MyList.java .
The changes are mostly made in MyLinkedList.java. All the algorithms are there. Some slight changes are made in Lab24a.java, where the command prompt menu is contained, so it links to the algorithms for remove and insert from there.
I will include my base code and the included text files below the pdf pictures below of my assignment:
Assignment "Project 3":
Here is my finished Lab24a code. There are three java files:
1st-Lab24a.java
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package lab24a; /* * CIT242 Lab24a: Add enhancements to linked list class. */ import java.io.File; import java.io.FileNotFoundException; import java.io.IOException; import java.util.*;
public class Lab24a {
private static boolean verboseMode = false; // Debug aid (== false by default) static String userInput; private static MyLinkedList list;
/** * Output information regarding user commands. */ public static void outputHelpText() {
System.out.println(" COMMAND PARAMETER(S) DESCRIPTION"); System.out.println(" help Output this help text."); System.out.println(" contains string Test if list contains string (incomplete)."); System.out.println(" first string Get the first index of specified string (incomplete)."); System.out.println(" get index Get the list item at specified index (incomplete)."); System.out.println(" last string Get the last index of specified string (incomplete)."); System.out.println(" load filename Read input file into memory."); System.out.println(" print Output memory data to console."); System.out.println(" verbose Turn VERBOSE mode ON or OFF (optional feature)."); System.out.println(" q exit the program."); System.out.println(); } //_________________________________________________________________________ // Main method.
public static void main(String[] args) throws IOException { Scanner input = new Scanner(System.in); System.out.println("This program accepts the following inputs and performs the corresponding actions:"); outputHelpText(); do { System.out.print("Command: "); userInput = input.nextLine(); if (Lab24a.getVerboseMode()) { System.out.printf("userInput=%s ", userInput); } if (userInput.charAt(0) == 'h') { outputHelpText(); } else if (userInput.toUpperCase().equals("VERBOSE")) { Lab24a.setOrClearVerboseMode(); if (Lab24a.getVerboseMode()) { System.out.println("Verbose mode is now ON."); } else { System.out.println("Verbose mode is now OFF."); } } else if (userInput.charAt(0) != 'q') { processCommand(userInput.split("[ ]")); }
} while (userInput.charAt(0) != 'q'); System.out.println("Exit program."); } // end main
static void processCommand(String[] commandArgs) { String command; if (commandArgs.length
}// end processCommand
/** * Read the contents of a text file into an ArrayList of String objects. * * @param fName = file name * @return */ static ArrayList loadFromFile(String fName) { File documentFile = new File(fName); ArrayList contents; contents = new ArrayList(); Scanner input; int lineNum = 0; try { input = new Scanner(documentFile); while (input.hasNextLine()) { String inputText = input.nextLine(); if (Lab24a.getVerboseMode()) { System.out.println("inputText=" + inputText); } lineNum++; contents.add(inputText.trim()); } System.out.printf("%d records loaded. ", lineNum); } catch (FileNotFoundException FNF) { System.out.printf("file not found: %s ", fName); } return contents; }// end loadFromFile
//________________________________________________________________________________________ /** * * @return the current value of the Boolean 'verboseMode' attribute. */ static Boolean getVerboseMode() { return verboseMode; }
//________________________________________________________________________________________ /** * * @param value = new value for the Boolean 'verboseMode' attribute. */ static void setVerboseMode(Boolean value) { verboseMode = value; }
//________________________________________________________________________________________ /** * Turn "verbose mode" ON (true) or OFF (false). */ static void setOrClearVerboseMode() { if (verboseMode) { verboseMode = false; } else { verboseMode = true; } } } // end class Lab24a
2nd- MyLinkedList.java
package lab24a;
public class MyLinkedList implements MyList {
private Node head, tail;
private int size = 0; // Number of elements in the list
/** Create an empty list */
