Question
Overview Would you take a job where your starting pay is a random amount between $0.01 and $0.05, inclusive? How about if you could only
Overview
Would you take a job where your starting pay is a random amount between $0.01 and $0.05, inclusive? How about if you could only work a random number of days (between 1 and 30, inclusive)? If your answer is "no" to either of those questions, what if your pay doubled each day that you worked? Would you take the job now?
For this assignment, write a program that will calculate how much a person would earn over a finite (random) period of time if his/her salary is a random amount for the first day and continues to double each day for three different jobs.
So if a person's starting salary is $0.04, they would earn that $0.04 for the first day of work, $0.08 for the second day of work, $0.16 for the third day of work, etc.... Over three days, the person would earn $0.28.
Random Number Generation
In the first few programs, the user has been asked for input. This program will be different. Rather than asking the user for input, the random number generator will be used to determine the starting salary and the number of days worked.
To use the random number generator, first add a #include statement for the cstdlib library to the top of the program:
#include
Next, initialize the random number generator. This is done by calling the srand function and passing in an integer value (known as a seed value). This should only be done ONE time and it MUST be done before actually getting a random number. A value of 1 (or any integer literal) will generate the same sequence of "random" numbers every time the program is executed. This can be useful for debugging:
srand(1);
To get a different series of random numbers each time the program is run, the actual time that the program is run can be passed as the seed value for the random number generator:
srand(time(0));
If the time function is used, make sure to #include the ctime library as well.
Finally, generate a random number by calling the rand function:
num = rand();
The above line of C++ code will generate a "random" integer between 0 and RAND_MAX and saves the value in an integer variable named num. RAND_MAX is a pre-defined constant that is equal to the maximum possible random number. It is implementation dependent but is guaranteed to be at least 32,767.
Modulus division can be used to restrict the "random" integer to a smaller range:
num = rand() % 7;
will produce a value between 0 and 6. To change the range to 1 through 7, simply add 1:
num = rand() % 7 + 1;
Note for Mac users: the sequence of random numbers that is generated on a Mac will likely be different than those generated on a Windows machine. This means that the output produced by a program running on a Mac will likely be different than the output produced by a program running on Windows.
Basic Program Logic
Use a value of 10 to seed the random number generator.
For the first job, generate a random number between 1 and 5. This will be used to determine the user's starting salary. A value of 1 sets the starting salary to $0.01, 2 sets the starting salary to $0.02, 3 to $0.03, etc...
Generate another random number. This time is should be between 1 and 30. It will represent the number of days to be worked.
Display the starting salary and the number of days to be worked.
In a for loop that executes exactly one time for each day to be worked, calculate and display the user's salary for each day, and update the user's total pay.
After the for loop has finished executing, display the user's total pay and the number of days that it took to earn the pay.
For the second job, repeat the basic process that was laid out for Job 1. However, rather than using a for loop, use a while loop.
For the third job, repeat the basic process that was laid out for Job 1. However, rather than using a for loop, use a do while loop.
Symbolic Constants
This program requires the use of 3 symbolic constants. One of the constants is pre-defined and the remaining 2 must be defined by you.
Pre-defined constant
RAND_MAX is a symbolic constant that holds the maximum possible random number that is available on the system that is executing the program. At a minimum, the value will be 32767. Make sure to add #include to the top of the program. DO NOT declare this constant in the program.
Constants that need to be created/defined
The first constant is an integer that represents the maximum number of days that will be worked. The value should be 30.
The second constant is an integer that represents the maximum starting salary. The value should be 5.
Programming Requirements
Make sure to seed the random number generator by calling the srand function. This MUST be done in main before calling the rand function. The file that is handed in for grading must use srand(10);.
Include line documentation. There is no need to document every single line, but logical "chunks" of code should be preceded by a line or two that describes what the "chunk" of code does. This will be a part of every program that is submitted during the semester and this will be the last reminder in the program write-ups.
