Question
Original question: I need a program that simulates a flower pack with the traits listed below: - You must use a LinkedList for storage (not
Original question:
I need a program that simulates a flower pack with the traits listed below:
- You must use a LinkedList for storage (not an Arrray list).
- You must be able to display, add, remove, sort, filter, and analyze information from our flower pack.
- The flower pack should hold flowers, weeds, fungus, and herbs. All should be a subclass of a plant class.
- Each subclass shares certain qualities (ID, Name, and Color) plant class
- Flower traits include (Thorns, and Smell)
- Fungus traits include (Poisonous)
- Weed traits include (Poisonous, Edible and Medicinal)
- Herb traits include (Flavor, Medicinal, Seasonal)
No GUI is required.
The code I have is listed below, it appears to do just about everything I need it to. The one part of the instructions I am unclear on is when it says we need to be able to analyze information from our flower pack.
Here are the instructions regarding analyzing information from our flower pack:
Analysis can be case sensitive, if you so desire. Remember - you MUST use recursion to solve this problem. Meaning not a single loop should be called when doing these calculations. You CAN use a loop when you want to move from analyzing one flower to the next, but your loop CANNOT be used when analyzing a specific flower.
Analysis Examples:
- How you display the results is up to you
- Analyze 3 different strings such as ar, ne, and um which strings is up to you and does not require user input
How do I modify my code to analyze information from the flower pack according to the above instructions (with use of recursion)? Thank you.
Here is the code I have:
PlantDriver.java ----------------------------
import java.util.*; import java.io.*; public class PlantDriver { public static void main(String[] args) throws IOException { new PlantDriver(); } public PlantDriver() throws IOException { Scanner input = new Scanner(System.in); LinkedList
} private void searchPlants(LinkedList
------------------------------------------------------------ Flower.java ------------------------------------ public class Flower extends Plant { private boolean hasThorns; private String smell;
public Flower(String flowerColor, String flowerID, String flowerName, String smell, boolean hasThorns) { super(flowerColor, flowerID, flowerName); this.hasThorns = hasThorns; this.smell = smell; }
public void setSmell(String flowerSmell) { this.smell = flowerSmell; }
public void setThorns(boolean isThorny) { this.hasThorns = isThorny; }
public String getSmell() { return smell; }
public boolean getIsThorny() { return hasThorns; }
public String toString() { return "This flower is colored: " + super.getColor() + " with a name of " + super.getName() + " and an ID of " + super.getID() + " the smell is " + this.smell + " and it is thorny = " + this.hasThorns; }
@Override public boolean equals(Object otherFlower) { if (otherFlower == null) { return false; }
if (!Flower.class.isAssignableFrom(otherFlower.getClass())) { return false; }
Flower other = (Flower) otherFlower; if (!(this.getName().equals(other.getName()))) { return false; }
if (!(this.getColor().equals(other.getColor()))) { return false; }
if ((this.getSmell() != (other.getSmell()))) { return false; }
if (!(this.getID().equals(other.getID()))) { return false; }
if(this.getIsThorny() != other.getIsThorny()) { return false; }
return true; } } ------------------------------------------------------------ Fungus.java ------------------------------------ public class Fungus extends Plant{
private boolean isPoisonous;
public Fungus(String fungusColor, String fungusID, String fungusName, boolean isPoisonous) { super(fungusColor, fungusID, fungusName); this.isPoisonous = isPoisonous; }
public void setIsPoisonous(boolean isPoisonous) { this.isPoisonous = isPoisonous; }
public boolean getIsPoisonous(){ return isPoisonous; }
public String toString() { return "This fungus as sampled is " + this.getColor() + " in color " + " with an ID of " + this.getID() + " and a name of " + this.getName() + " and it's poisonous = " + this.getIsPoisonous(); }
@Override public boolean equals(Object otherFungus) {
if (otherFungus == null) { return false; } if (!Fungus.class.isAssignableFrom(otherFungus.getClass())) { return false; }
Fungus other = (Fungus) otherFungus;
if (!(this.getColor().equals(other.getColor()))) { return false; }
if (!(this.getID().equals(other.getID()))) { return false;
}
if (!(this.getName().equals(other.getName()))) { return false; }
if (this.isPoisonous != other.isPoisonous) { return false; }
return true; }
} ------------------------------------------------------------ Herb.java ------------------------------------ public class Herb extends Plant{ private String flavor; private boolean isMedicinal, isSeasonal;
