Question
OUTPUTMake sure that your program works at least with this scenario. Please enter a number of rows for an classroom seating. 3 Please enter a
OUTPUTMake sure that your program works at least with this scenario.
Please enter a number of rows for an classroom seating. 3 Please enter a number of columns for an classroom seating. 3 Please enter a student information or enter "Q" to quit. Mickey-Mouse A student information is read. Mickey-Mouse Please enter a row number where the student wants to sit. 1 Please enter a column number where the student wants to sit. 2 The seat at row 1 and column 2 is assigned to the student M-M. The current seating -------------------- *-*. *-*. *-*. *-*. *-*. M-M. *-*. *-*. *-*. Please enter a student information or enter "Q" to quit. Daisy-Duck A student information is read. Daisy-Duck Please enter a row number where the student wants to sit. 2 Please enter a column number where the student wants to sit. 0 The seat at row 2 and column 0 is assigned to the student D-D. The current seating -------------------- *-*. *-*. *-*. *-*. *-*. M-M. D-D. *-*. *-*. Please enter a student information or enter "Q" to quit. Clarabelle-Cow A student information is read. Clarabelle-Cow Please enter a row number where the student wants to sit. 2 Please enter a column number where the student wants to sit. 1 The seat at row 2 and column 1 is assigned to the student C-C. The current seating -------------------- *-*. *-*. *-*. *-*. *-*. M-M. D-D. C-C. *-*. Please enter a student information or enter "Q" to quit. Max-Goof A student information is read. Max-Goof Please enter a row number where the student wants to sit. 0 Please enter a column number where the student wants to sit. 0 The seat at row 0 and column 0 is assigned to the student M-G. The current seating -------------------- M-G. *-*. *-*. *-*. *-*. M-M. D-D. C-C. *-*. Please enter a student information or enter "Q" to quit. Horace-Horsecollar A student information is read. Horace-Horsecollar Please enter a row number where the student wants to sit. 5 Please enter a column number where the student wants to sit. 1 row or column number is not valid. A student Horace Horsecollar is not assigned a seat. Please enter a student information or enter "Q" to quit. Sylvester-Shyster A student information is read. Sylvester-Shyster Please enter a row number where the student wants to sit. 2 Please enter a column number where the student wants to sit. 0 The seat at row 2 and column 0 is taken. Please enter a student information or enter "Q" to quit. Snow-White A student information is read. Snow/White Please enter a row number where the student wants to sit. -1 Please enter a column number where the student wants to sit. 0 row or column number is not valid. A student Snow White is not assigned a seat. Please enter a student information or enter "Q" to quit. Jiminy-Criket A student information is read. Jiminy-Criket Please enter a row number where the student wants to sit. 0 Please enter a column number where the student wants to sit. 2 The seat at row 0 and column 2 is assigned to the student J-C. The current seating -------------------- M-G. *-*. J-C. *-*. *-*. M-M. D-D. C-C. *-*. Please enter a student information or enter "Q" to quit. Q
CODE
#include struct student { char last_name[30] ; char first_name[30]; }; struct classroom_seating { struct student **seating; }; void student_init_default (struct student *g ) {} void student_init (struct student *g, char *info) {} void student_to_string (struct student *g ) {} void classroom_seating_init (int rowNum, int columnNum, struct classroom_seating *a ) {} int assign_student_at (int row, int col, struct classroom_seating *a, struct student* g) {} int check_boundaries (int row, int col, struct classroom_seating *a) {} void classroom_seating_to_string (struct classroom_seating *a ) {} void main() { struct classroom_seating classroom_seating; struct student temp_student; int row, col, rowNum, columnNum; char student_info[30]; // Ask a user to enter a number of rows for an classroom seating printf ("Please enter a number of rows for an classroom seating."); scanf ("%d", &rowNum); // Ask a user to enter a number of columns for an classroom seating printf ("Please enter a number of columns for an classroom seating."); scanf ("%d", &columnNum); // classroom_seating classroom_seating_init(rowNum, columnNum, &classroom_seating); printf("Please enter a student information or enter \"Q\" to quit."); /*** reading a student's information ***/ scanf ("%s", student_info); /* we will read line by line **/ while (1 /* change this condition*/ ){ printf (" A student information is read."); // printing information. printf ("%s", student_info); // student student_init (&temp_student, student_info); // Ask a user to decide where to seat a student by asking // for row and column of a seat printf ("Please enter a row number where the student wants to sit."); scanf("%d", &row); printf("Please enter a column number where the student wants to sit."); scanf("%d", &col); // Checking if the row number and column number are valid // (exist in the theatre that we created.) if (check_boundaries(row, col, &classroom_seating) == 0) { printf(" row or column number is not valid."); printf("A student %s %s is not assigned a seat.", temp_student.first_name, temp_student.last_name); } else { // Assigning a seat for a student if (assign_student_at(row, col, &classroom_seating, &temp_student) == 1){ printf(" The seat at row %d and column %d is assigned to the student",row, col); student_to_string(&temp_student); classroom_seating_to_string(&classroom_seating); } else { printf(" The seat at row %d and column %d is taken.", row, col); } } // Read the next studentInfo printf ("Please enter a student information or enter \"Q\" to quit."); /*** reading a student's information ***/ scanf("%s", student_info); } }
IMPORTANT NOTE:
PLEASE WRITE THE COMMENTS FOR EACH LINE OF THE CODE, AND PLEASE USE THE ORIGINAL CODE THAT I GIVE TO YOU. THANK YOU VERY MUCH.