To use the random number generator, add two additional #includes: #include
The dollar amounts should be displayed with exactly 2 digits after the decimal point.
Hand in a copy of your source code (the CPP file) using Blackboard.
Output
Run 1 (using srand(10);)
***** JOB 1 ***** Starting Salary: $0.02 Number of days to work: 10 Day 1: 0.02 Day 2: 0.04 Day 3: 0.08 Day 4: 0.16 Day 5: 0.32 Day 6: 0.64 Day 7: 1.28 Day 8: 2.56 Day 9: 5.12 Day 10: 10.24 For Job 1, you earned $20.46 in 10 day(s). ***** JOB 2 ***** Starting Salary: $0.03 Number of days to work: 15 Day 1: 0.03 Day 2: 0.06 Day 3: 0.12 Day 4: 0.24 Day 5: 0.48 Day 6: 0.96 Day 7: 1.92 Day 8: 3.84 Day 9: 7.68 Day 10: 15.36 Day 11: 30.72 Day 12: 61.44 Day 13: 122.88 Day 14: 245.76 Day 15: 491.52 For Job 2, you earned $983.01 in 15 day(s). ***** JOB 3 ***** Starting Salary: $0.03 Number of days to work: 27 Day 1: 0.03 Day 2: 0.06 Day 3: 0.12 Day 4: 0.24 Day 5: 0.48 Day 6: 0.96 Day 7: 1.92 Day 8: 3.84 Day 9: 7.68 Day 10: 15.36 Day 11: 30.72 Day 12: 61.44 Day 13: 122.88 Day 14: 245.76 Day 15: 491.52 Day 16: 983.04 Day 17: 1966.08 Day 18: 3932.16 Day 19: 7864.32 Day 20: 15728.64 Day 21: 31457.28 Day 22: 62914.56 Day 23: 125829.12 Day 24: 251658.24 Day 25: 503316.48 Day 26: 1006632.96 Day 27: 2013265.92 For Job 3, you earned $4026531.81 in 27 day(s).
Run 2 (using srand(time(0));)
***** JOB 1 ***** Starting Salary: $0.02 Number of days to work: 16 Day 1: 0.02 Day 2: 0.04 Day 3: 0.08 Day 4: 0.16 Day 5: 0.32 Day 6: 0.64 Day 7: 1.28 Day 8: 2.56 Day 9: 5.12 Day 10: 10.24 Day 11: 20.48 Day 12: 40.96 Day 13: 81.92 Day 14: 163.84 Day 15: 327.68 Day 16: 655.36 For Job 1, you earned $1310.70 in 16 day(s). ***** JOB 2 ***** Starting Salary: $0.02 Number of days to work: 24 Day 1: 0.02 Day 2: 0.04 Day 3: 0.08 Day 4: 0.16 Day 5: 0.32 Day 6: 0.64 Day 7: 1.28 Day 8: 2.56 Day 9: 5.12 Day 10: 10.24 Day 11: 20.48 Day 12: 40.96 Day 13: 81.92 Day 14: 163.84 Day 15: 327.68 Day 16: 655.36 Day 17: 1310.72 Day 18: 2621.44 Day 19: 5242.88 Day 20: 10485.76 Day 21: 20971.52 Day 22: 41943.04 Day 23: 83886.08 Day 24: 167772.16 For Job 2, you earned $335544.30 in 24 day(s). ***** JOB 3 ***** Starting Salary: $0.01 Number of days to work: 27 Day 1: 0.01 Day 2: 0.02 Day 3: 0.04 Day 4: 0.08 Day 5: 0.16 Day 6: 0.32 Day 7: 0.64 Day 8: 1.28 Day 9: 2.56 Day 10: 5.12 Day 11: 10.24 Day 12: 20.48 Day 13: 40.96 Day 14: 81.92 Day 15: 163.84 Day 16: 327.68 Day 17: 655.36 Day 18: 1310.72 Day 19: 2621.44 Day 20: 5242.88 Day 21: 10485.76 Day 22: 20971.52 Day 23: 41943.04 Day 24: 83886.08 Day 25: 167772.16 Day 26: 335544.32 Day 27: 671088.64 For Job 3, you earned $1342177.27 in 27 day(s).