public Herb(String herbColor, String herbID, String herbName, String flavor, boolean isMedicinal, boolean isSeasonal) { super(herbColor, herbID, herbName); this.flavor = flavor; this.isMedicinal = isMedicinal; this.isSeasonal = isSeasonal; }
public void setHerbFlavor(String herbFlavor) { this.flavor = herbFlavor; }
public String getHerbFlavor() { return flavor; }
public void setMedicinalProperty(boolean isMedicinal) { this.isMedicinal = isMedicinal; }
public boolean getMedicinalProperty() { return isMedicinal; }
public void setSeasonalProperty(boolean isSeasonal) { this.isSeasonal = isSeasonal; }
public boolean getSeasonalProperty() { return isSeasonal; }
public String toString() { return "This herb is of " + this.getColor() + " color and is called " + this.getName() + " with an ID of " + this.getID() + " with a " + this.getHerbFlavor() + " flavor and " + " with a seasonal property of " + this.getSeasonalProperty() + " and a Medicinal Property of " + this.getMedicinalProperty(); }
@Override public boolean equals(Object otherHerb) { if(otherHerb == null) { return false; }
if(!Herb.class.isAssignableFrom(otherHerb.getClass())) { return false; }
Herb other = (Herb) otherHerb;
if (!this.flavor.equals(other.flavor)) { return false; }
if (!this.getColor().equals(other.getColor())) { return false; }
if (!this.getID().equals(other.getID())) { return false; }
if (!this.getName().equals(other.getName())) { return false; }
if (this.isMedicinal != other.isMedicinal) { return false; }
if (this.isSeasonal != other.isSeasonal) { return false; }
return true;
} } ------------------------------------------------------------ Plant.java ------------------------------------ import java.util.*;
public class Plant {
private String id; private String name; private String color;
public Plant() { this.id = ""; this.name = ""; this.color = ""; }
public Plant(String plantColor, String plantID, String plantName) { this.id = plantID; this.color = plantColor; this.name = plantName; }
public void setID(String plantID) { this.id = plantID; }
public void setColor(String plantColor) { this.color = plantColor; }
public void setName(String plantName) { this.name = plantName; }
public String getName() { return name; }
public String getColor() { return color; }
public String getID() { return id; }
public String toString() { return "This plant's name is " + this.getName() + " with a color of: " + this.getColor() + " with a unique ID of: " + this.getID(); }
@Override public boolean equals(Object otherPlant) { if (otherPlant == null) { return false; }
if (!Plant.class.isAssignableFrom(otherPlant.getClass())) { return false; }
final Plant other = (Plant) otherPlant;
if ((this.name == null) ? (other.name != null) : !this.name.equals(other.name)) { return false; } if (!(this.color.equals(other.color))) { return false; }
if (!(this.id.equals(other.id))) { return false; }
return true; }
} ------------------------------------------------------------ Weed.java ------------------------------------ import java.util.*; public class Weed extends Plant { private boolean isEdible, isMedicinal, isPoisonous;
public Weed(String weedColor, String weedID, String weedName, boolean isEdible, boolean isMedicinal, boolean isPoisonous) { super(weedColor, weedID, weedName); this.isEdible = isEdible; this.isMedicinal = isMedicinal; this.isPoisonous = isPoisonous; }
public void setIsEdible(boolean isEdible) { this.isEdible = isEdible; }
public boolean getIsEdible() { return isEdible; }
public void setIsMedicinal(boolean isMedicinal) { this.isMedicinal = isMedicinal; }
public boolean getIsMedicinal() { return isMedicinal; }
public void setIsPoisonous(boolean isPoisonous) { this.isPoisonous = isPoisonous; }
public boolean getIsPoisonous() { return isPoisonous; }
public String toString() { return "This weed is of " + this.getColor() + " color and is called " + this.getName() + " with an ID of " + this.getID() + " it is edible = " + this.getIsEdible() + " and it is Poisonous " + this.getIsPoisonous() + " and it is medicinal " + this.getIsMedicinal(); }
@Override public boolean equals(Object otherWeed) { if (otherWeed == null) { return false; }
if (!(Weed.class.isAssignableFrom(otherWeed.getClass()))) { return false; }
Weed other = (Weed) otherWeed;
if (!(this.getID().equals(other.getID()))) { return false; }
if (!(this.getName().equals(other.getName()))) { return false; }
if (!(this.getColor().equals(other.getColor()))) { return false; }
if (this.isEdible != other.isEdible) { return false; }
if (this.isMedicinal != other.isMedicinal) { return false; }
if (this.isPoisonous != other.isPoisonous) { return false; }
return true;
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
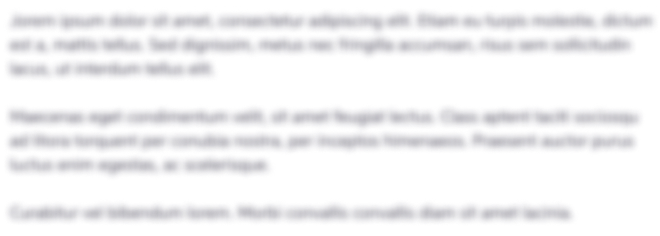
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started