public MyLinkedList() {
}
/** Create a list from an array of objects */
public MyLinkedList(E[] objects) {
for (int i = 0; i
add(objects[i]);
}
/** Return the head element in the list */
public E getFirst() {
if (size == 0) {
return null;
}
else {
return head.element;
}
}
/** Return the last element in the list */
public E getLast() {
if (size == 0) {
return null;
}
else {
return tail.element;
}
}
/** Add an element to the beginning of the list */
public void addFirst(E e) {
Node newNode = new Node(e); // Create a new node
newNode.next = head; // link the new node with the head
head = newNode; // head points to the new node
size++; // Increase list size
if (tail == null) // the new node is the only node in list
tail = head;
}
/** Add an element to the end of the list */
public void addLast(E e) {
Node newNode = new Node(e); // Create a new for element e
if (tail == null) {
head = tail = newNode; // The new node is the only node in list
}
else {
tail.next = newNode; // Link the new with the last node
tail = newNode; // tail now points to the last node
}
size++; // Increase size
}
@Override /** Add a new element at the specified index
* in this list. The index of the head element is 0 */
public void add(int index, E e) {
if (index == 0) {
addFirst(e);
}
else if (index >= size) {
addLast(e);
}
else {
Node current = head;
for (int i = 1; i
current = current.next;
}
Node temp = current.next;
current.next = new Node(e);
(current.next).next = temp;
size++;
}
}
/** Remove the head node and
* return the object that is contained in the removed node. */
public E removeFirst() {
if (size == 0) {
return null;
}
else {
E temp = head.element;
head = head.next;
size--;
if (head == null) {
tail = null;
}
return temp;
}
}
/** Remove the last node and
* return the object that is contained in the removed node. */
public E removeLast() {
if (size == 0) {
return null;
}
else if (size == 1) {
E temp = head.element;
head = tail = null;
size = 0;
return temp;
}
else {
Node current = head;
for (int i = 0; i
current = current.next;
}
E temp = tail.element;
tail = current;
tail.next = null;
size--;
return temp;
}
}
@Override /** Remove the element at the specified position in this
* list. Return the element that was removed from the list. */
public E remove(int index) {
if (index = size) {
return null;
}
else if (index == 0) {
return removeFirst();
}
else if (index == size - 1) {
return removeLast();
}
else {
Node previous = head;
for (int i = 1; i
previous = previous.next;
}
Node current = previous.next;
previous.next = current.next;
size--;
return current.element;
}
}
@Override /** Override toString() to return elements in the list */
public String toString() {
StringBuilder result = new StringBuilder("[");
Node current = head;
for (int i = 0; i
result.append(current.element);
current = current.next;
if (current != null) {
result.append(", "); // Separate two elements with a comma
}
else {
result.append("]"); // Insert the closing ] in the string
}
}
return result.toString();
}
@Override /** Clear the list */
public void clear() {
size = 0;
head = tail = null;
}
//WORKING ON
@Override /** Return true if this list contains the element e */
public boolean contains(Object e) {
Node temp = head;
while(temp != null)
{
if(e.equals(temp.element))
{
return true;
}
temp = temp.next;
}
return false;
}
@Override /** Return the element at the specified index */
public E get(int index) {
Node temp = head;
if (index = size) {
return null;
}
for (int i = 1; i
temp = temp.next;
}
return temp.element;
}
@Override /** Return the index of the head matching element in
* this list. Return -1 if no match. */
public int indexOf(Object e) {
Node temp = head;
for (int i = 0; i
// If e is null
if (e == null) // look for a null element in the list
{
if (temp.element == null) {
return i;
}
} else {// e is an object (use equals)
if (e.equals(temp.element)) {
return i;
}
}
temp = temp.next;
}
// if we get here, e is not in the list
return -1;
}
@Override /** Return the index of the last matching element in
* this list. Return -1 if no match. */
public int lastIndexOf(E e) {
Node temp = head;
int index = 0, highest = -1;
while(temp != null){
if(temp.element.equals(e))
highest = index;
index++;
temp = temp.next;
}
return highest;
}
@Override /** Replace the element at the specified position