Run 3 (using srand(time(0));)
***** JOB 1 ***** Starting Salary: $0.03 Number of days to work: 22 Day 1: 0.03 Day 2: 0.06 Day 3: 0.12 Day 4: 0.24 Day 5: 0.48 Day 6: 0.96 Day 7: 1.92 Day 8: 3.84 Day 9: 7.68 Day 10: 15.36 Day 11: 30.72 Day 12: 61.44 Day 13: 122.88 Day 14: 245.76 Day 15: 491.52 Day 16: 983.04 Day 17: 1966.08 Day 18: 3932.16 Day 19: 7864.32 Day 20: 15728.64 Day 21: 31457.28 Day 22: 62914.56 For Job 1, you earned $125829.09 in 22 day(s). ***** JOB 2 ***** Starting Salary: $0.05 Number of days to work: 3 Day 1: 0.05 Day 2: 0.10 Day 3: 0.20 For Job 2, you earned $0.35 in 3 day(s). ***** JOB 3 ***** Starting Salary: $0.05 Number of days to work: 7 Day 1: 0.05 Day 2: 0.10 Day 3: 0.20 Day 4: 0.40 Day 5: 0.80 Day 6: 1.60 Day 7: 3.20 For Job 3, you earned $6.35 in 7 day(s).
Extra Credit 1
For up to 5 points of extra credit, add code that will display the total amount that the user has earned each day along with the daily salary. The values should be displayed in neat columns.
Note about extra credit: the points will ONLY be awarded if the required portions of the assignment work correctly. In other words, don't take short cuts in the rest of the program because it is assumed that 5 extra points will be awarded.
Extra Credit 1 Output
***** JOB 1 ***** Starting Salary: $0.02 Number of days to work: 10 Daily Salary Amount Earned Day 1: 0.02 0.02 Day 2: 0.04 0.06 Day 3: 0.08 0.14 Day 4: 0.16 0.30 Day 5: 0.32 0.62 Day 6: 0.64 1.26 Day 7: 1.28 2.54 Day 8: 2.56 5.10 Day 9: 5.12 10.22 Day 10: 10.24 20.46 For Job 1, you earned $20.46 in 10 day(s). ***** JOB 2 ***** Starting Salary: $0.03 Number of days to work: 15 Daily Salary Amount Earned Day 1: 0.03 0.03 Day 2: 0.06 0.09 Day 3: 0.12 0.21 Day 4: 0.24 0.45 Day 5: 0.48 0.93 Day 6: 0.96 1.89 Day 7: 1.92 3.81 Day 8: 3.84 7.65 Day 9: 7.68 15.33 Day 10: 15.36 30.69 Day 11: 30.72 61.41 Day 12: 61.44 122.85 Day 13: 122.88 245.73 Day 14: 245.76 491.49 Day 15: 491.52 983.01 For Job 2, you earned $983.01 in 15 day(s). ***** JOB 3 ***** Starting Salary: $0.03 Number of days to work: 27 Daily Salary Amount Earned Day 1: 0.03 0.03 Day 2: 0.06 0.09 Day 3: 0.12 0.21 Day 4: 0.24 0.45 Day 5: 0.48 0.93 Day 6: 0.96 1.89 Day 7: 1.92 3.81 Day 8: 3.84 7.65 Day 9: 7.68 15.33 Day 10: 15.36 30.69 Day 11: 30.72 61.41 Day 12: 61.44 122.85 Day 13: 122.88 245.73 Day 14: 245.76 491.49 Day 15: 491.52 983.01 Day 16: 983.04 1966.05 Day 17: 1966.08 3932.13 Day 18: 3932.16 7864.29 Day 19: 7864.32 15728.61 Day 20: 15728.64 31457.25 Day 21: 31457.28 62914.53 Day 22: 62914.56 125829.09 Day 23: 125829.12 251658.21 Day 24: 251658.24 503316.45 Day 25: 503316.48 1006632.93 Day 26: 1006632.96 2013265.89 Day 27: 2013265.92 4026531.81 For Job 3, you earned $4026531.81 in 27 day(s).