4. Part 2 Structs and Arrays (65 points). In this assignment, we will be making a program that reads in student's information and create a classroom seating arrangement with a number of rows and columns specified by a user. Then it will attempt to assign each student to a seat in an classroom. Use the file homework part 2.c (attached at the end of this document). Complete the file and include all the following requested code in the file homework part 2.c Step 1 First, you need to create a structure student. It should contain two variables, last _name (char [30]) and first name (char [30]). In addition, the following functions should be defined. Function void student init default (struct student *s) Description Assign the default string" ***" to both variables, last name and first name. void student init (struct student *s, char *info) Use the strtok function to extract first name and last name from the variable student, then assign them to each instance variable of the student structure. An example of the input string is David-Johnson void student to string (struct student *s) It prints the initial character of the first name, a dash, the initial character of the last name, and a period. An example of such string for the student David Johnson is: D-J Step 2. You will be creating a structure called classroom seating in the same code file. The structure classroom scating will contain a 2-dimensional array called "seating" of student type. Define the following functions: Description It instantiates a two-dimensional array of the size "rowNum" by "columnNum" specified by the Function void classroom seating init (int rowNum, int columnNum, struct classroom_seating *a) meters inside the struct a. Then it initializes each para student element of this array using the student init default function. So, each student will have default values for its instance variables. int assign student _at (int row, int col, struct classroom seating *a, The function attempts to assign the "s" to the seat at "row" and "col" (specified by the parameters of this function). If the seat has a default student, i.e., a student 4. Part 2 Structs and Arrays (65 points). In this assignment, we will be making a program that reads in student's information and create a classroom seating arrangement with a number of rows and columns specified by a user. Then it will attempt to assign each student to a seat in an classroom. Use the file homework part 2.c (attached at the end of this document). Complete the file and include all the following requested code in the file homework part 2.c Step 1 First, you need to create a structure student. It should contain two variables, last _name (char [30]) and first name (char [30]). In addition, the following functions should be defined. Function void student init default (struct student *s) Description Assign the default string" ***" to both variables, last name and first name. void student init (struct student *s, char *info) Use the strtok function to extract first name and last name from the variable student, then assign them to each instance variable of the student structure. An example of the input string is David-Johnson void student to string (struct student *s) It prints the initial character of the first name, a dash, the initial character of the last name, and a period. An example of such string for the student David Johnson is: D-J Step 2. You will be creating a structure called classroom seating in the same code file. The structure classroom scating will contain a 2-dimensional array called "seating" of student type. Define the following functions: Description It instantiates a two-dimensional array of the size "rowNum" by "columnNum" specified by the Function void classroom seating init (int rowNum, int columnNum, struct classroom_seating *a) meters inside the struct a. Then it initializes each para student element of this array using the student init default function. So, each student will have default values for its instance variables. int assign student _at (int row, int col, struct classroom seating *a, The function attempts to assign the "s" to the seat at "row" and "col" (specified by the parameters of this function). If the seat has a default student, i.e., a studentStep by Step Solution
There are 3 Steps involved in it
Step: 1
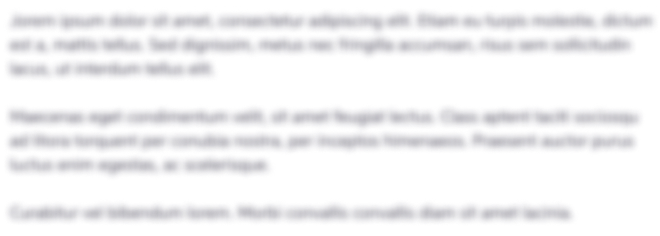
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started