* in this list with the specified element. */
public E set(int index, E e) {
// Left as an exercise
return null;
}
@Override /** Override iterator() defined in Iterable */
public java.util.Iterator iterator() {
return new LinkedListIterator();
}
private class LinkedListIterator
implements java.util.Iterator {
private Node current = head; // Current index
@Override
public boolean hasNext() {
return (current != null);
}
@Override
public E next() {
E e = current.element;
current = current.next;
return e;
}
@Override
public void remove() {
// Left as an exercise
}
}
private static class Node {
E element;
Node next;
public Node(E element) {
this.element = element;
}
}
@Override /** Return the number of elements in this list */
public int size() {
return size;
}
}
3rd-MyList.java
package lab24a;
import java.util.Collection;
public interface MyList extends Collection { /** Add a new element at the specified index in this list */ public void add(int index, E e);
/** Return the element from this list at the specified index */ public E get(int index);
/** Return the index of the first matching element in this list. * Return -1 if no match. */ public int indexOf(Object e);
/** Return the index of the last matching element in this list * Return -1 if no match. */ public int lastIndexOf(E e);
/** Remove the element at the specified position in this list * Shift any subsequent elements to the left. * Return the element that was removed from the list. */ public E remove(int index);
/** Replace the element at the specified position in this list * with the specified element and returns the new set. */ public E set(int index, E e); @Override /** Add a new element at the end of this list */ public default boolean add(E e) { add(size(), e); return true; }
@Override /** Return true if this list contains no elements */ public default boolean isEmpty() { return size() == 0; }
@Override /** Remove the first occurrence of the element e * from this list. Shift any subsequent elements to the left. * Return true if the element is removed. */ public default boolean remove(Object e) { if (indexOf(e) >= 0) { remove(indexOf(e)); return true; } else return false; }
@Override public default boolean containsAll(Collection c) { // Left as an exercise return true; }
@Override public default boolean addAll(Collection c) { // Left as an exercise return true; }
@Override public default boolean removeAll(Collection c) { // Left as an exercise return true; }
@Override public default boolean retainAll(Collection c) { // Left as an exercise return true; }
@Override public default Object[] toArray() { // Left as an exercise return null; }
@Override public default T[] toArray(T[] array) { // Left as an exercise return null; } }
THREE TEXT FILES TO TEST WITH
Cities.txt
Boston Paris Philadelphia Miami London Paris Chicago
firstTen.txt
Washington Adams Jefferson Madison Monroe Adams Jackson VanBuren Harrison Tyler
names.txt
Tom Susan Kim George James Jean George Jane Denise Jenny Kathy Jane
Thank you! and Take Care!
PS- if you are curious for Lab24a information, here is a link:
https://www.chegg.com/homework-help/questions-and-answers/desperately-need-figure-lab-stuck-dozens-hours-weeks--tried-post-question-screen-froze-90--q30316247
I ended up finishing the project before the answer was posted so my code here is different, but the exercise assignment is posted there and other info.
CIT242 Data Structures Programming Project 3 ue date: Monday, Jul The due date for this programming project is Monday, July 9,2018 Be sure that you read and understand this entire document before you start writing any code. Also be sure that you have completed Lab24a Ordered Linked List The objective of this project is to enhance the linked list program (specifically, your solution code for Lab24a) to maintain the linked list in sorted (alphabetical) order The new program will also not allow the list to have duplicate nodes: if the input data contains duplicate values, then the program w increment a nodeCount field in the corresponding Node object. The starting code that you must use is your solution to Lab24a Changes to the NodeStep by Step Solution
There are 3 Steps involved in it
Step: 1
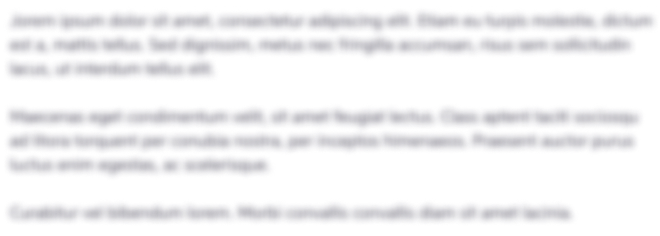
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started