Extra Credit 2
For up to 5 points of extra credit, add a cascading decision statement to determine which job paid the most money and display a message that tells the user which job paid the most.
Extra Credit 1 DOES NOT have to be attempted to receive the extra credit points for Extra Credit 2.
Note about extra credit: the points will ONLY be awarded if the required portions of the assignment work correctly. In other words, don't take short cuts in the rest of the program because it is assumed that 5 extra points will be awarded.
Extra Credit 2 Output
***** JOB 1 ***** Starting Salary: $0.02 Number of days to work: 10 Daily Salary Amount Earned Day 1: 0.02 0.02 Day 2: 0.04 0.06 Day 3: 0.08 0.14 Day 4: 0.16 0.30 Day 5: 0.32 0.62 Day 6: 0.64 1.26 Day 7: 1.28 2.54 Day 8: 2.56 5.10 Day 9: 5.12 10.22 Day 10: 10.24 20.46 For Job 1, you earned $20.46 in 10 day(s). ***** JOB 2 ***** Starting Salary: $0.03 Number of days to work: 15 Daily Salary Amount Earned Day 1: 0.03 0.03 Day 2: 0.06 0.09 Day 3: 0.12 0.21 Day 4: 0.24 0.45 Day 5: 0.48 0.93 Day 6: 0.96 1.89 Day 7: 1.92 3.81 Day 8: 3.84 7.65 Day 9: 7.68 15.33 Day 10: 15.36 30.69 Day 11: 30.72 61.41 Day 12: 61.44 122.85 Day 13: 122.88 245.73 Day 14: 245.76 491.49 Day 15: 491.52 983.01 For Job 2, you earned $983.01 in 15 day(s). ***** JOB 3 ***** Starting Salary: $0.03 Number of days to work: 27 Daily Salary Amount Earned Day 1: 0.03 0.03 Day 2: 0.06 0.09 Day 3: 0.12 0.21 Day 4: 0.24 0.45 Day 5: 0.48 0.93 Day 6: 0.96 1.89 Day 7: 1.92 3.81 Day 8: 3.84 7.65 Day 9: 7.68 15.33 Day 10: 15.36 30.69 Day 11: 30.72 61.41 Day 12: 61.44 122.85 Day 13: 122.88 245.73 Day 14: 245.76 491.49 Day 15: 491.52 983.01 Day 16: 983.04 1966.05 Day 17: 1966.08 3932.13 Day 18: 3932.16 7864.29 Day 19: 7864.32 15728.61 Day 20: 15728.64 31457.25 Day 21: 31457.28 62914.53 Day 22: 62914.56 125829.09 Day 23: 125829.12 251658.21 Day 24: 251658.24 503316.45 Day 25: 503316.48 1006632.93 Day 26: 1006632.96 2013265.89 Day 27: 2013265.92 4026531.81 For Job 3, you earned $4026531.81 in 27 day(s). Job 3 was the highest paying job
Step by Step Solution
There are 3 Steps involved in it
Step: 1
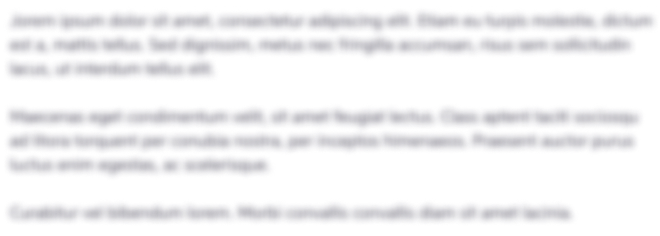
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started